Kodeclik Blog
How to remove parentheses from strings in Python
Let us suppose we have a string like so, “Hi, (Howdy), Whatsup?” We would like to remove parentheses from it, so we would like to obtain “Hi, Howdy, Whatsup?”
Method 1: String find-and-replace using the replace() method
The replace() method takes two arguments, namely the string to be found and the string to be replaced with. By default it replaces all occurrences of the original string. Here is an example to replace the left parenthesis with the empty string (and thus removing it).
mystring = "Hi, (Howdy), Whatsup?"
print(mystring.replace("(",""))
The output is:
Hi, Howdy), Whatsup?
Let us now try to replace the closing (right) parentheses as well.
mystring = "Hi, (Howdy), Whatsup?"
print(mystring.replace("()",""))
The output is:
Hi, (Howdy), Whatsup?
Wow - what happened? The reason this didn’t work is because it looks for the string “()” (and will replace it with the empty string). Instead we would like to look separately for both opening and closing parentheses and replace these occurrences with the empty string.
The way to accomplish what we need is to first replace the left parentheses and then replace the right parentheses on the output of the first operation.
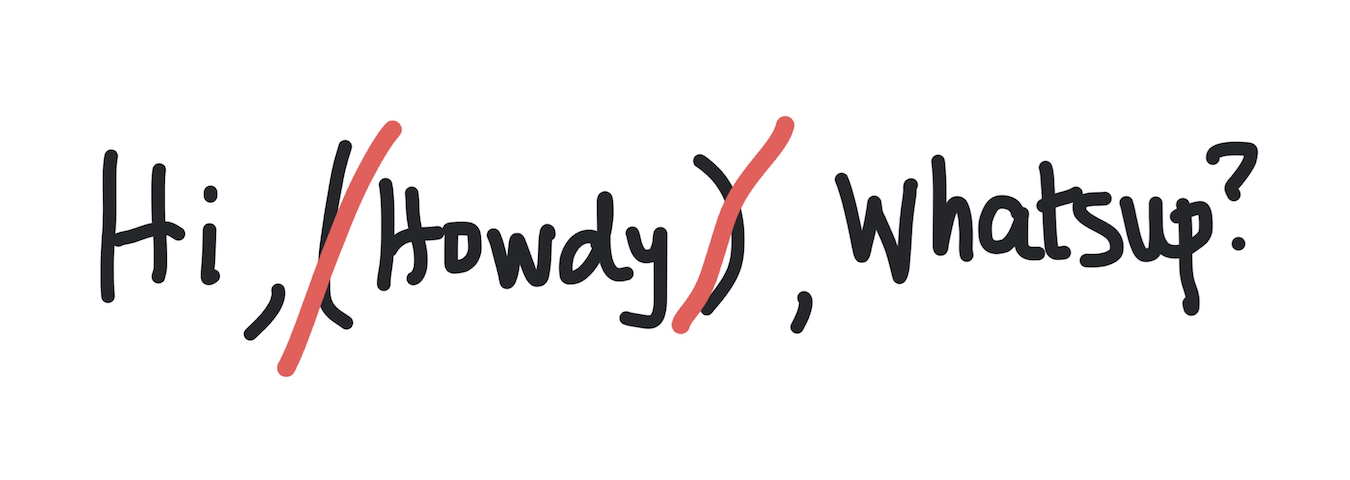
Here is the code to make this idea work:
mystring = "Hi, (Howdy), Whatsup?"
print(mystring.replace("(","").replace(")",""))
The output of this program will be:
Hi, Howdy, Whatsup?
as desired.
Method 2: sub() function from the re module
The re module is a “regular expression” module and is ideal for a lot of find-and-replace operations. In particular, the sub function from this module takes three inputs: the pattern to be searched for, the replacement string, and finally the string on which the replacements have to be made. (Note that because sub() is a function, not a method, all arguments have to be passed to it in the invocation.)
Here’s a simple example:
import re
mystring = "Hi, (Howdy), Whatsup?"
re.sub("Hi","Hello",mystring)
print(mystring)
Here we seek to replace the “Hi” with “Hello”. If you run this program, you will see that nothing happens, i.e., the output is:
Hi, (Howdy), Whatsup?
This is because the original string is not modified. re.sub() returns the modified string, so we must print that to observe any changes.
Here’s the updated program:
import re
mystring = "Hi, (Howdy), Whatsup?"
print(re.sub("Hi","Hello",mystring))
The output is:
Hello, (Howdy), Whatsup?
Going back to our original task, i.e., removing parentheses, the way to do it using re.sub() would be as follows:
import re
mystring = "Hi, (Howdy), Whatsup?"
print(re.sub("[()]", "",mystring))
The square brackets in the pattern argument of the sub() function indicate that any of the characters enclosed by the square brackets are to be replaced. The replacement string is the empty string. Finally as before mystring is the string on which these replacements are performed. The output is:
Hi, Howdy, Whatsup?
You can confirm that this works if you have multiple nested parentheses and even if you have unmatched parentheses:
import re
mystring = "((Hi,) (Howdy), ((Whatsup?)"
print(re.sub("[()]", "",mystring))
The output is again:
Hi, Howdy, Whatsup?
Method 3: Use a for loop
The final method we will see is an old-fashioned iteration through the string and dropping characters in our program:
mystring = "((Hi,) (Howdy), ((Whatsup?)"
newstring = ""
for i in mystring:
if (i not in ['(',')']):
newstring = newstring + i
print(newstring)
Here we use the “not in” operator to check for opening and closing parentheses and only if they are not present they are conatenated to a “newstring” variable which is then printed as the modified string.
So you have learnt three different methods in Python to remove parentheses from strings in Python. Which one is your favorite?
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.