Kodeclik Blog
How to take input until enter is pressed in Python
In your Python journey you will encounter a need to write interactive Python programs, i.e., programs that stop to take input from the user and then do some processing based on the user’s input. In this blogpost we will see how to write such interactive Python programs.
There are two scenarios of user input-driven execution and we will cover both of them here.
Let us suppose we desire to write a program that greets the user, asks for their name, and prints a response in return. Here is how such a program will look:
greeting = "Hi, how are you? What is your name? "
name = input(greeting)
print("Welcome " + name + "! Nice to meet you.")
In the first line, we initialize a string containing the greeting. The second line uses the input() function which prints the greeting first and waits for input from the user (till the Enter key is pressed). Once the enter key is pressed the input provided by the user is stored in the variable “name”. In the third line, we use this variable to compose a suitable response. Here’s an example of how the program execution will unfold:
Hi, how are you? What is your name? Tommy
Welcome Tommy! Nice to meet you.
The input() function takes only one argument, namely the prompt. Note that the prompt is given an extra space at the end of its string for ease of readability when the user types his/her response.
If the user types Enter immediately upon seeing the prompt, here’s how that interaction will look like:
Hi, how are you? What is your name?
Welcome ! Nice to meet you.
If we wish to check for these situations, we can conduct some error trapping:
greeting = "Hi, how are you? What is your name? "
valid_input = False
while (not valid_input):
name = input(greeting)
if (name != ''):
print("Welcome " + name + "! Nice to meet you.")
valid_input = True
break
Let us try out this program with a string of Enters followed by a suitable response:
Hi, how are you? What is your name?
Hi, how are you? What is your name?
Hi, how are you? What is your name?
Hi, how are you? What is your name? k
Welcome k! Nice to meet you.
In the first three prompts above, we press Enter and our program traps this condition and continues asking the prompting question. In the fourth prompt, the user enters his/her name as “k” and the program execution terminates after printing the message.
This program can be made more succinct as follows:
greeting = "Hi, how are you? What is your name? "
name = ""
while (not name):
name = input(greeting)
print("Welcome " + name + "! Nice to meet you.")
Here is a sample output, as before:
Hi, how are you? What is your name?
Hi, how are you? What is your name?
Hi, how are you? What is your name?
Hi, how are you? What is your name?
Hi, how are you? What is your name?
Hi, how are you? What is your name?
Hi, how are you? What is your name? Kodeclik
Welcome Kodeclik! Nice to meet you.
You might sometimes need to write a program that accepts multiple lines of input each with different values and a blank Enter signifies that you are done inputting. For instance let us write a program that reads numbers, one line at a time, until you press a blank Enter. Upon receiving a blank Enter, the program must output the sum of the numbers read.
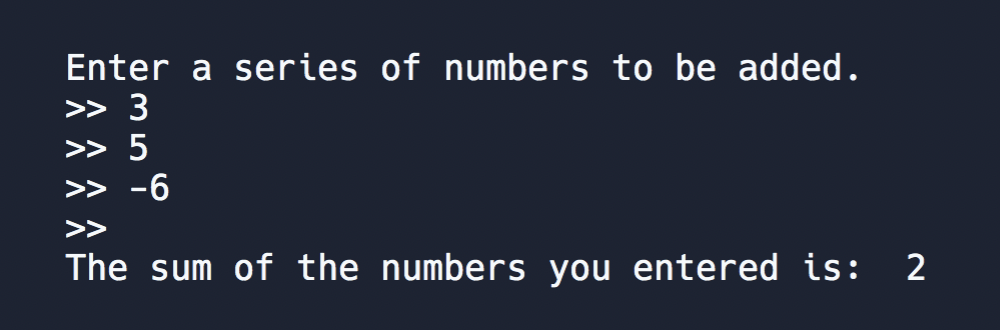
Here is a first try at how such a program can be organized:
print("Enter a series of numbers to be added.")
prompt = ">> "
done = False
while (not done):
num = input(prompt)
Here we print a message, and perform an infinite while loop asking for numbers. Note that because the done variable is never made True, the while loop will continue indefinitely. Here is a sample run:
Enter a series of numbers to be added.
>> 3
>> 3
>> 4
>> 5
Now let us modify the program to check for an Enter key and if so reset the done variable so we can exit the loop:
print("Enter a series of numbers to be added.")
prompt = ">> "
done = False
while (not done):
num = input(prompt)
if (num == ""):
done = True
Here is a sample output:
Enter a series of numbers to be added.
>> 1
>> 2
>> 3
>> 4
>> 5
>> 6
>>
When we press Enter for the last prompt, the program exits.
Now finally, we can add the logic to sum the series of numbers:
print("Enter a series of numbers to be added.")
prompt = ">> "
done = False
sum = 0
while (not done):
num = input(prompt)
if (num == ""):
done = True
else:
sum = sum + int(num)
print("The sum of the numbers you entered is: ", sum)
Here is some sample output:
Enter a series of numbers to be added.
>> 1
>> 2
>> 3
>>
The sum of the numbers you entered is: 6
Note that we accumulate the sum in the “sum” variable. Also observe that we use the int() casting operator to convert the user’s input (which will be a string) into a form that can be added.
In this blogpost, we have seen code that can take inputs in Python till the Enter key is pressed. We covered both single line input cases as well as multi-line input cases.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.