Kodeclik Blog
How to loop through two arrays in Javascript
You will sometimes have two arrays in Javascript and have a need to extract corresponding elements from these arrays and group them. Python has a function called zip() for this purpose. We will show you how to create a comparable looping functionality in Javascript here!
We will embed our Javascript code inside a HTML page like so:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Looping through two arrays in Javascript</title>
</head>
<body>
<script>
</script>
</body>
</html>
In the above HTML page you see the basic elements of the page like the head element containing the title (“Looping through two arrays in Javascript”) and a body with an empty script tag. The Javascript code goes inside these tags.
The wrong way to loop through two arrays
Let us assume we are given two arrays like so:
array1 = ['Microsoft','Alphabet','Amazon','Netflix']
array2 = ['MSFT','GOOG','AMZN','NFLX']
The two arrays contain company names and their ticker symbols (respectively). We wish to loop over them and print output such as:
Microsoft's ticker symbol is: MSFT
Alphabet's ticker symbol is: GOOG
Amazon's ticker symbol is: AMZN
Netflix's ticker symbol is: NFLX
Here’s a first attempt at doing it through nested loops.
<script>
array1 = ['Microsoft', 'Alphabet', 'Amazon', 'Netflix']
array2 = ['MSFT', 'GOOG', 'AMZN', 'NFLX']
array1.forEach(item1 => {
array2.forEach(item2 => {
document.write(item1 + "'s ticker symbol is: " + item2)
document.write('<BR>')
});
});
</script>
The output will be:
Microsoft's ticker symbol is: MSFT
Microsoft's ticker symbol is: GOOG
Microsoft's ticker symbol is: AMZN
Microsoft's ticker symbol is: NFLX
Alphabet's ticker symbol is: MSFT
Alphabet's ticker symbol is: GOOG
Alphabet's ticker symbol is: AMZN
Alphabet's ticker symbol is: NFLX
Amazon's ticker symbol is: MSFT
Amazon's ticker symbol is: GOOG
Amazon's ticker symbol is: AMZN
Amazon's ticker symbol is: NFLX
Netflix's ticker symbol is: MSFT
Netflix's ticker symbol is: GOOG
Netflix's ticker symbol is: AMZN
Netflix's ticker symbol is: NFLX
Oops! This is running a nested for loop with each element of the first array paired with each element of the second array. This is obviously not what is intended.
Use the forEach method with the index argument
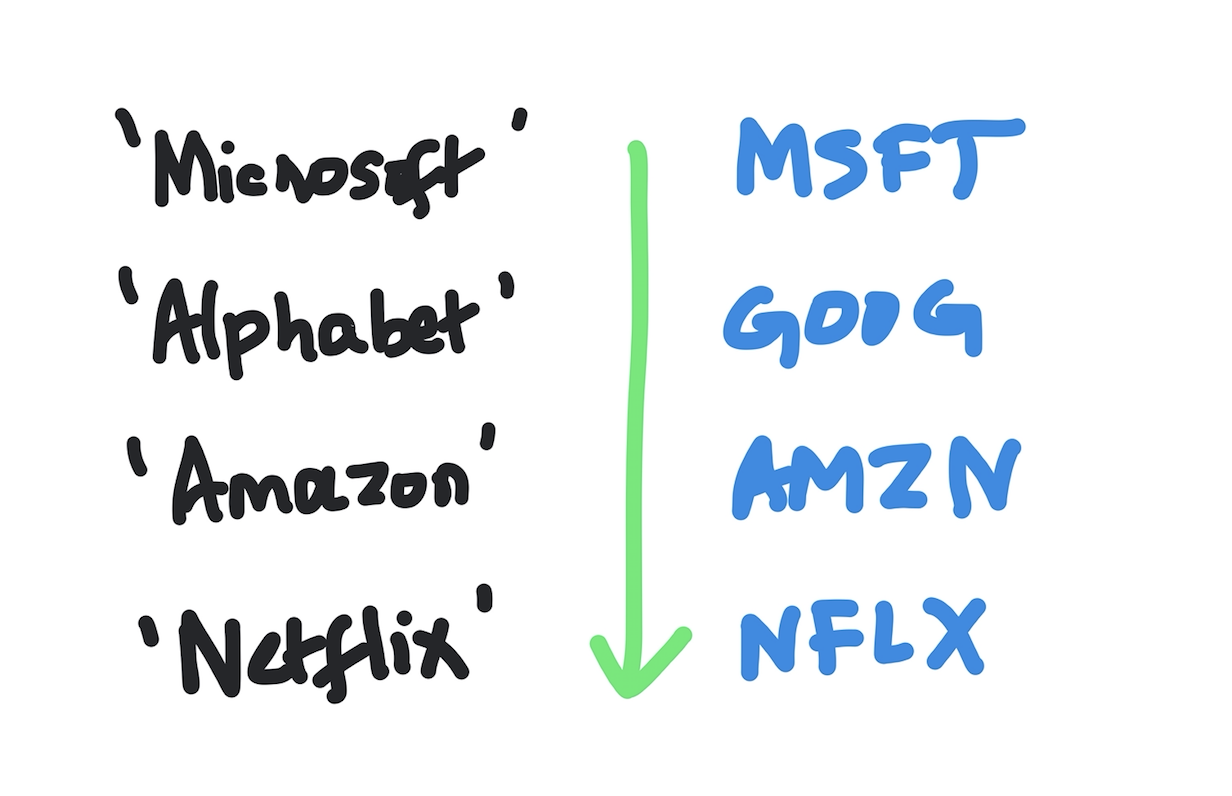
