Kodeclik Blog
Python’s range() function
Python’s range function becomes second nature to Python programmers that we sometimes do not notice that it is a real function with arguments and return values. The range function is used to return a list of values between specified start and stop points and incrementing with specified step sizes.
Python’s range() function with one input
The easiest way to use Python’s range() function is to give it one input value:
r = range(6)
If you run the above code, you will notice that nothing happens. Let us try to print the variable “r”:
r = range(6)
print(r)
The output is now:
range(0, 6)
This is not very descriptive but for our purposes it represents a sequence of numbers. To understand the sequence, we can index into it. For instance if we do:
r = range(6)
print(r[0])
print(r[1])
print(r[2])
we get:
0
1
2
In general the common practice is to use the range function in a for loop:
for x in range(6):
print(x)
The output is:
0
1
2
3
4
5
Note that the start value is zero (by default) and the ending value is one less than the input. Because the input is 6, the final value is 5. You can use the range() operator to perform some suitable operation, eg:
for x in range(6):
print(str(x) + " squared is: " + str(x*x))
The output of the above program will be:
0 squared is: 0
1 squared is: 1
2 squared is: 4
3 squared is: 9
4 squared is: 16
5 squared is: 25
Python’s range() function with two inputs
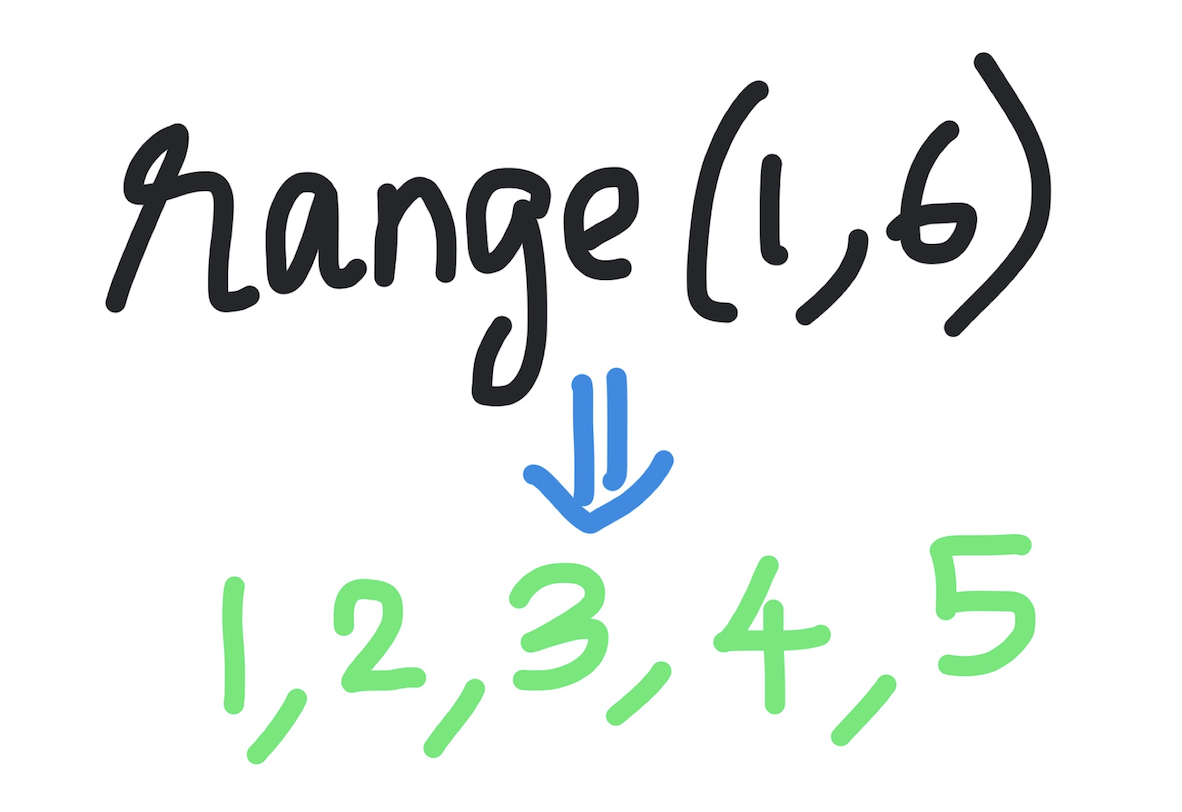
If range() is given two inputs, the first input is taken as the starting value and the second input is one less than the final value (as before). Consider the following code:
for x in range(1,6):
print(str(x) + " squared is: " + str(x*x))
The output is:
1 squared is: 1
2 squared is: 4
3 squared is: 9
4 squared is: 16
5 squared is: 25
Note that the output begins at 1 (because that is the first parameter to range()) and ends at 5 (one less than the second argument).
Python’s range() function with three inputs
If range() is given three inputs, the first two inputs are the same as before (start, and ending-1 values) but the third input is viewed as the step size:
for x in range(1,10,3):
print(str(x) + " squared is: " + str(x*x))
The output will be:
1 squared is: 1
4 squared is: 16
7 squared is: 49
The starting value is 1 because that is the first argument. The step size is 3 so at each step the indicator variable x is incremented by 3, so it becomes 4, then 7. But when the value becomes 10, it exceeds (or equals) the range’s second parameter and therefore the loop stops.
Using range() to count backwards
We can easily adapt the code above to count backwards. Just use a starting value higher than the stop value and a negative value for the step size.
for x in range(20,2,-3):
print(str(x) + " squared is: " + str(x*x))
The output is:
20 squared is: 400
17 squared is: 289
14 squared is: 196
11 squared is: 121
8 squared is: 64
5 squared is: 25
Note that the start argument is inclusive which is why the range begins at 20. At each step the value is decremented by 3. Once it equals or exceeds the stop value, the iteration stops.
Using range() to create ad-hoc sequences
We can use range() as part of a larger strategy to create ad-hoc sequences for use in an iteration. For this purpose we use the itertools module available in Python:
from itertools import chain
for x in chain(range(0,10,3),range(20,30,2)):
print(str(x) + " squared is: " + str(x*x))
In the above code we have two range operators, one from 0 (inclusive) to 10 (not inclusive) in steps of 3, and another from 20 (inclusive) to 30 (not inclusive) in steps of 2. These two sequences are chained using the chain function available in itertools. The output will be:
0 squared is: 0
3 squared is: 9
6 squared is: 36
9 squared is: 81
20 squared is: 400
22 squared is: 484
24 squared is: 576
26 squared is: 676
28 squared is: 784
In this blogpost we have learnt about the very useful range() function, the various ways in which it could be invoked and how it can be used to both count up and count down. We also learnt about step sizes and how to chain different ranges. For a slight variant of the Python range() function, see our blogpost on creating an inclusive range in Python. What other ideas do you have for the range() function?
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.