Kodeclik Blog
Python’s min function
The min() function in Python is one of the easiest to understand. It is also very versatile. It takes any type of iterable (e.g., a list) as input and returns the minimum value in that iterable.
Let us first try using this function with a list as input.
Python min with list iterable
Consider for instance:
numbers = [4,9,1,-1,25]
print (min(numbers))
This outputs, as expected:
-1
Let us try it with a list of strings:
states = ["Virginia","California","Alabama"]
print (min(states))
The output is:
Alabama
The reason Alabama is printed is because by default, min on a list of strings returns the string that has the lexicographically smallest value. In other words, sort the strings by the lexicographic order and pick the string that is at the beginning. Let us try:
mylist = ["America", "Russia", "China"]
print (min(mylist))
This gives:
America
Python min with string
Because min takes an iterable as input, a string is a valid input. Let us try:
mylist = "Kodeclik"
print (min(mylist))
We get:
K
This is because the individual characters of the string, when sorted, are organized into “K”, “c:, “d”, and so on with “K” being the lexicographically smallest character (upper case characters come earlier than lower case characters). To confirm, let us try a different string:
mylist = "KodeClik"
print (min(mylist))
We get:
C
as expected.
Python min with list iterable and custom sorting function
You are not restricted to using Python defaults for sorting. We can specify a different comparison operator to use with the min() function. For instance, in the earlier example of sorting the elements of a list, we can use length of the element as the criterion to sort the elements:
mylist = ["Humpty", "Dumpty", "sat", "on", "a", "wall"]
print (min(mylist,key=len))
The output is:
a
Note that without the key argument, the result of this min() function would be “Dumpty”. Now it is “a” because we are seeking the (string) element that has the smallest length.
Python max with individual objects
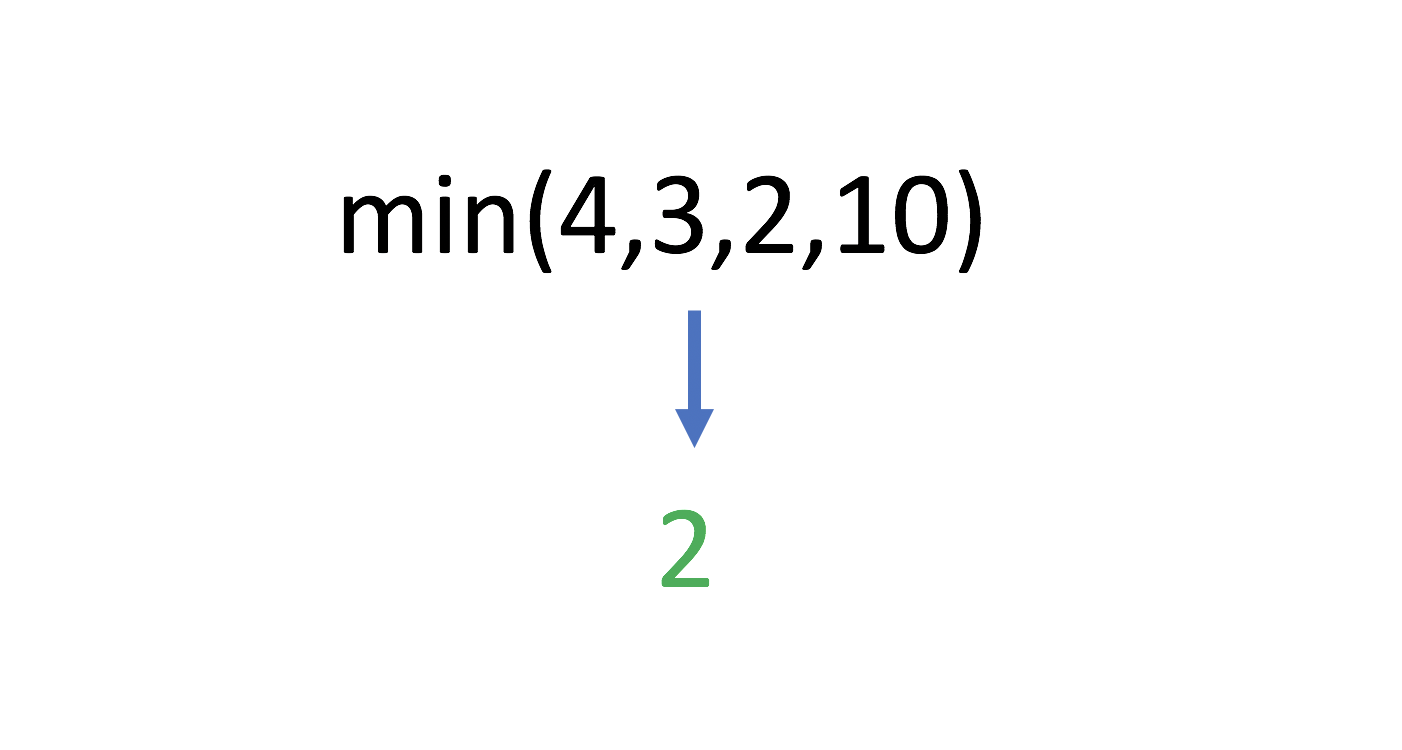
We can also pass to min, not an iterable, but individual objects. For instance:
print (min(4,3,2,10))
This gives as output:
2
We can mix integers and floats in the input:
print (min(4,3,2,-31.3,10))
This gives as output:
-31.3
On the other hand, if you try:
print (min(2,31.3,4,10,"hello"))
we get:
Traceback (most recent call last):
File "main.py", line 1, in <module>
print (min(2,31.3,4,10,"hello"))
TypeError: '<' not supported between instances of 'str' and 'int'
This is because the comparison operator does not know to compare an integer against a string. (But it works when you mix integers and floats).
