Kodeclik Blog
Printing to stderr in Python
Consider a very simple Python program.
print("Hello from Kodeclik!")
This outputs, as expected:
Hello from Kodeclik!
When you issue a print statement such as above, you typically also have to describe where exactly you want the printing to happen. By default, this happens in the standard output (or stdout) channel which is usually your monitor or screen. To be explicit and understand that this is what is happening, you can specify:
import sys
print("Hello from Kodeclik!", file=sys.stdout)
This program will give the same output as before.
How to print to stderr
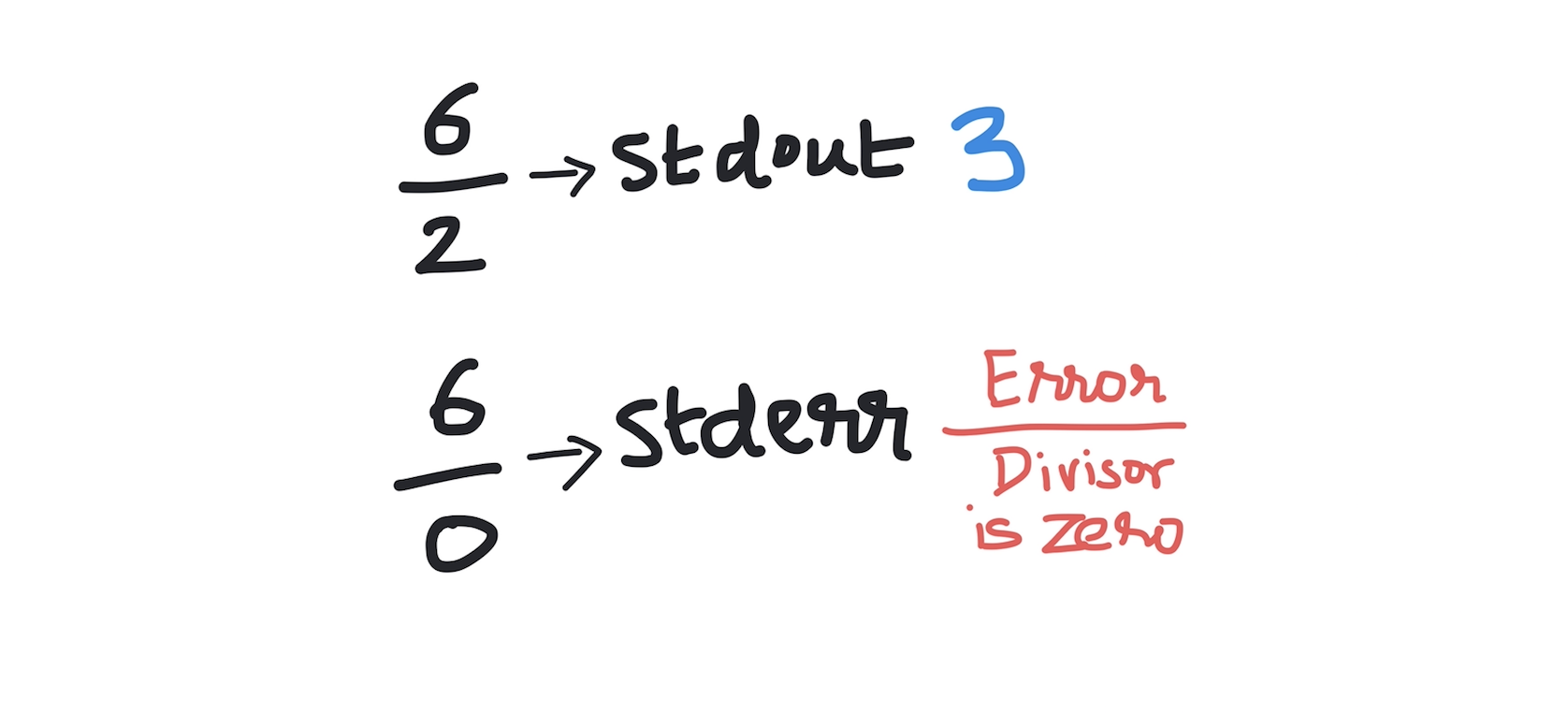
Let us suppose you are writing a division program to take two numbers as input and output a message. Successful program execution results are typically displayed on stdout whereas error messages are displayed on stderr.