Because we are looking for corresponding elements it suffices to loop through one array using the forEach method but using two arguments (the element as well as the index). The forEach method applied to array1 can be used to return both the element and the index of the element. We use the returned index value to index into the second array and find the corresponding element. Then we use both these pieces of information to print the composite message. Here is the program to do so:
<script>
array1 = ['Microsoft', 'Alphabet', 'Amazon', 'Netflix']
array2 = ['MSFT', 'GOOG', 'AMZN', 'NFLX']
array1.forEach((ele, i) => {
document.write(ele + "'s ticker symbol is: " + array2[i])
document.write('<BR>')
})
</script>
The output of the program is, as expected:
Microsoft's ticker symbol is: MSFT
Alphabet's ticker symbol is: GOOG
Amazon's ticker symbol is: AMZN
Netflix's ticker symbol is: NFLX
Use the Javasript Array map() method
The second approach we will explore uses the map() method that works on Javascript arrays. map() goes through the array, element by element, and applies a given function on each element systematically. This is precisely what we will do here. Consider the code here:
<script>
array1 = ['Microsoft', 'Alphabet', 'Amazon', 'Netflix']
array2 = ['MSFT', 'GOOG', 'AMZN', 'NFLX']
array1.map(function (company, index) {
document.write(company + "'s ticker symbol is: " + array2[index])
document.write('<BR>')
})
</script>
Here the function being applied has two arguments (only the first argument is required). The first argument is company which is the element that we are iterating over in the array. The second argument is the index of the corresponding element. Thus, for each element in array1, we print the company followed by the ticker symbol. The output is, as before:
Microsoft's ticker symbol is: MSFT
Alphabet's ticker symbol is: GOOG
Amazon's ticker symbol is: AMZN
Netflix's ticker symbol is: NFLX
Use the collect.js library
A final approach we can use involves the collect.js Javascript library which is a very handy way to work with arrays and other data structures. However this library does not work out of the box in a browser environment and you have to use it in an elaborate tech stack like Node.js. collect.js has a zip() method very similar to Python’s zip function that will serve your needs. We will cover it in detail in a future blogpost.
Which of the three approaches above is your favorite?
Interested in learning more Javascript? Learn about the Javascript array reduce method!
Want to learn Javascript with us? Sign up for 1:1 or small group classes.