Kodeclik Blog
How to download a file from a URL using Javascript
There are many ways to download a file from a Javascript program. We will explore these ways in this blogpost.
Method 1: Use the download attribute in HTML
To download a file you must first know the address (ie the URL) to the file. The file can be an image, or a document (e.g., a PDF), or even a HTML document. For our purposes we will use this URL (“https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf”) which contains a dummy PDF file that simply says “Dummy PDF file”.
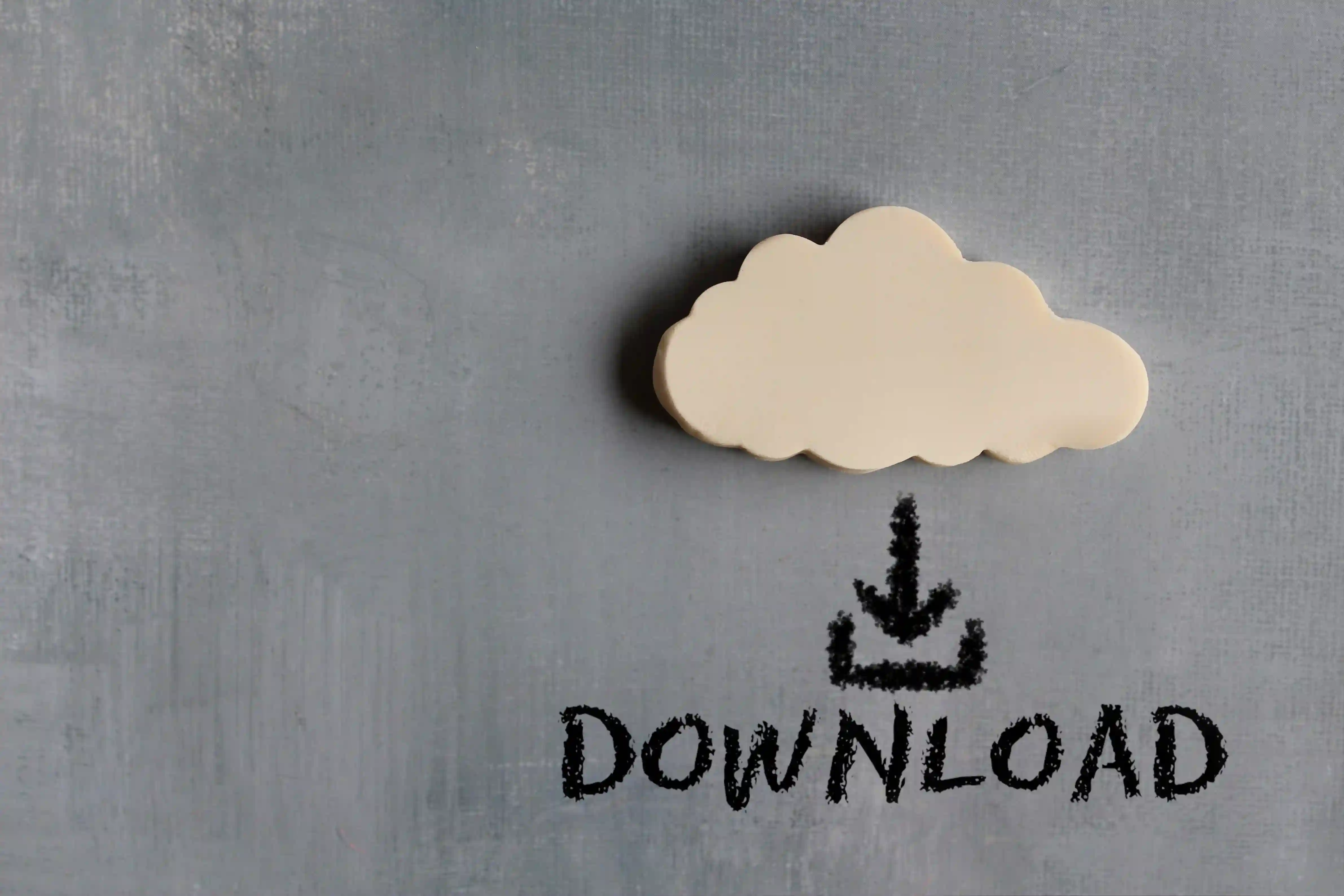
The easiest way to download a file such as the above s to use the download attribute in HTML, which allows the user to download a file directly. We create a HTML page that has the below code in it:
<a href="https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf" download>Download PDF</a>
Clicking on “Download PDF” will thus download the file. It will either download it and save it on your computer, or display it in the browser itself (depending on how your browser is configured to behave to links of type PDF).
