Kodeclik Blog
Javascript instanceof
We will learn about the Javascript instanceof operator which is a very handy way to understand and verify the types of objects. To explore this operator, we will write and embed our Javascript code inside a HTML page like so:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Javascript's instanceof Operator</title>
</head>
<body>
<script>
</script>
</body>
</html>
In the above HTML page you see the basic elements of the page like the head element containing the title (“Javascript's instanceof Operator”) and a body with an empty script tag. The Javascript code goes inside these tags.
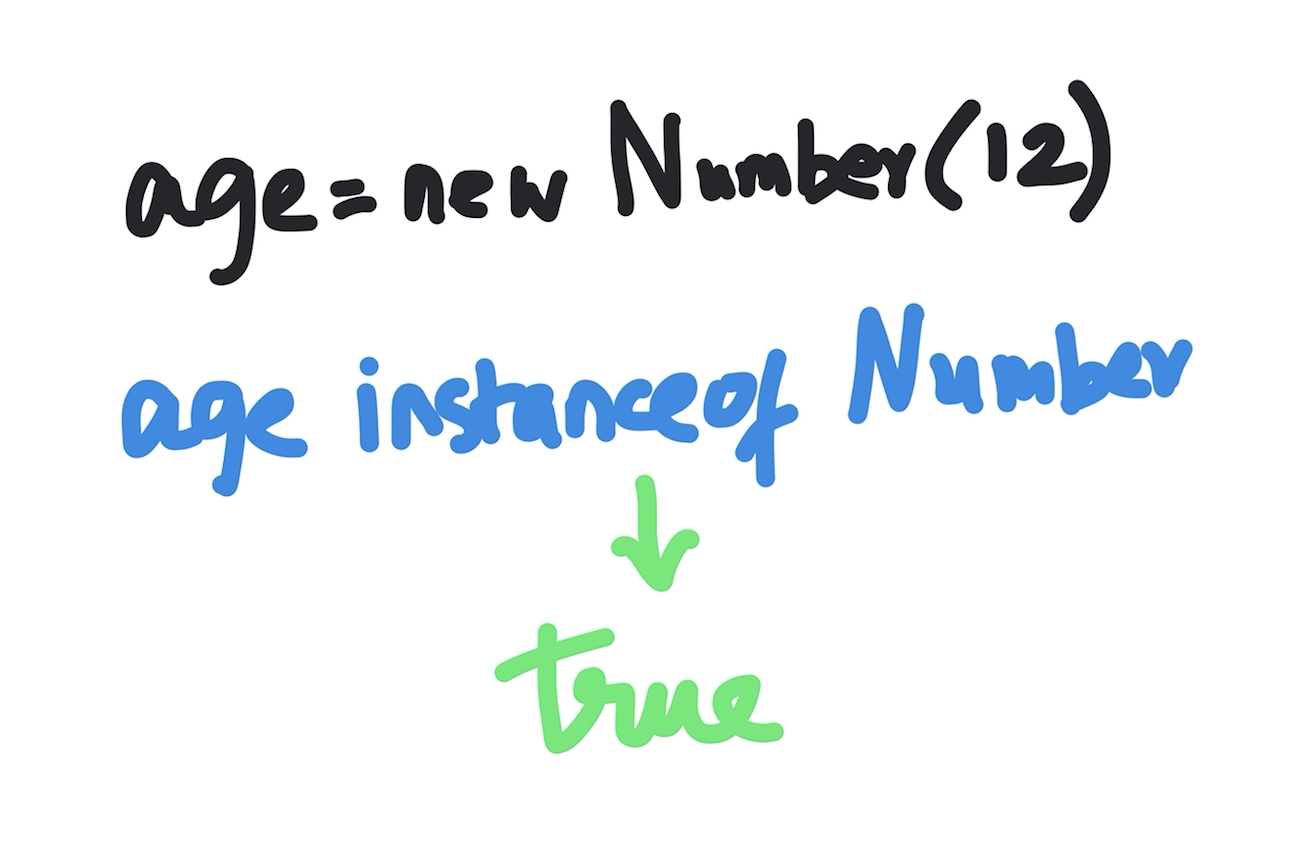
The instanceof operator can be one of the most complicated ones to understand but it need not be so. Let us try using it to understand if a given integer is an instance of the Number type.
<script>
var age = 12
document.write(age instanceof Number)
</script>
In the above code we create an integer variable (age) and assign a value of 12 to it. Then we use the instanceof operator to check if the age variable is an instanceof Number. Unfortunately this gives:
false
Why? This is because the way we have defined age it is considered a primitive and not quite a Number object created within Javascript. Let us try to use the Number() constructor:
<script>
var age = Number(12)
document.write(age instanceof Number)
</script>
The output is:
false
Unfortunately this still hasn’t worked. This is because the expression “Number(12)” does not quite create a Number object but instead converts it into a primitive value. To really construct a Number object you must use the “new” operator.