import sys
num1 = input("Please enter the dividend: ")
num2 = input("Please enter the divisor: ")
if (int(num2) == 0):
print("Err: Divisor is zero", file=sys.stderr)
else:
print(int(num1)/int(num2), file=sys.stdout)
Note how in the above program the two print statements have different destinations. When you use repl.it you can see that these channels are different by the way repl highlights the results. A correct execution leads to:
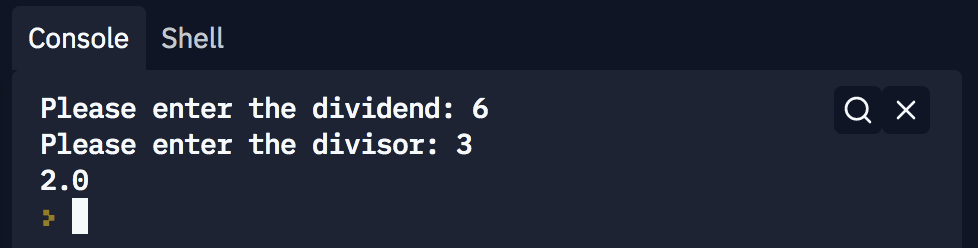
An incorrect execution leads to:
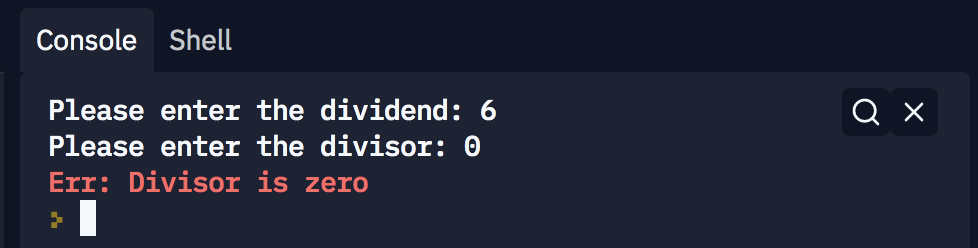
Such messages sent to stderr can be collected in a separate file in a specific location. In fact this is how error messages in various programs, e.g., browsers, are collected so that when something goes wrong we can debug them by inspecting the error log.
Using sys.stderr.write() to print to stderr
An alternative way to print to stderr is to use the write() method provided as part of the sys package. Here is the above program rewritten using this approach:
import sys
num1 = input("Please enter the dividend: ")
num2 = input("Please enter the divisor: ")
if (int(num2) == 0):
sys.stderr.write('Err: Divisor is zero')
else:
print(int(num1)/int(num2), file=sys.stdout)
It produces the same output:
Please enter the dividend: 6
Please enter the divisor: 0
Err: Divisor is zero
Note that this approach, unlike the print command, does not automatically add a newline so an additional write will continue from where it left off. So you will need to update the program as follows:
import sys
num1 = input("Please enter the dividend: ")
num2 = input("Please enter the divisor: ")
if (int(num2) == 0):
sys.stderr.write('Err: Divisor is zero')
sys.stderr.write('\\n')
sys.stderr.write('Please try again.')
else:
print(int(num1)/int(num2), file=sys.stdout)
The output is:
Please enter the dividend: 6
Please enter the divisor: 0
Err: Divisor is zero
Please try again.
What is the difference between print() and write() to stderr?
One key difference between these two approaches is that sys.stderr.write() is limited in that it can only output string messages. Suppose, in addition to outputting the helpful division-by-zero message above we also wish to reproduce the divisor and dividend, we try:
import sys
num1 = input("Please enter the dividend: ")
num2 = input("Please enter the divisor: ")
if (int(num2) == 0):
sys.stderr.write('Err: Divisor is zero')
sys.stderr.write('\\n')
sys.stderr.write([num1,num2])
else:
print(int(num1)/int(num2), file=sys.stdout)
We get:
Please enter the dividend: 6
Please enter the divisor: 0
Err: Divisor is zero
Traceback (most recent call last):
File "main.py", line 9, in <module>
sys.stderr.write([num1,num2])
TypeError: write() argument must be str, not list
Interestingly, the first “Err: Divisor is zero” message is printed by us to stderr and the remaining lines are also printed to stderr (but by the Python interpreter). To be able to print lists, the print command is more versatile:
import sys
num1 = input("Please enter the dividend: ")
num2 = input("Please enter the divisor: ")
if (int(num2) == 0):
sys.stderr.write('Err: Divisor is zero')
sys.stderr.write('\\n')
print([num1,num2],file=sys.stderr)
else:
print(int(num1)/int(num2), file=sys.stdout)
The output will now be:
Please enter the dividend: 6
Please enter the divisor: 0
Err: Divisor is zero
['6', '0']
You have learnt all about the Python stderr channel and how you can use it to output error messages, warnings, and other useful information in the course of your program execution. Next time you interact with a program you can use your understanding to distinguish between messages sent to stdout versus those sent to stderr.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.