Before you go ahead and start exploring this, we should warn you that you should do this only with trusted sources (such as the above URL). Downloading files using Javascript may have security implications and should be used with caution.
Method 2: Create a download link programmatically using Javascript
Consider the following code:
<!DOCTYPE html>
<html>
<head>
<title>Download a file using Javascript</title>
</head>
<body>
<button>Download File</button>
</body>
<script>
document.querySelector('button').onclick = function () {
var link = document.createElement('a');
link.href = 'https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf';
link.download = 'file.pdf';
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
}
</script>
</html>
The provided JavaScript code above works by creating an HTML anchor element, setting its href attribute to the URL of the file you want to download, and then programmatically clicking the anchor element to initiate the download.
In the HTML part of the code, we display a button. In the Javascript portion of the code, a click event listener is added to the button element in the HTML. When the button is clicked, it triggers a function that creates a new anchor element using the document.createElement('a') method. This anchor element is then assigned a href attribute, which is set to the URL of the file to be downloaded. The download attribute of the anchor element is also set to the desired name of the downloaded file ('file.pdf' in this case). This attribute instructs the browser to download the URL instead of navigating to it, and also provides a default filename for the downloaded file.
In the second part of the function, the newly created anchor element is appended to the body of the document using document.body.appendChild(link). This makes the anchor element part of the DOM, although it is not visible to the user. The click() method is then called on the anchor element, which programmatically clicks the element and initiates the download of the file. After the download is initiated, the anchor element is removed from the document body using document.body.removeChild(link). This is done to clean up the DOM and remove the anchor element that was temporarily added.
In summary, this code is similar to Method 1 in that it uses the HTML anchor element and its download attribute to download a file from a given URL when a button is clicked. It does this by creating and clicking an anchor element programmatically, and then removing the element after the download has started.
Method 3: Use the Fetch API
This is a more complicated approach and might not work in all cases. The use of the Fetch API works like this:
fetch('https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf')
.then(response => response.blob())
.then(blob => {
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.href = url;
a.download = 'file.pdf';
document.body.appendChild(a);
a.click();
a.remove();
window.URL.revokeObjectURL(url);
})
.catch(error => console.error(error));
In this example, we first make a GET request to the URL of the file we want to download using the fetch() function. We then extract the file data from the response using the blob() method. We create a URL for the file data using the window.URL.createObjectURL() method and create a new element with the href attribute set to the URL of the file and the download attribute set to the desired filename. We then append the "a" element to the document body, simulate a click on the element using the click() method, and remove the element from the document. Finally, we revoke the URL created earlier using the window.URL.revokeObjectURL() method.
Note that this code snippet assumes that the file being downloaded is a PDF file. If you're downloading a different type of file, you'll need to adjust the download attribute accordingly.
Now, if you try this code you might get an error such as:
Access to fetch at 'https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf'
from origin 'null' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header
is present on the requested resource.
The error message you are seeing indicates that the server hosting the file you are trying to download has not included the necessary CORS headers in its response. This is a security feature implemented by web browsers to prevent cross-site scripting attacks.
When a web page running on one domain tries to access resources on another domain, the browser will check for the presence of the Access-Control-Allow-Origin header in the response. If this header is not present, the browser will block the request.
To resolve this issue, you can either contact the owner of the server hosting the file and ask them to add the necessary CORS headers to their response, or you can use a proxy server to bypass the CORS restrictions. These solutions are beyond the scope of this blogpost; but note that using a proxy server to bypass CORS restrictions can introduce security risks, as it allows your web page to access resources on other domains that may not be intended for public access. Use this approach with caution and only when necessary.
If you liked this blogpost, learn how to get URL parameters using Javascript.
Want to learn Javascript with us? Sign up for 1:1 or small group classes.