Kodeclik Blog
How to initialize a Queue in Python
A queue is a first-in-first-out (FIFO) data structure. For instance, consider a queue of passengers boarding the plane. The person who enters the queue last will be the last person to board the plane. Likewise, the person who was first will be the earliest to board the plane.
Queues can be implemented in Python in many ways. Below we highlight each of these methods.
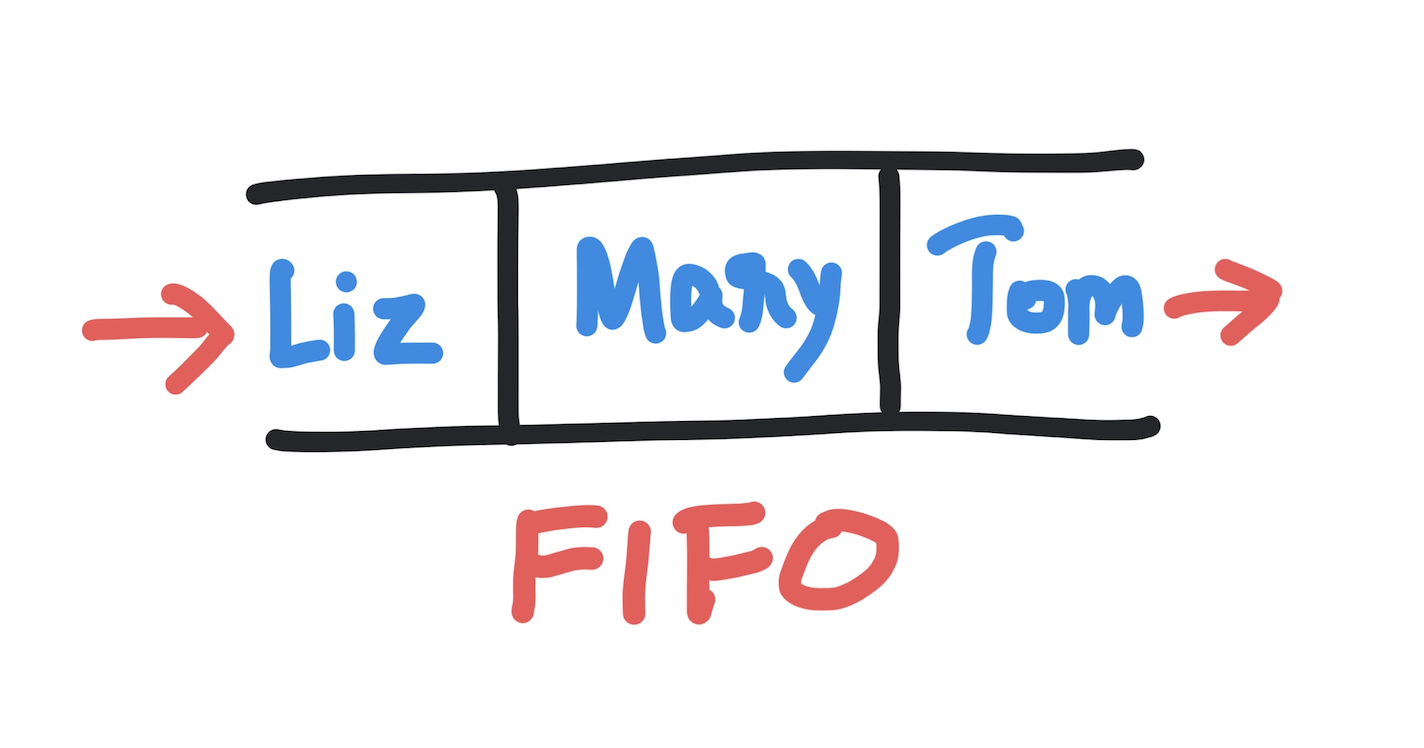
Method 1: Use a list data structure
Lists come by default in Python and we can use a list to mimic queues. Assume you have a queue of passengers: “Tom”, “Mary”, and “Liz”. Below is code to initialize the queue (as an empty list), adding passengers to the list using the “append” method and finally printing the passengers.
passengers = []
passengers.append('Tom')
passengers.append('Mary')
passengers.append('Liz')
print(passengers)
The output is:
['Tom', 'Mary', 'Liz']
As you can see the item inserted earliest appears first in the list and the item inserted last appears last.
Of course you can also initialize the queue directly with three passengers rather than one at a time. The code below accomplishes this:
passengers = ['Tom','Mary','Liz']
print(passengers)
with the same output as before.
Method 2: Use a collections.deque data structure
The second approach to initialize and implement a queue in Python is to use the collections module and specifically the data structure called “deque” (double ended queue). A deque is more than what we need but it is optimized for fast insertions and removals. Here is the code to initialize a queue using the collections.deque data structure and add elements one by one.
from collections import deque
passengers = deque([])
passengers.append('Tom')
passengers.append('Mary')
passengers.append('Liz')
print(passengers)
The passengers data structure is initially set to be an empty deque. Then three elements are added one by one so that when we print it we get the output:
deque(['Tom', 'Mary', 'Liz'])
Again, like the Python list data structure you can initialize it with the three elements directly:
from collections import deque
passengers = deque(['Tom','Mary','Liz'])
print(passengers)
giving the same output as before.
Method 3: Use a queue.Queue data structure
The third approach to initialize a queue is to use the queue module and the Queue data structure within it. Here is the corresponding code and methods to accomplish the same example as above:
from queue import Queue
passengers = Queue(maxsize=5)
passengers.put('Tom')
passengers.put('Mary')
passengers.put('Liz')
print(passengers)
In the above program, the parameter 5 refers to the maximum size of the queue. Each put() command adds an element. The output is (your specific output might vary):
<queue.Queue object at 0x7fc510228580>
This output is not very informative. To confirm the contents of the queue have been added as intended, we can use the get() method:
print(passengers.get())
print(passengers.get())
print(passengers.get())
The output will be:
Tom
Mary
Liz
The get() method removes elements from the queue in the order they are added. Following these get() methods, the queue will be empty.
There you have it - three different methods to initialize a queue. Which method is your favorite?
Interested in more things Python? Checkout our post on Python stacks. See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.