<script>
var age = new Number(12)
document.write(age instanceof Number)
</script>
The output is, finally:
true
So the moral of the story is that you must use the right, corresponding, constructor and the “new” operator to create a suitable object of the given type.
We will go through the same motions but this time apply the instanceof operator to strings. Consider the following program:
<script>
var book1 = "Harry Potter"
document.write(book1 instanceof String, '<BR>')
var book2 = String("Harry Potter")
document.write(book2 instanceof String, '<BR>')
var book3 = new String("Harry Potter")
document.write(book3 instanceof String)
</script>
The output is:
false
false
true
The output can be explained as follows. The first use of instanceof returns false because it does not use the String constructor. The second use also returns false because while it uses the String constructor it does not use the new operator. The third use works similar to our earlier example.
The same idea can be applied to other types such as the Date type. Here is an example:
<script>
var date1 = "25 May 2010"
document.write(date1 instanceof Date, '<BR>')
var date2 = Date("25 May 2010")
document.write(date2 instanceof Date, '<BR>')
var date3 = new Date("25 May 2010")
document.write(date3 instanceof Date)
</script>
The output is:
false
false
true
with the same rationale as given earlier for Strings.
To confirm that the instanceof operator also works as intended when given wrong types here is some code to test this:
<script>
var age = new Number(12)
document.write(age instanceof Number, ",",
age instanceof String, ",",
age instanceof Date,"<BR>")
var book = new String("Harry Potter")
document.write(book instanceof Number, ",",
book instanceof String, ",",
book instanceof Date, "<BR>")
var date = new Date("25 May 2010")
document.write(date instanceof Number, ",",
date instanceof String, ",",
date instanceof Date, "<BR>")
</script>
The output is:
true,false,false
false,true,false
false,false,true
As can be seen each of the newly created objects is an instanceof exactly one of the tested types each.
All Javascript objects inherit from a base type called “Object” and thus all objects are instances of Object as well. We have updated the above code to also test for instanceof w.r.t. Objects:
<script>
var age = new Number(12)
document.write(age instanceof Number, ",",
age instanceof String, ",",
age instanceof Date, ",",
age instanceof Object, "<BR>")
var book = new String("Harry Potter")
document.write(book instanceof Number, ",",
book instanceof String, ",",
book instanceof Date, ",",
book instanceof Object, "<BR>")
var date = new Date("25 May 2010")
document.write(date instanceof Number, ",",
date instanceof String, ",",
date instanceof Date, ",",
date instanceof Object, "<BR>")
</script>
The output is:
true,false,false,true
false,true,false,true
false,false,true,true
As can be seen the last entry printed in each row is “true”.
By default the way we create and initialize arrays enables Javascript to recognize that it is of type Array. Thus the following code:
<script>
var nums = [12,13,14,16]
document.write(nums instanceof Array)
</script>
returns:
true
The advantage of instanceof is that it applies not just to existing types like Date, Number, and String but also objects of new classes that you might create. Let us create a constructor for books:
<script>
function Book(title, year, author) {
this.title = title;
this.year = year;
this.author = author;
}
const myfavbook = new Book("Harry Potter and the Philosopher's Stone",
1997, "J.K. Rowling")
document.write(myfavbook instanceof Book)
</script>
The output is:
true
To make sure we have mastered the instanceof operator let us apply it to the title field of the Book object.
<script>
function Book(title, year, author) {
this.title = title;
this.year = year;
this.author = author;
}
const myfavbook = new Book("Harry Potter and the Philosopher's Stone",
1997, "J.K. Rowling")
document.write(myfavbook instanceof Book,'<BR>')
document.write(myfavbook.title instanceof String)
</script>
The output is:
true
false
As expected the second line prints “false” because the title field does not use the constructor and the new operator. Let us modify that part of the constructor:
<script>
function Book(title, year, author) {
this.title = new String(title);
this.year = year;
this.author = author;
}
const myfavbook = new Book("Harry Potter and the Philosopher's Stone",
1997, "J.K. Rowling")
document.write(myfavbook instanceof Book,'<BR>')
document.write(myfavbook.title instanceof String)
</script>
The output is:
true
true
as we desired.
We hope by now you have mastered the instanceof operator. Where will we make use of it? When we have a Javascript program with lots of types of objects it can become very handy to check for specific instances before executing specific pieces of code.
Interested in learning more Javascript? Learn how to loop through two Javascript arrays in coordination and how to make a Javascript function return multiple values!
Want to learn Javascript with us? Sign up for 1:1 or small group classes.