Kodeclik Blog
How to subtract dates in Javascript
To learn how to subtract dates in Javascript, lets first understand the Date constructor in Javascript. This is a handy way to create Date objects.
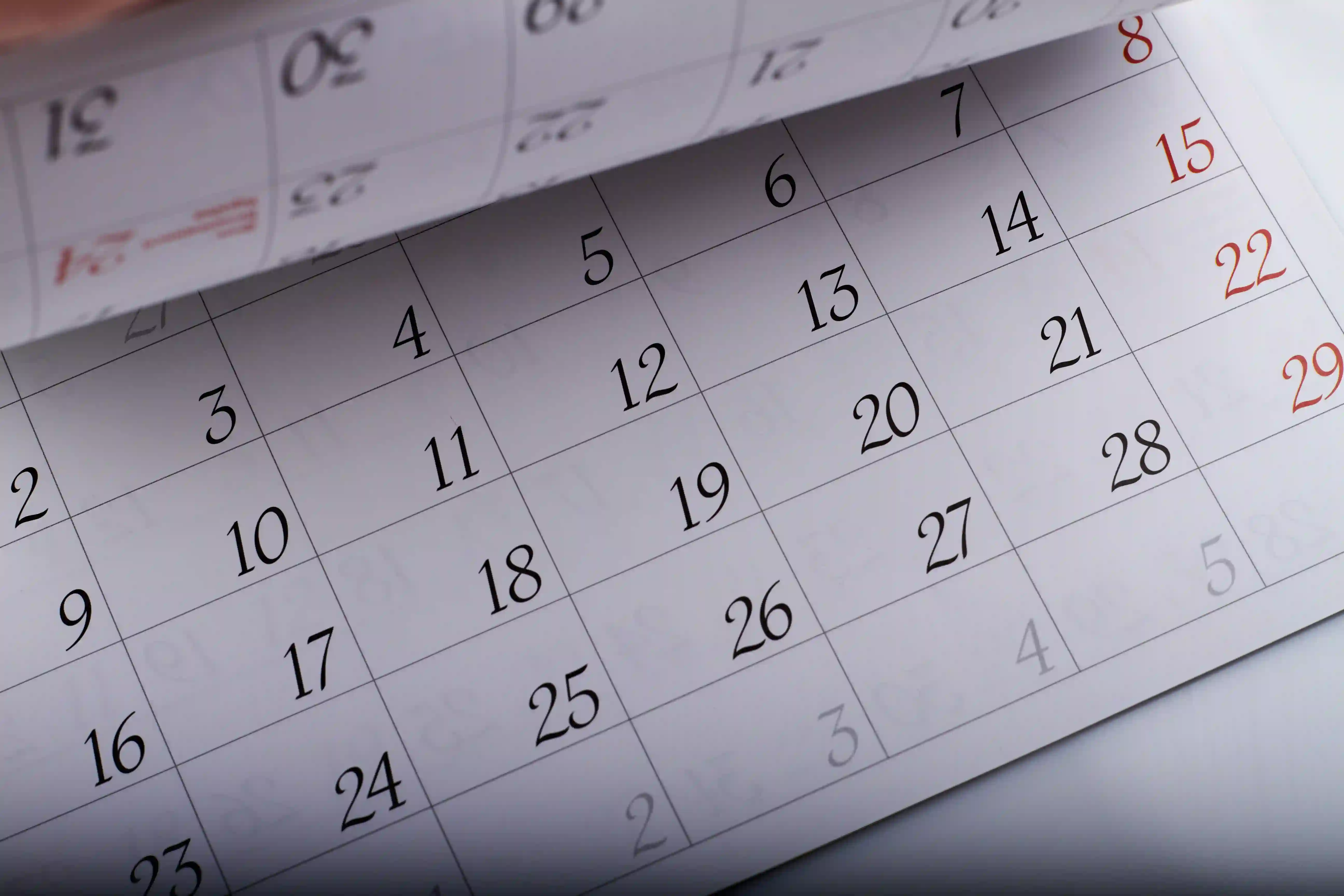
Here is a simple Javascript program to explore Date objects:
// Current date and time
const today = new Date();
document.write(today)
document.write('<BR><BR>')
// Date from a string
const specificDate = new Date("2023-12-31");
document.write(specificDate)
document.write('<BR><BR>')
// Date with specific components
const customDate = new Date(2023, 11, 31);
document.write(customDate)
document.write('<BR><BR>')
// Make a copy of an existing Date object
const dateCopy = new Date(customDate);
document.write(dateCopy)
document.write('<BR><BR>')
Note that this program shows different ways of using the Date constructor. In the first usage, we call it without arguments and it returns a Date object for the current date and time. In the second instance, we create a Date object using a date string (such as "2023-12-31"). In the third usage, we create a Date object with individual arguments (there are more possible but we show only three here) - the year is 2023, the month index is 11 (but since indices start at 0, this is really denoting Dec), and the third argument is 31 (meaning Day 31). Finally, in the fourth usage of the Date constructor, we are making a copy of an existing Date object by passing the Date object as a parameter, for example: new Date(customDate).
