Kodeclik Blog
Javascript’s Array reduce() method
You will sometimes have an array in Javascript and have a need to reduce the array into a single value or perform other accumulated operations on it. The Javascript Array reduce() method is perfect for this purpose! Let us learn how it works.
We will embed our Javascript code inside a HTML page like so:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Javascript’s Array reduce() method</title>
</head>
<body>
<script>
</script>
</body>
</html>
In the above HTML page you see the basic elements of the page like the head element containing the title (“Javascript’s Array reduce() method”) and a body with an empty script tag. The Javascript code goes inside these tags.
Summing the elements of an array
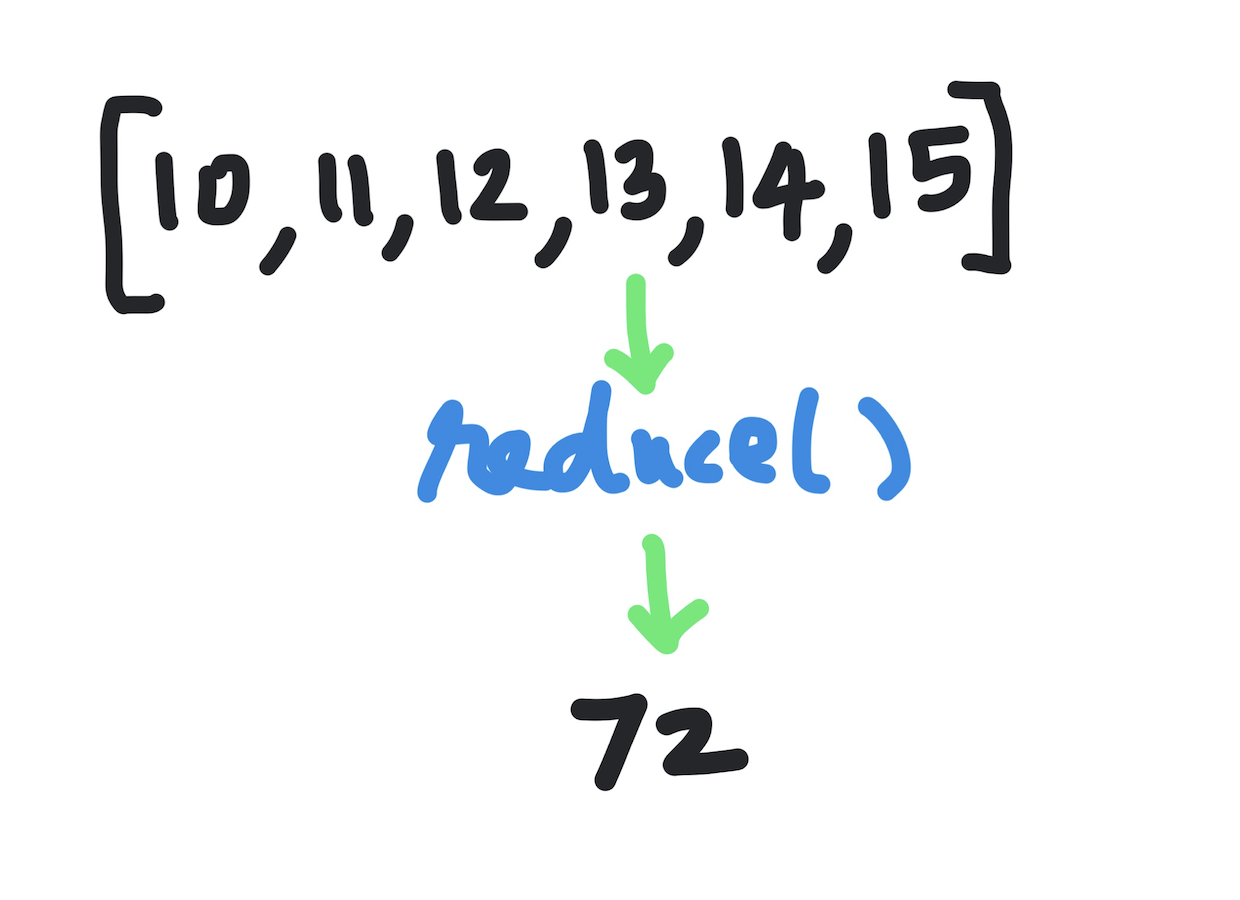
Assume we define a Javascript array of ages of students like so:
<script>
const ages = [10,11,12,11,13,15]
</script>
Our objective is to find the total of all these ages. We can iterate over the loop, of course, but let us learn how to achieve the same objective using the Array reduce() method.
The reduce() method takes a function as argument and applies it systematically over the array. The function itself has two required arguments: the first is the accumulated value and the second is the current value (element). Below is the code to sum the elements of this array:
<script>
const ages = [10,11,12,11,13,15]
const totalages= ages.reduce(function(total, currentValue) {
return total + currentValue
})
document.write(totalages)
</script>
Note that we are applying the reduce() method on the ages array and passing as an argument a function. The function takes two arguments as defined above. The semantics of the function is quite simple: it merely adds the currentValue to the (running) total. The result of applying this method is stored in “totalages” which is then printed. The output of this program is thus:
72
Optionally you can use an initial value which should be passed as an argument after the function (not as an argument to the function itself). Let us try this with an initial value of -72, so that the total should now become zero.
<script>
const ages = [10,11,12,11,13,15]
const totalages= ages.reduce(function(total, currentValue) {
return total + currentValue
},-72)
document.write(totalages)
</script>
The output will be, as expected:
0
Summing the squares of elements of an array
Let us adapt our first program to sum the squares of elements of the array instead of summing the elements. This is quite straightforward to implement:
<script>
const numbers = [1,2,3,4,5]
const sumOfSquaredValues = numbers.reduce(function(total, currentValue) {
return total + currentValue*currentValue
})
document.write(sumOfSquaredValues)
</script>
The output is:
55
because this is the sum of the squares of the first five positive integers.
Finding the average of elements of an array
Let us try to find the average of the numbers in an array. This is a very minor modification of the sum code earlier. We compute the sum and then use the length() method on the array to find the average:
<script>
const numbers = [1,2,3,4,5]
const sum = numbers.reduce(function(runningSum, currentValue) {
return (runningSum + currentValue)
},0)
document.write(sum/numbers.length)
</script>
The output will be:
3
Finding the maximum of elements of an array
Next, we will write a function to compute the maximum of the elements in an array and use it with the reduce() method.
<script>
const numbers = [1,2,5,4,3]
const max = numbers.reduce(function(runningMax, currentValue) {
return (runningMax > currentValue ? runningMax : currentValue)
},numbers[0])
document.write(max)
</script>
The function takes a runningMax and compares it with the currentValue. If the currentValue is greater (or equal) then it becomes the runningMax. The initial value for the reduce function is the first element of the array, i.e., numbers[0].
The output of the program is:
5
We hope you enjoyed learning about the Array reduce() method. Can you think of more examples?
Interested in learning more Javascript? Learn how to loop through two Javascript arrays in coordination and the Javascript instanceof operator!
Want to learn Javascript with us? Sign up for 1:1 or small group classes.