Kodeclik Blog
How do you measure file size in Python?
You will sometimes encounter the need to measure the size of files from a Python program. Here are 5 ways to do so!
Method 1: Use os.path.getsize()
In Python, there is a module called “os” (for operating system) that is used for a lot of interfacing functionality with the underlying operating system. Within “os”, “os.path” supports various pathname based manipulations. Finally, getsize() obtains the file size in bytes. Here is sample code:
import os
print(os.path.getsize('main.py'))
Here “main.py” refers to this program itself. Depending on your Python implementation, you might need to give the full path name rather than just “main.py”. The output of this program is:
44
Here 44 refers to the number of bytes in the program, with one byte storing one character.
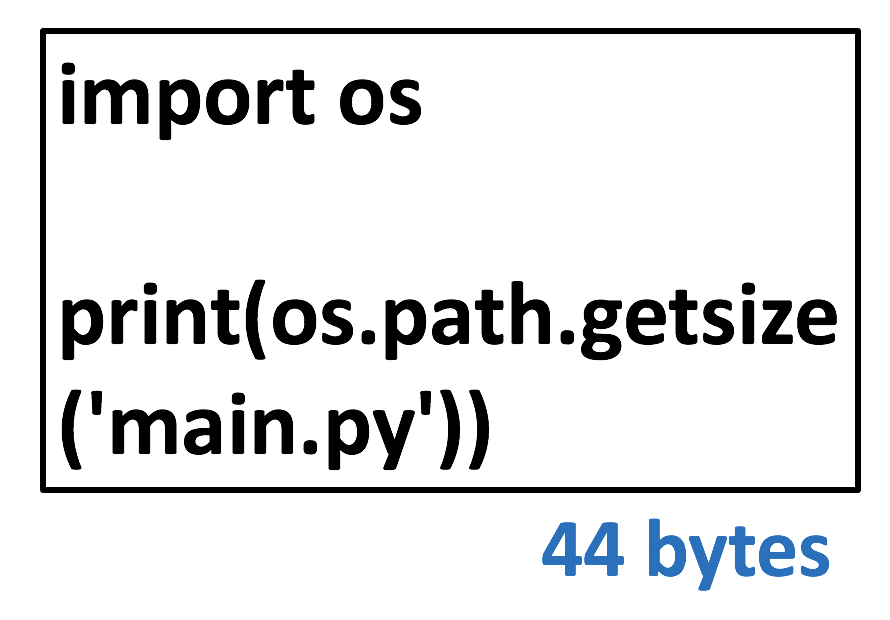
If you specify a filename that doesn’t exist, such as:
import os
print(os.path.getsize('main2.py'))
we will get the output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(os.path.getsize('main2.py'))
FileNotFoundError: [Errno 2] No such file or directory: 'main2.py'
Method 2: Use os.stat(<filaneme>).st_size
This is a second way to find the sizes of files but again using the os module in Python. But note that this uses a different library than “path”. Here we use “stat”.
The corresponding code looks like:
import os
print(os.stat("main.py").st_size)
The output will be the same as before:
44
Method 3: Iterate through the file and count bytes
This is a back-to-basics approach wherein we open the file and begin reading it byte and byte. As we keep reading the file, we increment a counter within our program. Once we reach the end of the program, we print the result. Here is the program:
size = 0
myfile = open("main.py")
while True:
x = myfile.read(1)
if not x:
break
else:
size = size+1
print(x,size)
print(size)
Note that the program does not use any libraries (hence the “back to basics” approach). We use a while loop to read one byte at a time (the read(1) statement). If that byte read does not exist we exit (break) out of the while loop. Else, we increment our counter.
This program will print (at the end, in-between prints not shown for brevity):
146
Note that the size of the program (which is now 146 bytes) has changed because it is a different program.
Method 4: Use pathlib.Path(<filename>).stat().st_size
This method is similar to method 2 above but uses a different module (namely, pathlib instead of os).
import pathlib
print (pathlib.Path('main.py').stat().st_size)
The output will be:
63
Again, note that the file size has changed because the program has changed.
Method 5: Use seek and tell to find filesize
This method is similar to method 3 but not as first principles as that approach. We use seek to first position the file pointer. Such positioning can be done either w.r.t. The beginning of the file or the end of the file. Once the positioning is done we can use tell to count the bytes read thus far and thus the filesize. Here is a program that encapsulates this logic:
import os
fp = open('main.py')
fp.seek(0, os.SEEK_END)
print(fp.tell())
This outputs:
72
(again note that the file has changed and thus the file size is different.)
There you have it - fie different ways to get the file size in your Python program! Which one is your favorite?
If you liked this blogpost, you will also find useful our blogpost on reading a Python file. For more Python system interfacing content, checkout our blogpost on measuring elapsed time in a Python program.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.