Kodeclik Blog
Finding cross products using numpy.cross()
The cross product of two vectors is another vector that is perpendicular to both the given vectors. The length of this vector is equal to the area of the parallelogram formed by the two given input vectors and its direction is determined by the so-called right-hand rule. The way the right-hand rule works is as follows: you point your index finger in the direction of the first vector and the middle finger in the direction of the second number. The cross product will then be along the direction given by your thumb.
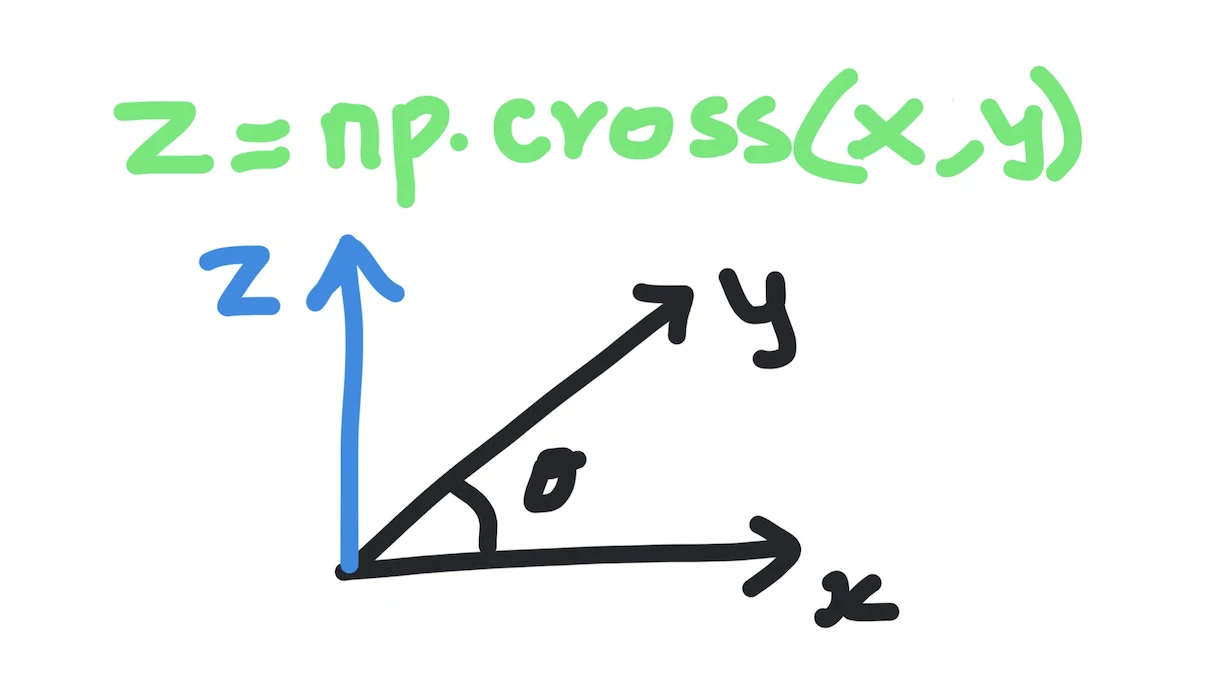
Numpy’s cross() function is a very handy function that allows users to quickly calculate the cross product of two vectors without having to write out any complex equations or code themselves. This makes it ideal for programmers who want to incorporate cross product calculations into their programs but don’t have the time or expertise to program those calculations from scratch.
To understand how numpy.cross() works it is helpful to take a 3D example and pick two vectors in one plane (e.g., the X-Y plane). Then the cross product will be along the Z-axis. Here is a simple numpy program to explore these concepts:
import numpy as np
x = [0, 1, 0]
y = [1, 0, 0]
print(np.cross(x, y))
Note that both x and y are 3D vectors but the third dimension is zero for both of them. So both vectors reside in the X-Y space. When we run this program:
[ 0 0 -1]
We see that the cross product resides along the z-axis (as it should) and pointing in the negative direction. You can verify with the right hand rule that this is correct.
In fact, if you flip the order of x and y, like so:
import numpy as np
x = [0, 1, 0]
y = [1, 0, 0]
print(np.cross(y, x))
you will get:
[0 0 1]
i.e., the cross-product is now pointing in the positive z-direction.
You can use numpy to confirm that the cross-product is orthogonal to the two given vectors. If two vectors are orthogonal (i.e., they are at ninety degrees to each other) then their dot product should be zero. We can use numpy.dot() to verify this:
import numpy as np
x = [0, 1, 0]
y = [1, 0, 0]
print(np.cross(y, x))
print(np.dot(x,np.cross(x,y)))
print(np.dot(y,np.cross(x,y)))
The output is:
[0 0 1]
0
0
indicating that the vector [0, 0, 1] is orthogonal to both x and y.
The numpy.cross() function has many variations and other arguments but the above captures the gist of how you use it. For instance you can pass on vectors in more than 3 dimensions. You can pass on arrays of vectors instead of two single vectors, and so on.
Using numpy’s cross() function makes calculating cross products much simpler than doing them manually with formulas yourself —all you need to do is pass in your two vectors as arguments and out comes your resulting vector! Whether you're working with 3D or 4D vectors or something else entirely, this approach will provide you with accurate results every time so you can focus on other parts of your project instead of worrying about getting stuck on math problems!
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.