Kodeclik Blog
How can we concatenate a string to an integer in Python?
In your Python journey, you will often come across a need to concatenate a string to an integer in your Python journey. The most common situation is when you would like to print out meaningful outputs based on input variables. Here is a simple Python program to illustrate this idea:
name = input("What is your name? ")
age = input("What is your age? ")
year = 2023
print(name + " is " + age + " years old in " + year + ".")
In this program we have three variables: name, age, and year. name and age are initialized to string variables whose values come from the user. year is an integer variable pre-set to 2023. Finally, we would like to print a message giving useful information summarizing the variables.
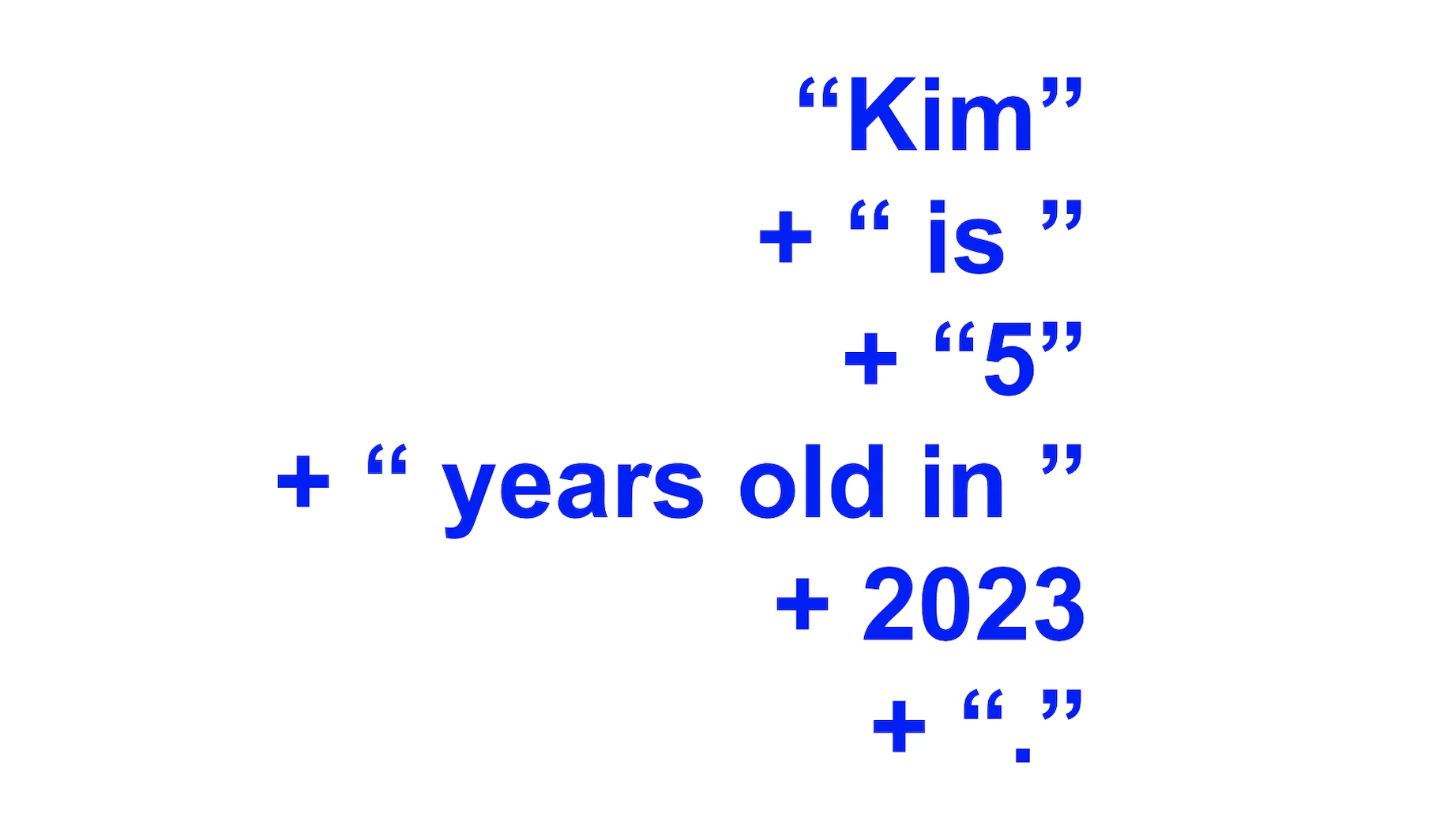
If we run this program with sample inputs like:
What is your name? Kim
What is your age? 5
We will get the output:
Traceback (most recent call last):
File "main.py", line 4, in <module>
print(name + " is " + age + " years old in " + year + ".")
TypeError: can only concatenate str (not "int") to str
Wow - what happened? The problem is that, as the error message says, we cannot concatenate the year (an integer) to a string (the rest of the parts of the print statement). We have to somehow figure out how to concatenate a string and an integer.
Note that the problem is not happening because of the age: even though the input is given as “5” this is recorded internally as the string “5”, rather than an integer with value of 5. The problem is happening because of the year variable which has been set to an integer inside the program.
To concatenate a string to an integer in Python, the solution is apply the str() function on the integer to obtain a string and then concatenate the resulting string with other strings as appropriate. So we make a really small modification to our program:
name = input("What is your name? ")
age = input("What is your age? ")
year = 2023
print(name + " is " + age + " years old in " + str(year) + ".")
The output now looks like:
What is your name? Kim
What is your age? 5
Kim is 5 years old in 2023.
Thus the str() function is very useful for concatenating strings and integers in Python. This function converts its input into a corresponding string representation.
Note also here that “+” is an overloaded operator. In the context of the programs above, it means (string) concatenation. If both sides of the “+” are integers or floats, then the symbol is taken to mean numeric addition.
In general, it is good practice, before concatenation, to be conscious of the variables you are working with, their types and which ones need to be typecast (using str()) before concatenation. For integers and floating point decimal values make sure to use str() before concatenation!
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.