Kodeclik Blog
How to find the average of a list in Python
When working with lists of numbers in Python, it's often necessary to calculate their average. The average, also known as the arithmetic mean, provides a measure of central tendency and is widely used in data analysis, statistics, and everyday programming tasks.
In this blog post, we'll showcase three different methods for finding the average of a list of numbers in Python, from simple arithmetic to powerful built-in functions and libraries.
Method 1: Compute it yourself
In our first method, we simply write a function that sums up the numbers in a list and divides it by the count to obtain the average:
def average(l):
return (sum(l)/len(l))
numbers = [1,2,3,4,5]
print(average(numbers))
The output is:
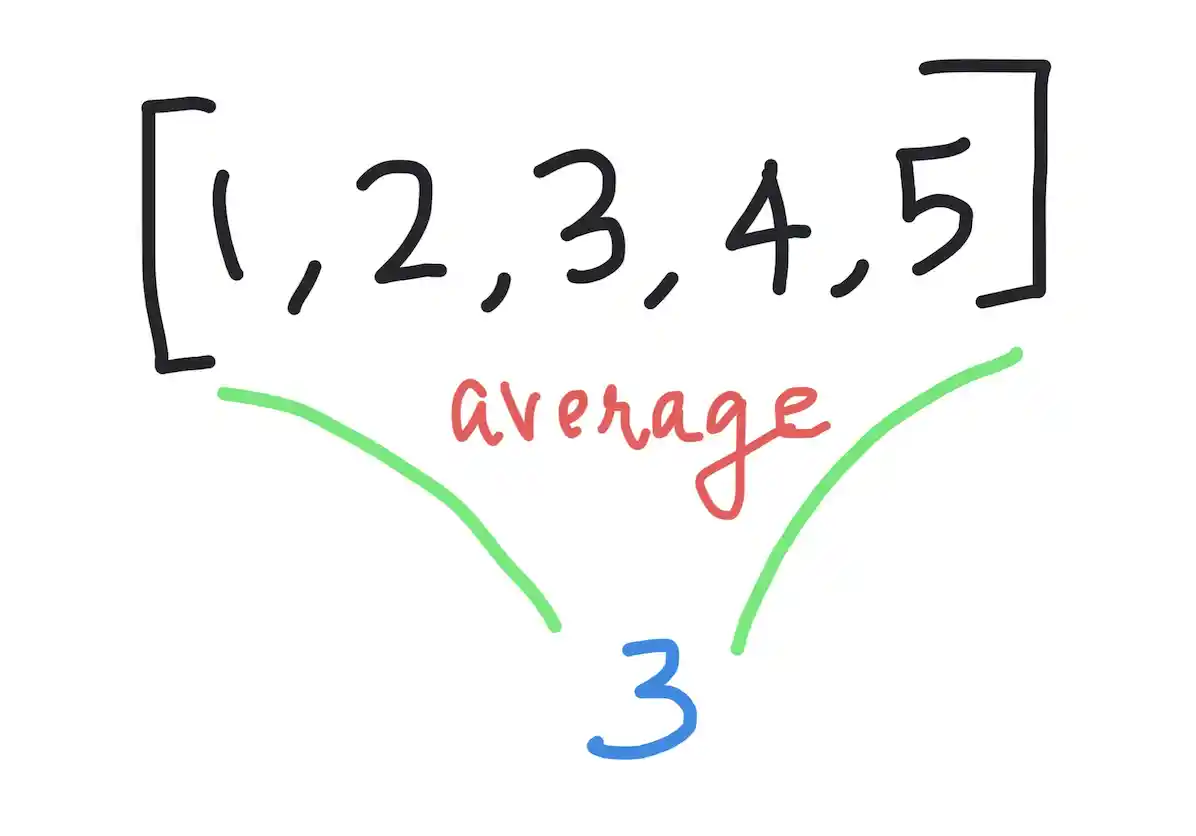
3.0
Note that the output is a floating point number, the result of the division. Also note that the above function will not work if the list is empty because you will get a division by zero. You will need to trap for that condition inside the average() function.
Method 2: Use statistics.mean()
Python provides the statistics module, which offers a wide range of statistical functions, including calculating the average. Here's an example using the statistics.mean() function:
import statistics as stat
numbers = [1,2,3,4,5]
print(stat.mean(numbers))
The output is as expected:
3
Once again note that this will not work if the list is empty and will throw an error. Nevertheless, the statistics.mean() function automatically computes the average of a list of numbers, saving you the hassle of performing manual calculations.
Method 3: Use numpy.average()
The numpy.average() function works very similar to the stat.mean() function:
import numpy as np
numbers = [1,2,3,4,5]
print(np.average(numbers))
The output is:
3.0
Again this will not work if the list is empty.
Calculating the average of a list of numbers is a common task in Python programming. In this blog post, we have seen various methods for finding the average, including basic arithmetic, utilizing the statistics module, and using the numpy module for more advanced numerical computations. By understanding these techniques, you can efficiently compute the average of any list of numbers in Python. Remember to handle edge cases, such as empty lists, to ensure your code is robust.
If you liked this blogpost, learn how to multiply a list of numbers in Python.
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.