Kodeclik Blog
Python’s sum() function
Python’s sum function is a very useful function to work with iterables. Recall that iterables are data structures in Python that are capable of returning their elements one by one so that you can use them in a for loop, for instance. Example iterables are lists, dictionaries, sets, and tuples.
Using the Python sum() function
Consider the following simple piece of code:
numbers = [1,3,5,7,9]
print(sum(numbers))
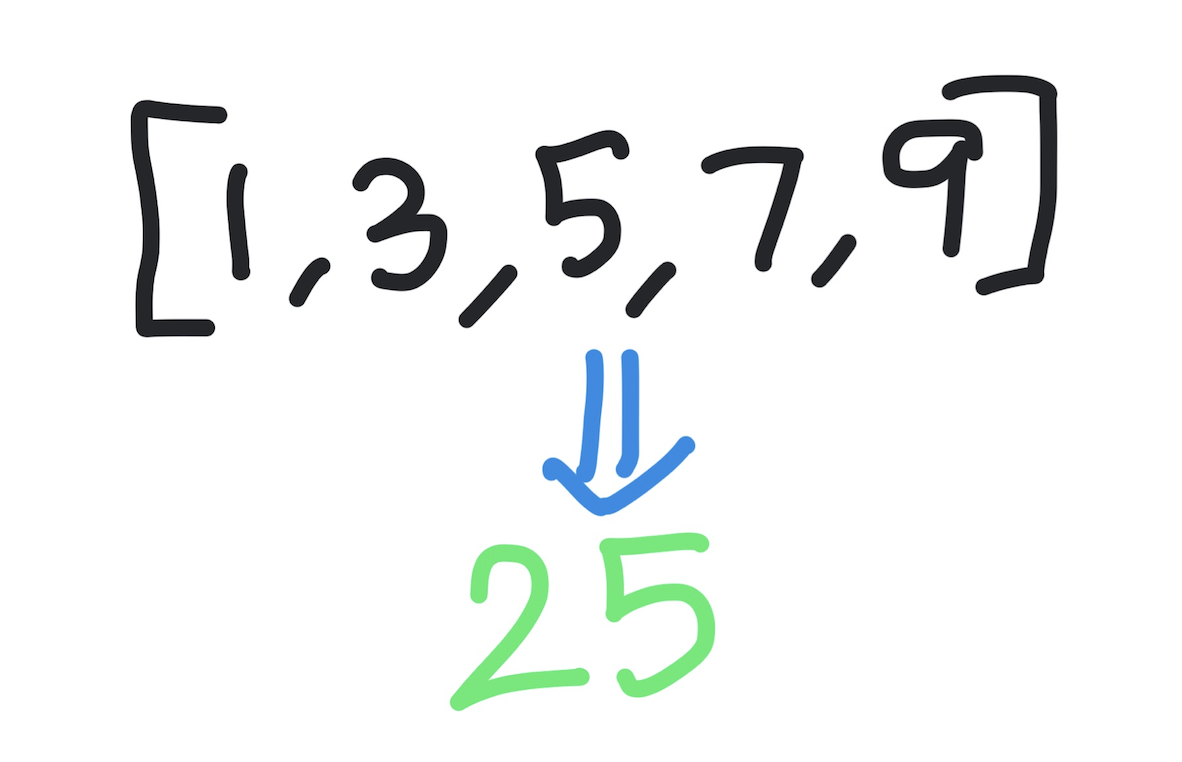
As shown above, numbers is a list containing the first five odd natural numbers. The sum function does what you think it does, namely add up these numbers and produce the output:
25
Note that you can use other iterables in place of a list. Let us adapt the above code to work with lists, sets, and tuples:
numbers_list = [1,3,5,7,9]
print(sum(numbers_list))
numbers_set = {1,3,5,7,9}
print(sum(numbers_set))
numbers_tuple = (1,3,5,7,9)
print(sum(numbers_tuple))
The output is, as expected:
25
25
25
Let us also try it with a dictionary. In the below dictionary, keys are the indices and the values are the numbers that we had before, i.e., the first five odd positive integers.
numbers_dict = {1: 1,2: 3,3: 5,4: 7,5: 9}
print(sum(numbers_dict))
The output is:
15
Whoa - what happened? Why did we get 15 instead of 25? This is because by default the sum() function applied to a dictionary operates on its keys, not its values. If you wish the sum() function to operate on the values, you must do:
numbers_dict = {1: 1,2: 3,3: 5,4: 7,5: 9}
print(sum(numbers_dict.values()))
This produces the output we are looking for:
25
Using the Python sum() function with an argument
In each of the above code, you can imagine the sum function taking an optional second argument which is the starting value for the sum. This value is considered to be zero by default when unspecified. You can make this explicit by:
numbers_list = [1,3,5,7,9]
print(sum(numbers_list,0))
numbers_set = {1,3,5,7,9}
print(sum(numbers_set,0))
numbers_tuple = (1,3,5,7,9)
print(sum(numbers_tuple,0))
numbers_dict = {1: 1,2: 3,3: 5,4: 7,5: 9}
print(sum(numbers_dict.values(),0))
The output is:
25
25
25
25
If you update the code to have:
numbers_list = [1,3,5,7,9]
print(sum(numbers_list,0))
numbers_set = {1,3,5,7,9}
print(sum(numbers_set,1))
numbers_tuple = (1,3,5,7,9)
print(sum(numbers_tuple,2))
numbers_dict = {1: 1,2: 3,3: 5,4: 7,5: 9}
print(sum(numbers_dict.values(),3))
you will get:
25
26
27
28
Note that the sum() function will not work if your input contains for instance strings, eg:
numbers_list = [1,3,5,'hello',7,9]
print(sum(numbers_list,0))
will cause:
Traceback (most recent call last):
File "main.py", line 2, in <module>
print(sum(numbers_list,0))
TypeError: unsupported operand type(s) for +: 'int' and 'str'
In this blogpost, we have learnt about Python sum(), a very useful function to reduce a given iterable into a single number. How will you make use of it?
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.