The output of this program is (note that your output will change, at least for the first line, depending on when you run this program):
Fri Dec 29 2023 11:41:45 GMT-0500 (Eastern Standard Time)
Sat Dec 30 2023 19:00:00 GMT-0500 (Eastern Standard Time)
Sun Dec 31 2023 00:00:00 GMT-0500 (Eastern Standard Time)
Sun Dec 31 2023 00:00:00 GMT-0500 (Eastern Standard Time)
The output is quite self-explanatory. The first date is printed in EST (since kodeclik.com is at EST) and it prints Dec 29, 2023 since that is the current date when the program is run.
The second date might be confusing. We specifically gave the input as Dec 31, 2023 but instead it is printed as Dec 30, 2023. Why? The reason is that when we create a date using new Date("2023-12-31"), the date is interpreted as UTC, and when you display it using document.write(specificDate), it's adjusted to your local time, which might result in the date being displayed as a day earlier due to the time zone difference. This behavior is due to the way the Date constructor handles the input date string. To display the date in the desired format, you can use the third approach which explicitly specifies the components. This prints the date as desired, i.e., Dec 31, 2023. Finally, the last Date is simply a copy of the third date and therefore there is no difference in outputs between the third and last dates.
To subtract dates in Javascript, you can use the following constructs:
let startDate = new Date(2023, 0, 01);
let endDate = new Date(2023, 11, 31);
let timeDifference = endDate.getTime() - startDate.getTime();
let dayDifference = (timeDifference / (1000 * 3600 * 24)) + 1;
document.write("Difference between dates is: " + dayDifference);
To understand what this program does, note that the two date objects, startDate and endDate, are created using the Date constructor using the second method above so we don’t run into timezone mishaps. The startDate is set to January 1, 2023, and the endDate is set to December 31, 2023. The program then calculates the time difference in milliseconds between the two dates using the getTime method of the Date object and subtracting the start date from the end date. Next, the time difference is converted to days by dividing it by the number of milliseconds in a day (1000 * 3600 * 24). The addition of 1 is done to include both the start and end dates in the count. Finally, the program uses document.write to display the calculated number of days between the two dates.
Thus, the use of the second type of Date constructor and the plus 1 in computing the “dayDifference” accounts for time zone mishaps and ensures that the number of days is calculated accurately, including the start and end dates.
The output will be:
Difference between dates is: 365
In fact, you can update the above program to:
let startDate = new Date(2023, 0, 1);
let endDate = new Date(2023, 11, 31);
let timeDifference = endDate - startDate;
let dayDifference = Math.floor(timeDifference / (1000 * 3600 * 24)) + 1;
document.write("Difference between dates is: " + dayDifference);
And it will produce the same output as before! In this updated program, the getTime() function is replaced with a direct subtraction of the two dates. The Math.floor function is used to ensure that the day difference is rounded down to the nearest whole number, and 1 is added to include both the start and end dates in the count. This approach provides an alternative way to calculate the number of days between two dates in JavaScript.
So why or when do we need getTime() at all? The reason is that the getTime() function is typically used to retrieve the number of milliseconds that have elapsed since January 1, 1970, 00:00:00 UTC. This value is essential for performing various date and time calculations, such as finding the difference between two dates, measuring time intervals, or setting very specific dates. It provides a consistent and reliable way to work with dates and times in JavaScript, allowing for precise calculations and comparisons. While there are alternative methods for date manipulation, the getTime() function remains a fundamental tool for handling dates and times in JavaScript. For our purposes of subtracting dates we can get away without it but it is handy to have access to the improved precision offered by getTime().
If you like dabbling with dates, checkout our blogpost on obtaining tomorrow’s (or yesterday’s) date in Javascript!
Want to learn Javascript with us? Sign up for 1:1 or small group classes.