Kodeclik Blog
How to merge multiple files into a single file in Python
You will sometimes have a situation where you have two or more files containing data and you desire to merge them into a single file. Here we will learn a Python program do accomplish this task.
Let us suppose we have 3 files named file1.txt, file2.txt, and file3.txt containing:
<file1.txt>
Harry Potter
and the Philosopher's Stone
<file2.txt>
Harry Potter
and the Chamber of Secrets
<file3.txt>
Harry Potter
and the Prisoner of Azkaban
The goal is to merge them into a single file as shown below:
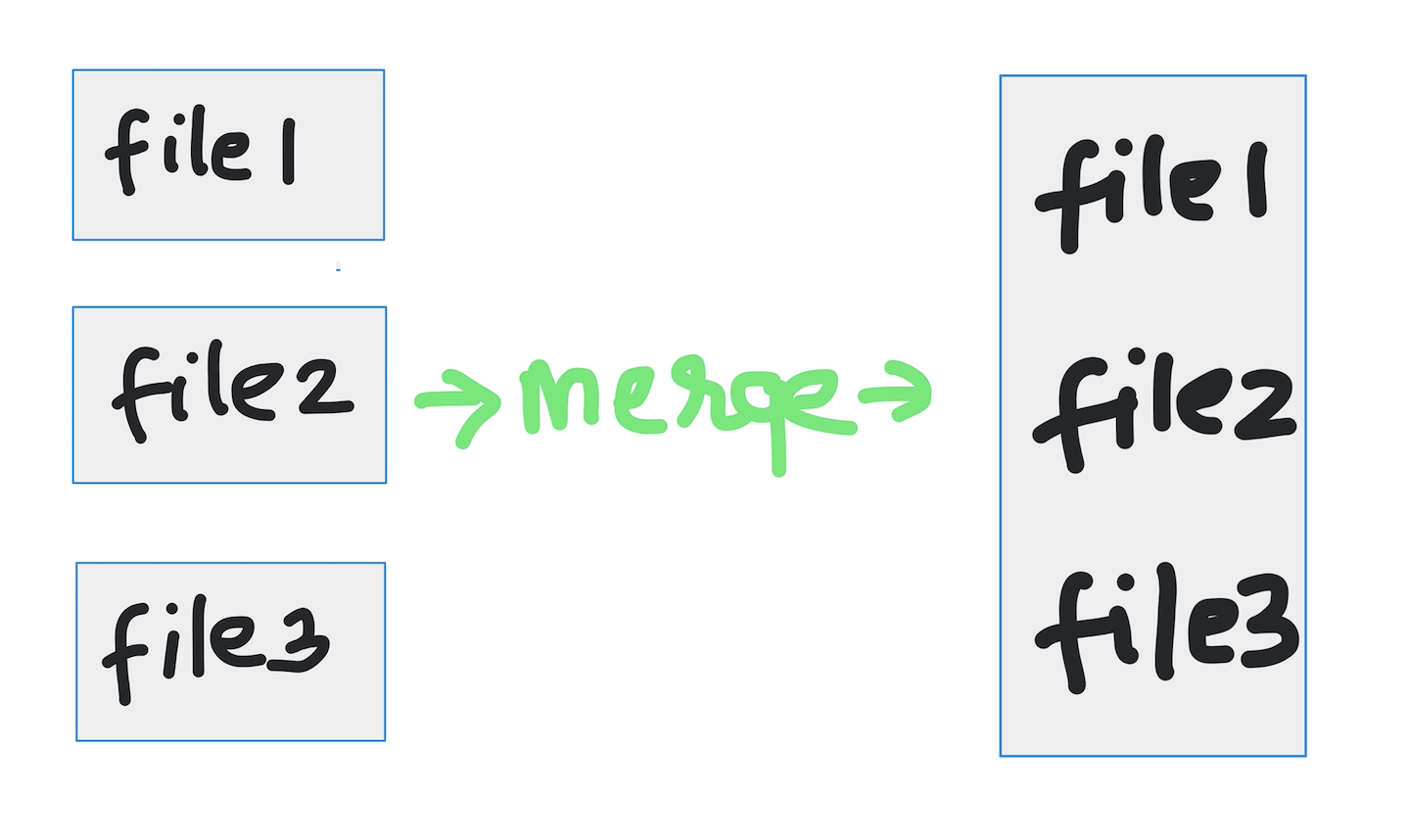
Let us write a Python program to first read these files. We embed the file opening and reading commands inside a for loop to cycle through these multiple files:
myfiles = ['file1.txt','file2.txt','file3.txt']
for f in myfiles:
with open(f) as infile:
contents = infile.read()
print(contents)
Here the loop cycles through the three files in the list called “myfiles”. For each of these files, represented by the variable “f”, we open the file and use “infile” as the file pointer variable. We then use the read() method to read the contents of “infile” and print them. Note that depending on the loop iteration, the variable “infile” refers to different files.
The output will be, as expected:
Harry Potter
and the Philosopher's Stone
Harry Potter
and the Chamber of Secrets
Harry Potter
and the Prisoner of Azkaban
Now we are ready to write these contents into a new file. Let us call it “mergedfile.txt”. The updated program will be:
myfiles = ['file1.txt','file2.txt','file3.txt']
with open('mergedfile.txt', 'w') as newfile:
for f in myfiles:
with open(f) as infile:
contents = infile.read()
newfile.write(contents)
Note that we now embed the existing for loop inside a larger loop that opens “mergedfile.txt” in write mode and then instead of printing the contents to the standard output channel, we write them into the newfile.
If we run this program, you should see a new file called “mergedfile.txt” being created by the program. Let us open it and inspect its contents:
Harry Potter
and the Philosopher's StoneHarry Potter
and the Chamber of SecretsHarry Potter
and the Prisoner of Azkaban
Oops! Looks like we didn’t print a newline after each file’s contents. Here is an updated program:
myfiles = ['file1.txt','file2.txt','file3.txt']
with open('mergedfile.txt', 'w') as newfile:
for f in myfiles:
with open(f) as infile:
contents = infile.read()
newfile.write(contents)
newfile.write('\n')
The new output will be as we expected:
Harry Potter
and the Philosopher's Stone
Harry Potter
and the Chamber of Secrets
Harry Potter
and the Prisoner of Azkaban
You can extend this program to work on more files by simply updating the “myfiles” variable.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.