Kodeclik Blog
Computing powers in Javascript
Javascript is used by almost every website to do calculations on the browser and support client-side interaction as well as server-side computations. Here we will see how to write simple Javascript programs to compute powers of numbers.
We will embed our Javascript code inside a HTML page like so:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Javascript power demo</title>
</head>
<body>
<script>
</script>
</body>
</html>
In the above HTML page you see the basic elements of the page like the head element containing the title (“Javascript power demo”) and a body with an empty script tag. The Javascript code goes inside these tags.
There are two ways to compute powers in Javascript. Below is each approach described in detail.
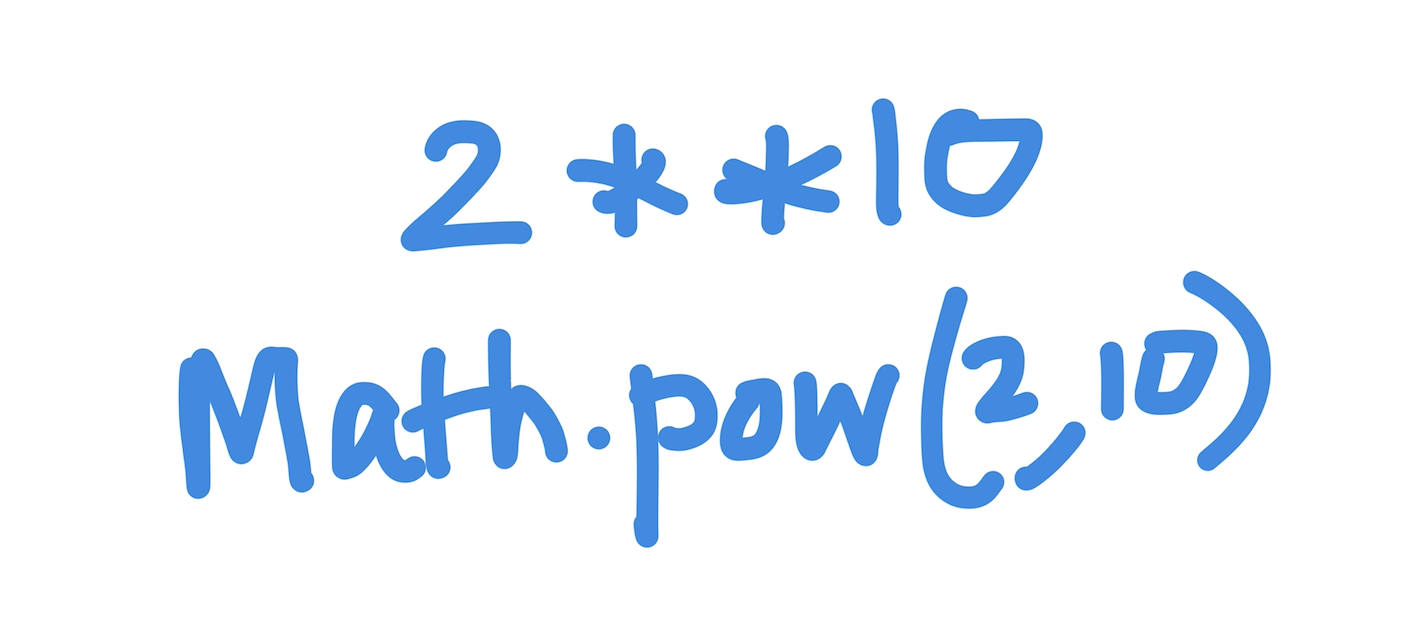
Method 1: Use the exponentiation operator (**)
The first way to compute powers is to use the exponentiation operator (**). Here is some basic code for the same (only the script parts of the page are shown):
<script>
document.write("2^10 is: " + 2**10);
</script>
When this page is rendered, it will look like:
2^10 is: 1024
You can also use the “**=” exponentiation assignment operator like so:
<script>
x = 2;
x **= 10;
document.write("2^10 is: " + x);
</script>
This will also output:
2^10 is: 1024
Method 2: Use the Math.pow() function
A second method is to use the Math.pow() function. Here is how that looks (with a newline printed in HTML):
<script>
document.write("2^10 is: " + 2**10);
document.write("<BR>");
document.write("Another way to do 2^10 is: " + Math.pow(2,10));
</script>
The output is:
2^10 is: 1024
Another way to do 2^10 is: 1024
In the Math.pow() function, the first argument is the base (2, in our case) and the second argument is the exponent (10, in our case).
Math.pow() when base or exponent is zero
Note that if the base is 0, the result of the exponentiation will be 0. Similarly, if the exponent is 0, the result of the exponentiation will be 1. Here is a simple program to try this:
<script>
document.write("2^0 is: " + Math.pow(2,0));
document.write('<BR>')
document.write("0^2 is: " + Math.pow(0,2));
</script>
The output is:
2^0 is: 1
0^2 is: 0
Math.pow() with negative (integer) arguments
You can also use the Math.pow() function with negative (Integer) arguments:
<script>
document.write("2^-1 is: " + Math.pow(2,-1));
document.write('<BR>')
document.write("-1^2 is: " + Math.pow(-1,2));
</script>
The output is:
2^-1 is: 0.5
-1^2 is: 1
Math.pow() with zero raised to negative exponents
If we try a program where zero is raised to negative exponents:
<script>
document.write("0^-3 is: " + Math.pow(0,-3));
</script>
Javascript outputs:
0^-3 is: Infinity
Math.pow() with floating point inputs
Let us try Math.pow() with floating point inputs for either the base or the exponent (or both):
<script>
document.write("0.5^3.5 is: " + Math.pow(0.5,3.5));
document.write('<BR>')
document.write("-0.5^3.5 is: " + Math.pow(-0.5,3.5));
document.write('<BR>')
document.write("0.5^-3.5 is: " + Math.pow(0.5,-3.5));
document.write('<BR>')
document.write("-0.5^-3.5 is: " + Math.pow(-0.5,-3.5));
</script>
The output will be:
0.5^3.5 is: 0.08838834764831843
-0.5^3.5 is: NaN
0.5^-3.5 is: 11.31370849898476
-0.5^-3.5 is: NaN
Note that we get a NaN (Not a Number) output whenever the base is negative and the exponent is a floating point number.
Try the same input combinations above with the exponentiation operator and see if you get the same outputs!
Enjoy computing powers in Javascript!
Would you like to learn more Javascript? Checkout our blog post on checking if numbers are within a range in Javascript!
Want to learn Python with us? Sign up for 1:1 or small group classes.