Kodeclik Blog
The Increment (++) Arithmetic Operator in Javascript
The increment operator (++) in JavaScript is used to increment (add one to) its operand and returns the value before or after the increment, depending on where the operator is placed.
Consider the following code:
let x = 3;
const y = x++; // x is 4; y is 3
In this example, the postfix increment operator is used, so the value of x is incremented after the assignment to y . This is why y still has the value 3.
On the other hand, if you consider the following code:
let x = 3;
const y = ++x; // x is 4; y is 4
In this example, the prefix increment operator is used, so the value of x is incremented before the assignment to y. So both x and y hold the value 4.
So in summary, if used postfix, i.e., with the operator after the operand (for example, x++), the increment operator increments and returns the value before incrementing. If used prefix, with the operator before the operand (for example, ++x), the increment operator increments and returns the value after incrementing.
Note that the increment operator can only be applied on operands that are references (variables and object properties; i.e. valid assignment targets). The operator is overloaded for two types of operands: number and BigInt. It first coerces the operand to a numeric value and tests the type of it. It performs BigInt increment if the operand becomes a BigInt; otherwise, it performs a standard number increment operation.
The increment operator can only be used on references, that is, the operator can only be applied to variables and object properties. In other words, you cannot use the increment operator (++) multiple times in a row on a single variable. This is because the increment operator, when applied, returns a value and not a reference to a variable.
In Javascript, thus chaining increment operators like ++(++x) would result in a SyntaxError. This is because the inner ++x operation increments x and evaluates to a value, not a reference. The outer ++ then tries to increment this value, not a variable or property, which is not allowed in Javascript.
Consider the following code:
let x = 1;
++(++x); // This will throw a SyntaxError
In this example, the inner ++x operation increments x from 1 to 2, and the result of this operation is the value 2. Then, the outer ++ operation tries to increment this value 2, but since 2 is not a reference (i.e., it's not a variable or property), Javascript throws a SyntaxError.
You can however, do the following, i.e., applying the increment operator separately for each increment:
let x = 1;
++x;
++x; // x is now 3
In this corrected example, the variable x is incremented twice, once for each line where the increment operator is applied. After these two operations, x is 3. The below is a summary of all the above programs:
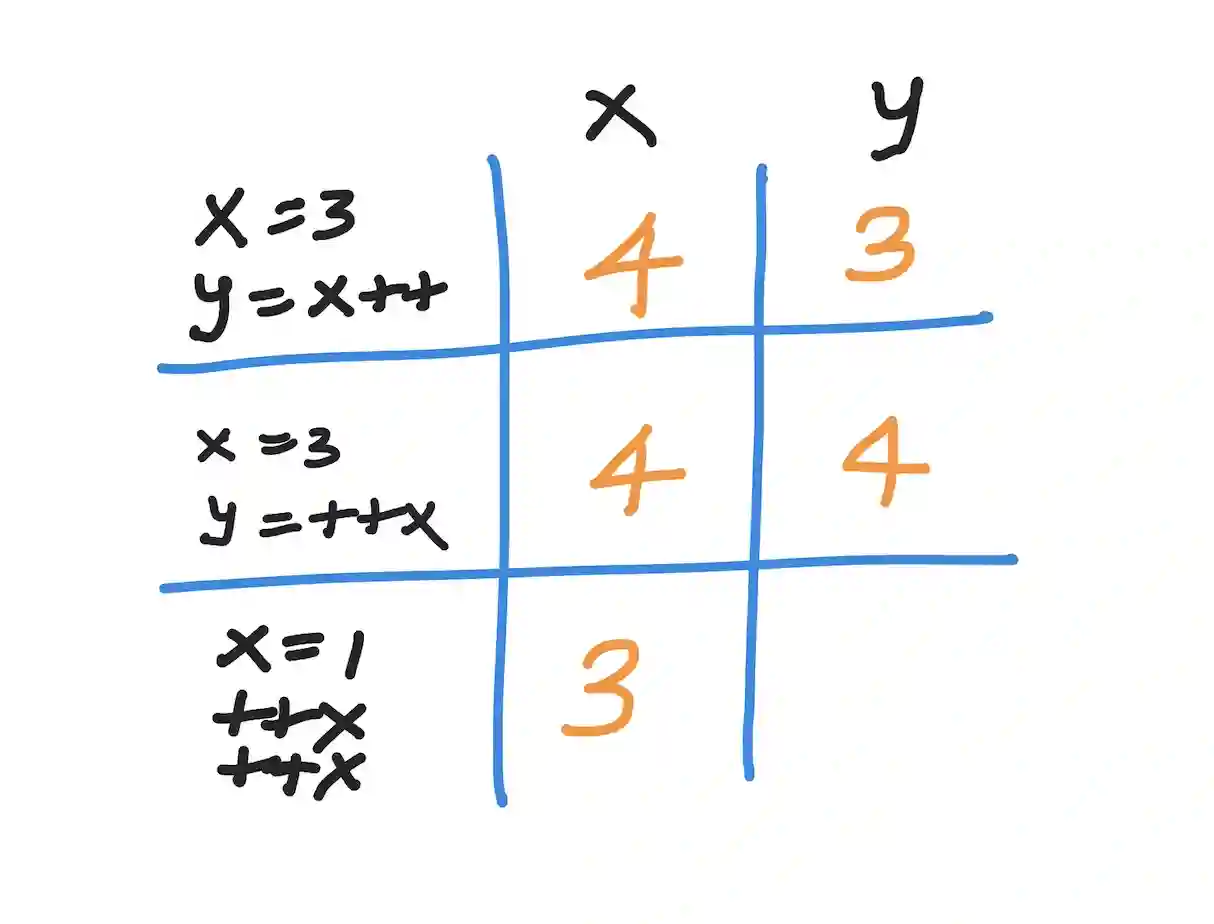
If you liked this blogpost, checkout our blogpost on creating a simple clicker game using incrementing operators.
Want to learn Javascript with us? Sign up for 1:1 or small group classes.