Kodeclik Blog
How to check if a Python String is CamelCase
Camelcase notation is a style of naming variables in your program where every word that makes up your variable is written with an uppercase letter. For instance, “ThisIsACamelCaseString” is in camelcase but “thisisacamelcasecasestring” is not in camelcase.
There are many interpretations of what constitutes CamelCase but here we assume that i) the string must begin with a capital letter, ii) every word in the string must also begin with a capital letter, iii) there are no numbers or special characters in it.
One easy way to check if a Python string is in CamelCase is to use the “re” (regular expression) module. We will import this module, construct a suitable pattern, and use the match() function in “re” to detect if the input string matches the pattern.
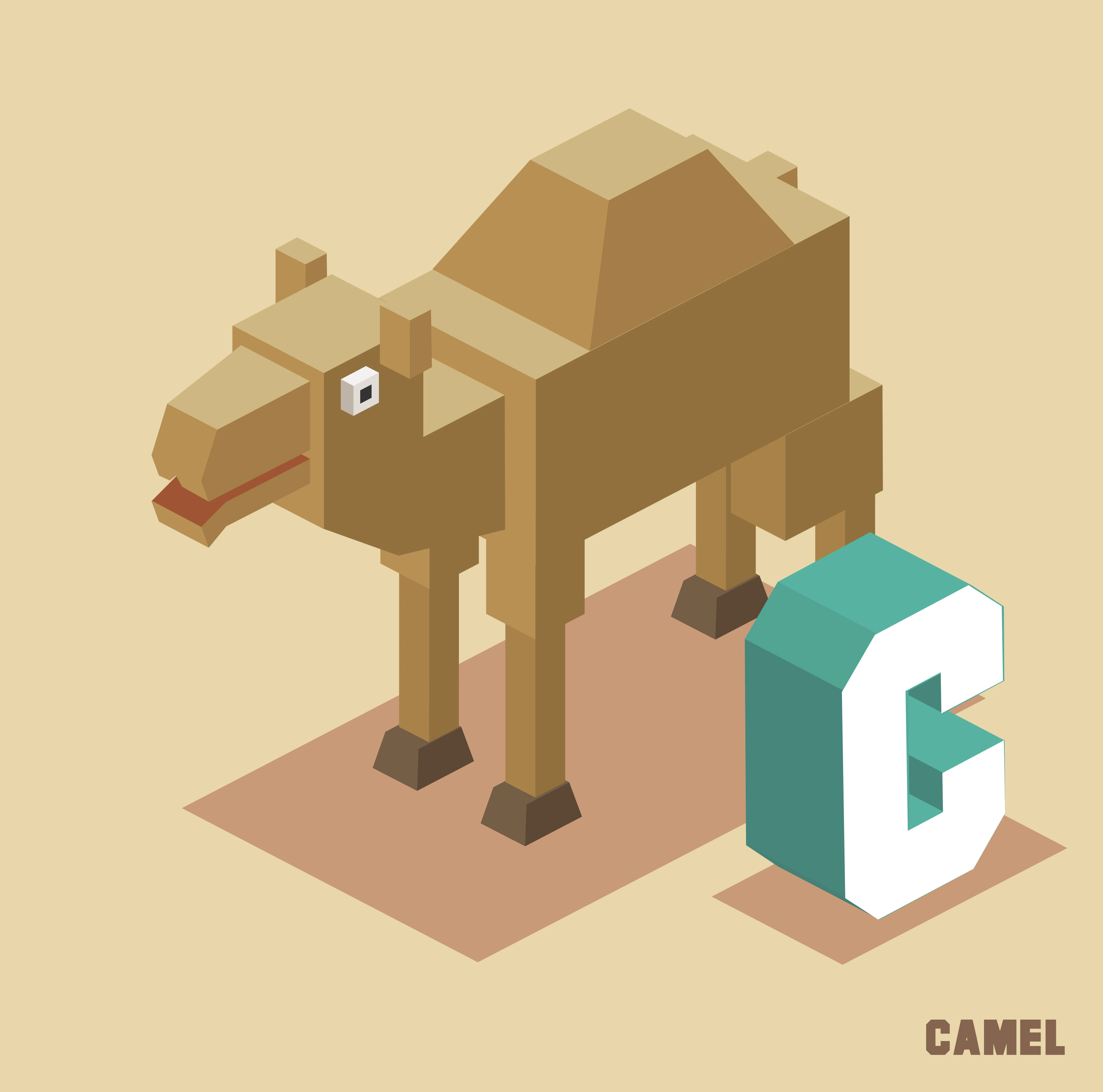
Here is a program to do so:
import re
def checkcamelcase(str):
pattern = "^([A-Z][a-z]+)*$"
if (re.match(pattern,str)):
return (True)
else:
return (False)
Note that the pattern is anchored with “^” (for beginning of the string) and “$” (for end of the string). Within these two anchors, we see that we are looking for multiple occurrences of “[A-Z][a-z]+”. (The multiple occurrences are denoted by the asterisk - “*”). The “[A-Z]” ensures that the part begins with an uppercase letter. The “[a-z]+” ensures that after the capital letter, we get one or more occurrences of a lowercase letter.
Let us try this program out:
print(checkcamelcase("KodeclikOnlineAcademy"))
print(checkcamelcase("Kodeclik"))
print(checkcamelcase("kodeclikonlineacademy"))
The output is:
True
True
False
The first two examples are true CamelCase strings. The last example string has no upper case letters, and thus the test fails.
Note that in the above code, an empty string is considered a valid example of CamelCase. If we do:
print(checkcamelcase(""))
we will get:
True
If we wish to disallow such cases, we can replace the “*” with “+” in the original regular expression, like so:
import re
def checkcamelcase(str):
pattern = "^([A-Z][a-z]+)+$"
if (re.match(pattern,str)):
return (True)
else:
return (False)
print(checkcamelcase("KodeclikOnlineAcademy"))
print(checkcamelcase("Kodeclik"))
print(checkcamelcase("kodeclikonlineacademy"))
print(checkcamelcase(""))
This program will output:
True
True
False
False
Note that the program has no understanding of “words”, i.e., which sequence of characters forms a word and when a new word begins. So if we try:
print(checkcamelcase("Kodeclikonlineacademy"))
we will get:
True
because Python thinks that there is only one word, namely “Kodeclikonlineacademy” and has no understanding that “Kodeclik”, “online”, and “academy” are separate words.
If you liked this blogpost, learn how to check for an empty string in Python.
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.