Kodeclik Blog
Tuple Index Out of Range!
Do you get the dreaded “Tuple Index Out of Range” error in your Python program? In this blogpost, we are going to learn what it means and how to fix it!
Let us construct a simple tuple comprising days of the week.
days_of_the_week = ('Mon','Tue','Wed','Thu','Fri','Sat','Sun')
Now let us try to print each element of the tuple using a for loop:
for i in range(1, 7):
print(days_of_the_week[i])
This produces the output:
Tue
Wed
Thu
Fri
Sat
Sun
Wow, note that it has printed only 6 days, not 7 days. Let us update our range operator:
for i in range(1, 8):
print(days_of_the_week[i])
This yields the output:
Tue
Wed
Thu
Fri
Sat
Sun
Traceback (most recent call last):
File "main.py", line 4, in <module>
print(days_of_the_week[i])
IndexError: tuple index out of range
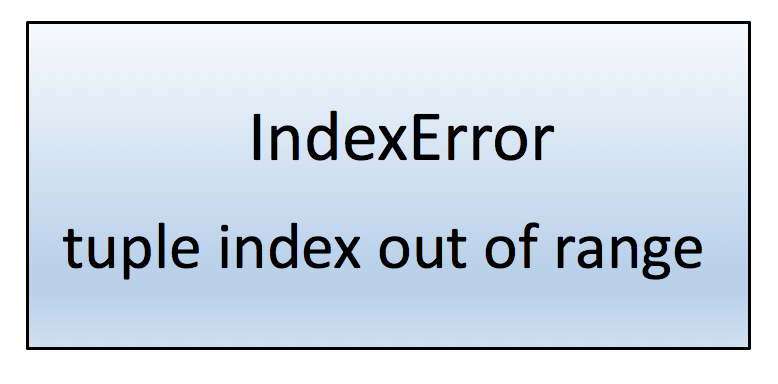
This time the program has printed the same output as before and then gives the dreaded “tuple index out of range” error. This should give you a clue that the upper bound of the index (i.e., 8) is out of range.
So what has happened? This error happens because the index you provided (ie 8) is out of range. So you should not exceed 7. So how can we print “Mon”?
Remember that when you use the range operator in a for loop the second index is not inclusive. So when you specify the range operator as range(1,7) the index takes values from 1 to 6. The first element of the tuple always begins at 0 (and in this case, the last element of the tuple has its index as 6). Let us update the program to reflect this:
for i in range(0, 7):
print(days_of_the_week[i])
Now we obtain the desired output:
Mon
Tue
Wed
Thu
Fri
Sat
Sun
If you reduce the left index of the range operator further, e.g. to -1, you might think you should get an “tuple index out of range” error again. Let us try that:
for i in range(-1, 7):
print(days_of_the_week[i])
The output is:
Sun
Mon
Tue
Wed
Thu
Fri
Sat
Sun
Wow - no error! How can this be? Also note that “Sun” is the first as well as last item to be printed. This is because “-1” is also a way to describe the last element. To simulate the tuple index error on the left side of the data structure, let us try a number like so:
for i in range(-12, 7):
print(days_of_the_week[i])
Now you will get an error:
Traceback (most recent call last):
File "main.py", line 4, in <module>
print(days_of_the_week[i])
IndexError: tuple index out of range
The fact that there are no days printed before this error should again give you a clue that the left index is the one out of range and you can take steps to fix it.
Thus, when you get this error, there are two things you should investigate. 1. Check the lower end of the index. 2. Check the upper end of the index. And recall that indices usually run from zero to one less than the size of your tuple.
Have fun debugging!
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.