Kodeclik Blog
How to exit a function in Python
Functions are very useful in Python to structure your code. For instance, we can write a function to print n copies of a given string:
def multi_print(message,number):
for i in range(number):
print(message)
We can invoke this function, like so:
multi_print("Hello from Kodeclik", 5)
which will give the output:
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
To exit a function properly, it is helpful to use the “return” command and furthermore to provide a return value. In the above, example, to see if you exited the function properly, try doing this:
print(multi_print("Hello from Kodeclik", 5))
This will yield:
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
None
In other words, we print the specified 5 lines of messages followed by “None” (because the function does not return any value).
Lets use a return statement and return “True” as the answer (you can return anything you desire, but here we will use True as the answer):
def multi_print(message,number):
for i in range(number):
print(message)
return(True)
print(multi_print("Hello from Kodeclik", 5))
This will yield:
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
True
If you had written
def multi_print(message,number):
for i in range(number):
print(message)
return("I am done")
print(multi_print("Hello from Kodeclik", 5))
The output would be:
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
Hello from Kodeclik
I am done
Note that the function execution stops as soon as a return statement is encountered. So if we were to do:
def multi_print(message,number):
return("I am not yet done")
for i in range(number):
print(message)
return("I am done")
print(multi_print("Hello from Kodeclik", 5))
The output will be:
I am not yet done
In other words as soon as the first return is encountered that is the value returned and no further statements are executed.
You can also use a return statement without any value, like so:
def multi_print(message,number):
return
for i in range(number):
print(message)
return("I am done")
print(multi_print("Hello from Kodeclik", 5))
This will output:
None
This is the same as if you had written “return None”. So return without an argument, return with None as an argument, or no return at all (see our first example earlier) all give a return value of “None”.
If your program has multiple branches of execution, e.g., an if statement or other complicated conditional logic, make sure all branches end with a return statement with an appropriate return value. Consider a function that checks if its input is even or odd:
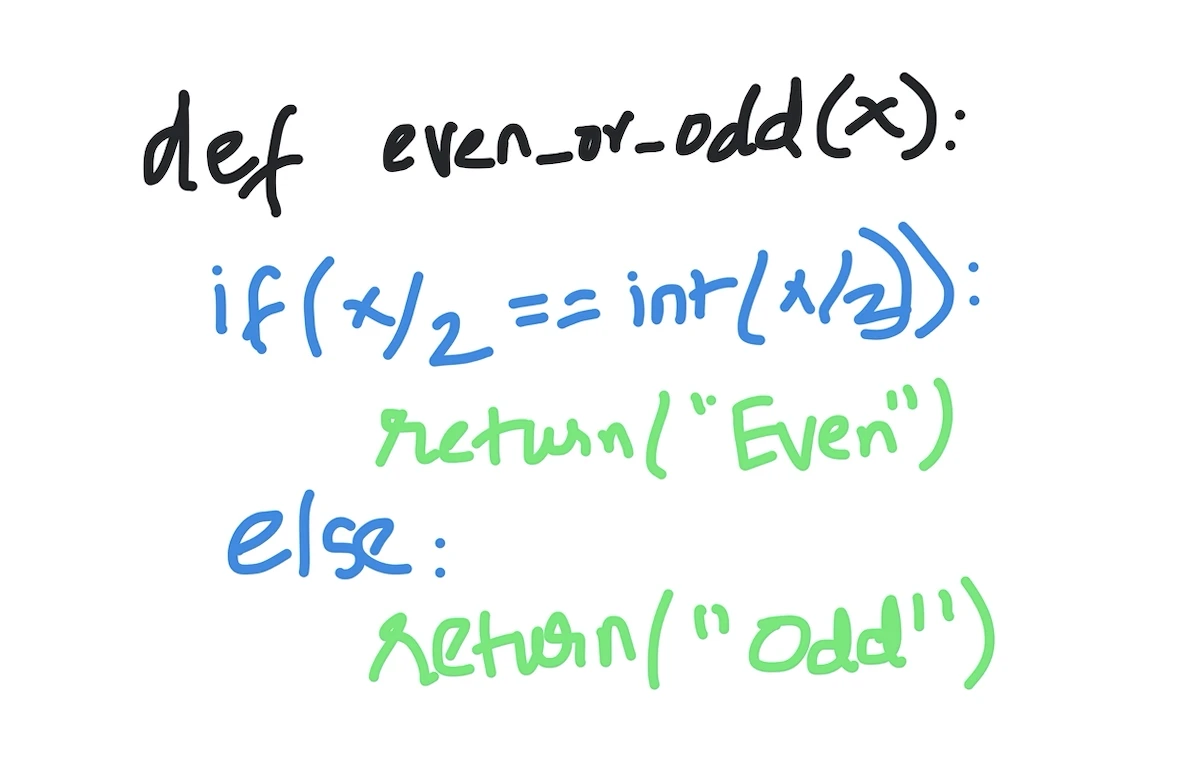
def even_or_odd(x):
if (x/2 == int(x/2)):
return("Even")
else:
return("Odd")
print(even_or_odd(7))
print(even_or_odd(8))
The output will be:
Odd
Even
Note that every branch of this program has a return statement with a suitable value. This is good programming practice for how to exit a function in Python.
So to summarize, to properly exit a function in Python, use a return statement. If your program has multiple branches of execution, e.g., an if statement or other complicated conditional logic, make sure all branches end with a return statement with an appropriate return value.
If you enjoyed this blogpost about exiting functions in Python, learn how to apply a function to a Python list. Also learn how to exit a function in Javascript.
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.