Kodeclik Blog
How to convert a list to a tuple in Python
In Python, lists and tuples are two commonly used data structures. While lists are mutable, tuples are immutable, meaning their elements cannot be changed once defined. Sometimes, you may need to convert a list to a tuple or vice versa to suit your specific programming needs. In this blog post, we will explore three methods to convert a list to a tuple in Python, along with some practical use cases.
Method 1: Typecasting using tuple()
Let us assume we have a list such as [‘January’, 1, 31] and wish to convert it into a tuple (perhaps signifying that January is the 1st month and has 31 days).
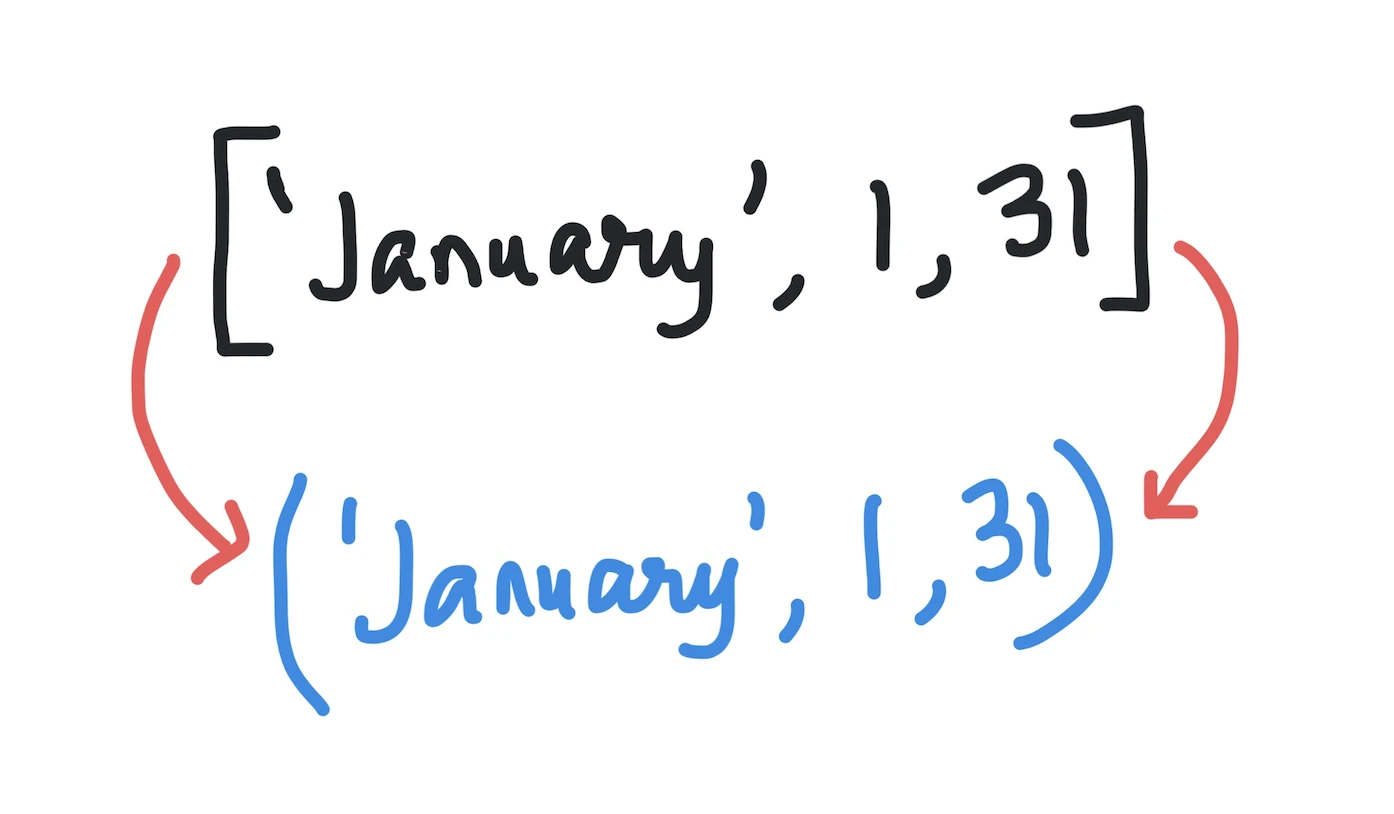
The simplest and most straightforward way to convert a list to a tuple is by using the built-in tuple() function. The tuple() function takes an iterable (such as a list) as its argument and returns a tuple containing the elements of the iterable.
Here is how it works for our example:
month_as_list = ['January', 1, 31]
month_as_tuple = tuple(month_as_list)
print(month_as_tuple)
The output is:
('January', 1, 31)
Method 2: Using a list comprehension
The second approach still uses the tuple() function but instead of passing the list as a whole uses a list comprehension to pass on one element of the list at a time:
month_as_list = ['January', 1, 31]
month_as_tuple = tuple(x for x in month_as_list)
print(month_as_tuple)
The output is still:
('January', 1, 31)
Method 3: Using the unpacking operator with a comma
The third approach uses the unpacking operator (*). Here we unpack the elements of a list and package it into a tuple (using brackets) with comma separations, indicating a tuple:
month_as_list = ['January', 1, 31]
month_as_tuple = (*month_as_list,)
print(month_as_tuple)
The output is again:
('January', 1, 31)
In summary, converting a list to a tuple in Python is a simple and versatile operation that can be achieved using the tuple() function, list comprehension with the tuple() constructor, or the unpacking operator. Whether you need to maintain data integrity, use tuples as dictionary keys, or return multiple values from a function, knowing how to convert between lists and tuples is a valuable skill. By understanding these conversion methods and their practical applications, you can enhance your Python programming skills and write more efficient and expressive code.
If you liked this blogpost, learn how to return a tuple in Python!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.