Kodeclik Blog
Python's Ternary Operator
Python ternary operators are conditional expressions that evaluate to one value if the condition is true and a different value if it is false.
Let us consider a simple function to test if a number is even or false:
def iseven(num):
if num % 2 == 0:
return True
else:
return False
If we use it like this:
print(iseven(355))
print(iseven(1286))
We will get the output:
False
True
Ternary Operators are shorthand for if..else statements
We can rewrite the above if… else statement as follows:
def iseven(num):
return(True if (num % 2 == 0) else False)
For the same inputs as above, it will give the same outputs.
As you can see the ternary operator is just syntactic shorthand. If you have a conditional that says (if a do X else do Y) the ternary operator way of saying it is just (X if a else Y).
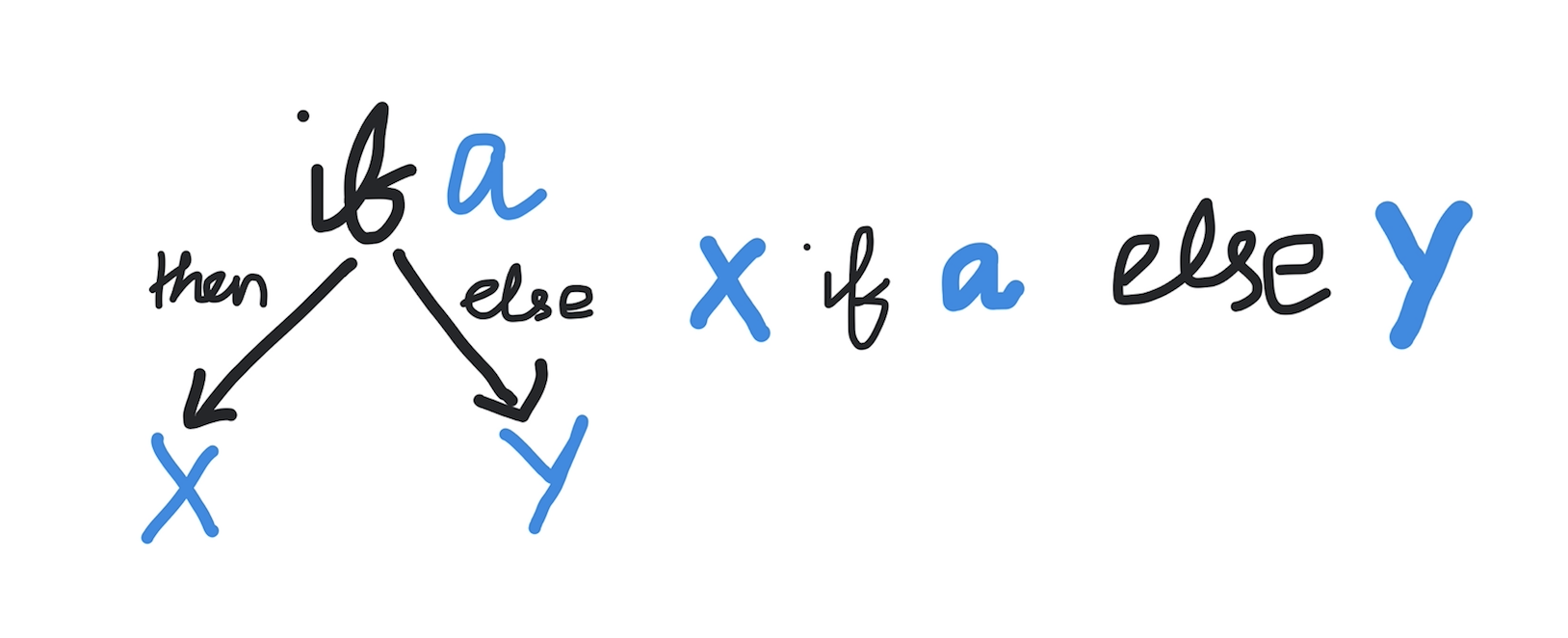
Ternary Operators to find the minimum of two numbers
Here is a ternary operator notation to find the smaller of two numbers:
def minimum(num1,num2):
return(num1 if num1 < num2 else num2)
print(minimum(56,567))
print(minimum(567,56))
This outputs:
56
56
The ternary conditional operator thus makes your program more concise and for many situations more readable. Of course if there is more program logic in the “if” and “else” parts of the conditional, then this operator can become cumbersome.
Ternary Operators to test if a user can drive
Here is a ternary operator expression to test if a user can drive by seeing if they exceed the prescribed age threshold. Some states in the US allow you to drive upon reaching age 16 while in other states the age limit is 18. We will use 18 as the limit for our example:
def CanIDrive(age):
return(True if age >= 18 else False)
print(CanIDrive(12))
print(CanIDrive(19))
This gives:
False
True
When are ternary operators not useful?
Ternary operators are cumbersome when either of two conditions are met. First if you have multiple conditions you will need to check for, then the code will get cumbersome. Second, if you have more complex logic to be executed within each condition, then ternary operators are not ideal.
Ternary Operators in Tuple Syntax
Consider the car driving example earlier. The same example can be written in so-called ternary tuple syntax as follows:
def CanIDrive(age):
return ((False, True) [age >= 18])
print(CanIDrive(12))
print(CanIDrive(19))
This produces as usual:
False
True
Note how the tuple syntax is constructed. First, you organize a tuple (False, True) that lists the “else” result first followed by the result if the conditional is true. Then this tuple is given the condition enclosed in square brackets.
Important: The else clause result is listed first in the tuple notation.
In this blog post you have learnt all about Python's ternary notation and how it can be a shorthand for if-then-else statements. Read in more detail in our blogpost on Python's inline-if.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.