Kodeclik Blog
How to obtain the first key in a Python dictionary
Recall that dictionaries are essentially key-value pairs and are thus useful to store information that can be represented in such forms. Here is an example of a dictionary that stores months and the number of days in each.
calendar = {'January': 31,
'February': 28,
'March': 31,
'April': 30,
'May': 31,
'June': 30,
'July': 31,
'August': 31,
'September': 30,
'October': 31,
'November': 30,
'December': 31}
The typical way to access dictionaries is via the (key,value) pair mappings. Internally, dictionaries are stored in an ordered fashion and you might find the need to obtain the first key in the dictionary. In our case the first key is “January” (and the first value is 31). There are three ways to do so.
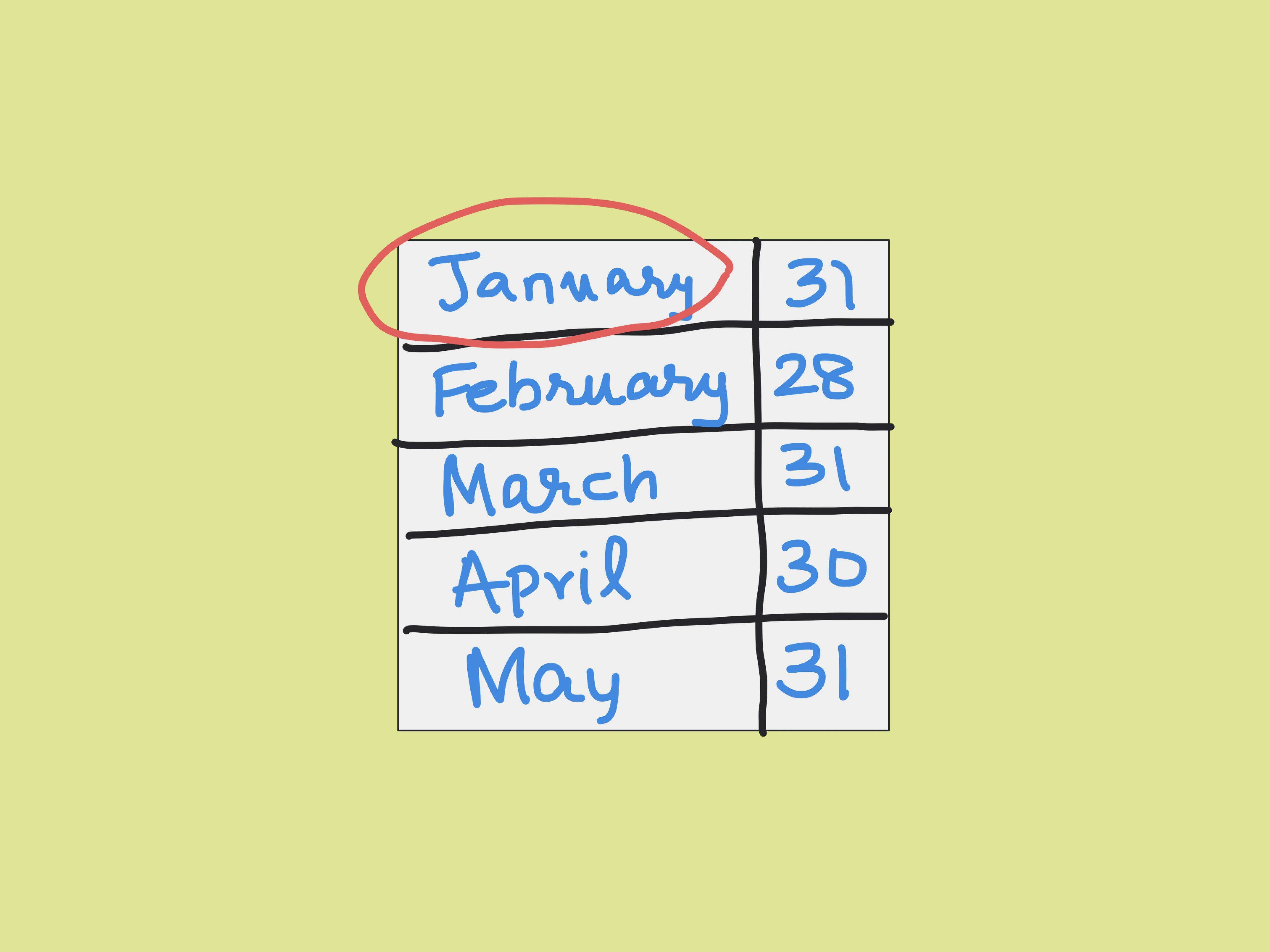
Method 1: Obtain all keys in a list and select the first element
In the first approach, we use the keys() method to obtain all the keys of a dictionary and convert it into a list using the list() constructor. Now we can simply obtain the first key by selecting the first element of this list:
allkeys = list(calendar.keys())
print(allkeys[0])
The output (for the above dictionary) is:
January
Note that you need the list() constructor step because without it, the keys() method returns an object that is not indexable. Thus, if you try:
allkeys = calendar.keys()
print(allkeys)
print(allkeys[0])
you will obtain:
dict_keys(['January', 'February', 'March', 'April', 'May', 'June',
'July', 'August', 'September', 'October', 'November', 'December'])
Traceback (most recent call last):
File "main.py", line 16, in <module>
print(allkeys[0])
TypeError: 'dict_keys' object is not subscriptable
Method 2: Setup an iterator and find the first element using next()
The second method is the more Python-ic way as it uses dictionaries in the way they are intended to be used. We use the iter() function to setup an iterator that sweeps over the dictionary and use the next() function to obtain the next element. Because the very first “next” element is the first element, we obtain what we are looking for:
print(next(iter(calendar)))
This results in:
January
Note that you can keep using next() as it simply moves the “pointer’ forward and returns the next element.
If we do:
my_iter = iter(calendar)
print(next(my_iter))
print(next(my_iter))
we will obtain:
January
February
as expected.
Method 3: Find the keys and use the unpacking operator to obtain the first key
This method is a variant of the first approach. We obtain the keys using the keys() method. Then, instead of converting the keys into a list, we unpack the keys using the unpacking operator, like so:
my_keys = calendar.keys()
first, *rest = my_keys
print(first)
The output will be:
January
as expected.
Thus, we have learnt three different ways to obtain the first key in a Python dictionary. Which one is your favorite?
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.