Kodeclik Blog
Boolean Operators in Python
Boolean operators in Python are among the most basic operators. Just like “+” is an arithmetic operator that takes numerical expressions and returns a numerical value, a boolean operator is one that takes boolean expressions (those that resolve to True or False) and returns another boolean expression.
The and Operator
Let us take the most basic boolean operator, the logical AND. AND returns True if and only if both of its operands are True. Here’s a simple Python program that prints the truth table for the boolean AND operator.
print('x\\t\\ty\\t\\tx and y')
print('------------------------')
for i in [False, True]:
for j in [False, True]:
print(i,'\\t',j,'\\t',i and j)
print('------------------------')
The output of this program is:
x y x and y
------------------------
False False False
False True False
True False False
True True True
------------------------
As you can see there are four combinations of truth values and the AND returns True only if both x and y are set to True.
You use the and operator, for instance, in an if conditional (or anywhere a conditional is used, e.g., a while loop).
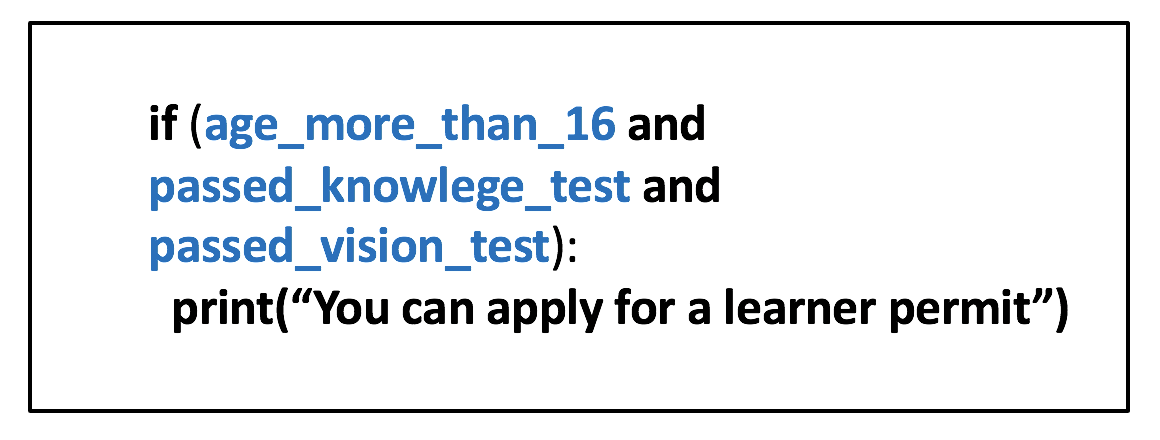
age_more_than_16 = True
passed_knowledge_test = True
passed_vision_test = True
if (age_more_than_16 and passed_knowledge_test and passed_vision_test):
print("You can apply for a learner permit")
if (not age_more_than_16):
print("Please wait till you cross 16 years of age.")
if (not passed_knowledge_test):
print("Please pass the knowledge test.")
if (not passed_vision_test):
print("Please pass the vision test.")
The above program encapsulates the logic that, to obtain a learner permit to drive a car, you need to satisfy three conditions. First, you must be of more than 16 years of age. Second, you must pass a knowledge test. Third, you must pass a vision test. Only if you have all three conditions set to True, you are eligible to apply for a learner permit.
If you run this program, you will get:
You can apply for a learner permit
If you update the first three lines to have False for even one of them the first “if” condition would not trigger and you will not be able to apply for a learner permit. The remaining lines will give you feedback on exactly what you are missing. For instance, if you set False, False, False as the values for the variables and run the program, you will get:
Please wait till you cross 16 years of age.
Please pass the knowledge test.
Please pass the vision test.
indicating that you need to satisfy three conditions before you are ready to apply for a learner permit.
The or Operator
The or operator returns True if any of its arguments are True. It is thus less strict than the and operator. Let us print out the truth table for the or operator:
print('x\\t\\ty\\t\\tx or y')
print('------------------------')
for i in [False, True]:
for j in [False, True]:
print(i,'\\t',j,'\\t',i or j)
print('------------------------')
The output is:
x y x or y
------------------------
False False False
False True True
True False True
True True True
------------------------
As you can see three rows have a True unlike the truth table for the and operator, which had True in only one row.
Notice that the or operator is less strict than the and operator. You use it for instance when you have multiple ways of satisfying a set of conditions. Consider the following program:
raining = False
windy = False
if (raining or windy):
print("I cannot play Tennis now.")
else:
print("I can play Tennis!")
This program will print:
I can play Tennis!
If you change any one of the two boolean variables to True, you will get:
I cannot play Tennis now.
As indicated by the above truth table, note that there are 3 sets of values for which you cannot play Tennis and only one set of values where you can play Tennis (namely when both conditions are False).
This means that the same program can be written as:
raining = False
windy = False
if (not raining and not windy):
print("I can play Tennis!")
else:
print("I cannot play Tennis now.")
Note that this will give you the same result as before. The only difference is that now you are using the and operator instead of the or operator, This is called “De Morgan’s Law”.
The not Operator
Unlike the and and or operators which are binary operators (i.e., they take two arguments), not is a unary operator (it takes only one argument). It is used to negate the value of its argument. In other words, not applied to True gives False. Similarly, not applied to False gives True.
Here’s a program to print the truth table for not:
print('x\\t\\tnot x')
print('------------------------')
for i in [False, True]:
print(i,'\\t',not i)
print('------------------------')
The output is:
x not x
------------------------
False True
True False
------------------------
Here’s a simple program to use the not operator:
raining = True
if (not raining):
do_I_carry_umbrella = False
else:
do_I_carry_umbrella = True
print(do_I_carry_umbrella)
The output, as expected, will be:
True
Hope you enjoyed learning about Python’s boolean operators! To learn more, checkout our blogpost on Python XOR.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.