Just as we customized how elements are sorted, we can do the same when passing individual objects:
print (min("Humpty","hello",key=len))
print (min("Humpty","hello"))
This outputs:
hello
Humpty
In the first line, we are arranging the elements by length and thus “hello” is returned because it has the fewer number of characters. In the second line, there is no “key” variable specified and as a result the default is lexicographic ordering and thus the min value returned is “Humpty”.
Python min with a dictionary
Let us create a dictionary and apply the max() function on it:
months = {1: "January",
2: "February",
3: "March",
4: "April",
5: "May",
6: "June",
7: "July",
8: "August",
9: "September",
10: "October",
11: "November",
12: "December"}
print(min(months))
This outputs:
1
Thus we can see that by default, min() applied to a dictionary uses the key values to sort. If we wish to sort not by the keys but by the associated values, we can define a key parameter and pass on a function to it. Let us suppose we want to sort by the value of the (month) strings so that the min() function should return “April” since the month starting with “Ap” is the lexicographically smallest. If we try:
print(min(months,key=len))
we will get the error:
Traceback (most recent call last):
File "main.py", line 14, in <module>
print(min(months,key=len))
TypeError: object of type 'int' has no len()
This is because by default the function specified in key is being applied to the dictionary’s keys, not to the dictionary’s values. We can fix this problem by defining a lambda function:
print(min(months, key= lambda k: months[k]))
This gives, as expected:
4
because the month with key=4 has the value “April”. To find the actual month, we can do:
monthkey = min(months, key= lambda k: months[k])
print(months[monthkey])
This will output:
April
Instead of sorting the dictionary’s values lexicographically, we can try to sort it by the length of the string.
monthkey = min(months, key= lambda k: len(months[k]))
print(months[monthkey])
This outputs:
May
because May has the fewest characters (3) in a month’s name.
As you have learnt in this blogpost, the min() function in Python is a very versatile function, applicable both to individual values as well as to values in an iterable. Furthermore, you can customize the default behavior of the min() function by providing your own way to rank order the input values.
If you liked the Python min() function, checkout our blogpost on the Python max() function.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.