Kodeclik Blog
How to list the alphabet in Python
How can we generate a handy list of the alphabet (i.e., from a to z, or from A to Z) using Python? In this blog post, we will explore three different approaches to achieve this task: using a for loop, employing a list comprehension, and leveraging the string module.
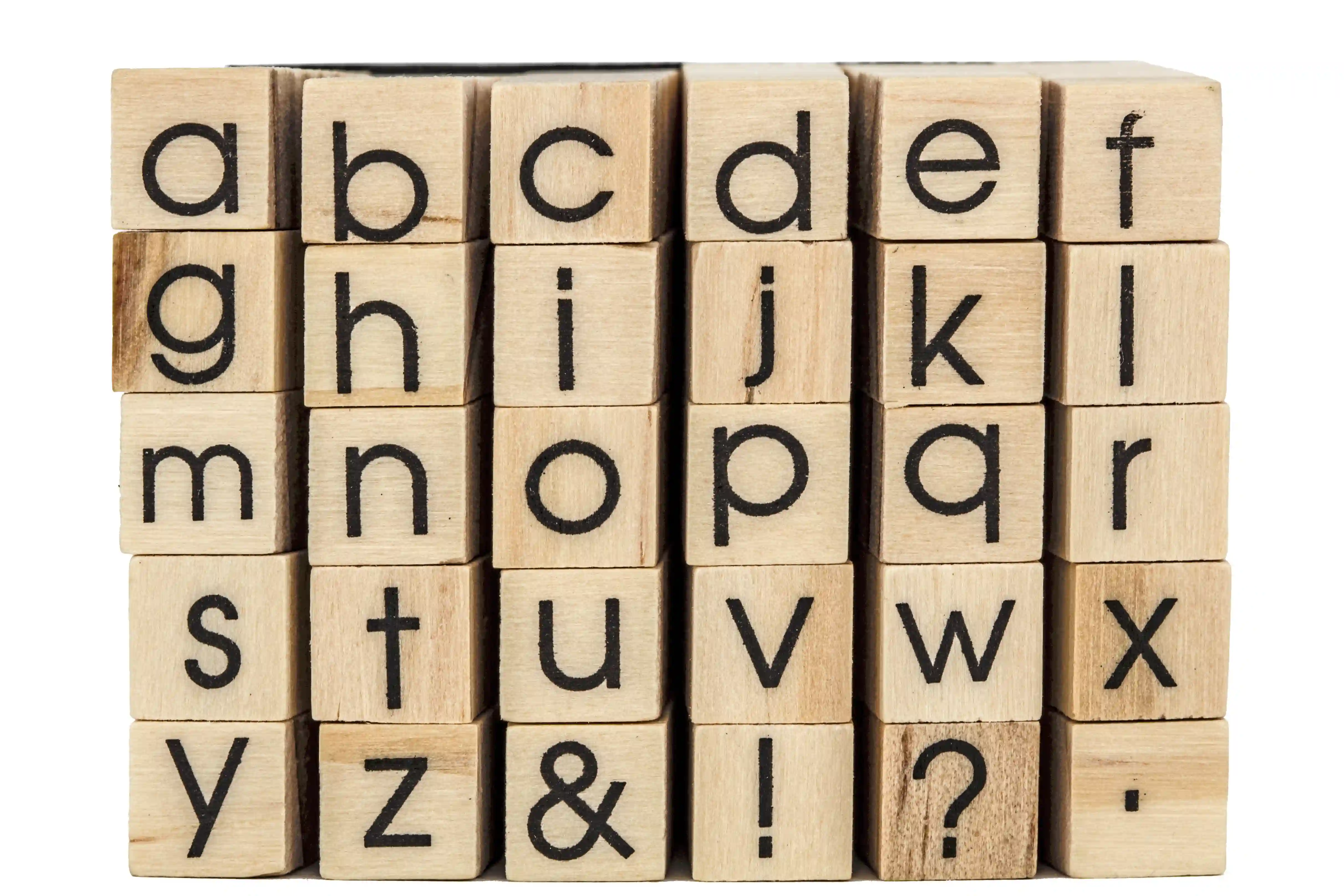
Method 1: Use a for loop to iterate from 'a' to 'z'
One of the most straightforward ways to generate an alphabet list is by utilizing a for loop to iterate from 'a' to 'z'.
alphabet = []
for x in range(ord('a'), ord('z') + 1):
alphabet.append(chr(x))
print(alphabet)
In the above code, we first initialize an empty list, and then using the built-in ord() function to convert characters to their corresponding Unicode values. By using chr() to convert the values back to characters, we can create the alphabet list efficiently.
The output is thus:
['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j',
'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't',
'u', 'v', 'w', 'x', 'y', 'z']
We can easily adapt it to create a list of uppercase characters:
alphabet = []
for x in range(ord('A'), ord('Z') + 1):
alphabet.append(chr(x))
print(alphabet)
The output is:
['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J',
'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T',
'U', 'V', 'W', 'X', 'Y', 'Z']
Method 2: Use list comprehensions
Python's list comprehensions are a concise and powerful way to create lists in a single line of code. The same approach as the for loop can be implemented using list comprehension, making the code more concise and readable.
alphabet = [chr(x) for x in range(ord('a'), ord('z') + 1)]
print(alphabet)
Notice how succinct the code is? The output is:
['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j',
'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't',
'u', 'v', 'w', 'x', 'y', 'z']
Method 3: Use the string module
Python's standard library provides a string module, which includes constants and utility functions for working with strings. We can directly access the lowercase alphabet string using string.ascii_lowercase to obtain the alphabet list.
import string
alphabet = list(string.ascii_lowercase)
print(alphabet)
Again the output is:
['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j',
'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't',
'u', 'v', 'w', 'x', 'y', 'z']
Similarly, we can access the uppercase characters using the code:
import string
alphabet = list(string.ascii_uppercase)
print(alphabet)
with the output being:
['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J',
'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T',
'U', 'V', 'W', 'X', 'Y', 'Z']
Generating an alphabet list in Python is a simple task, but notice how Python gives you multiple ways to do the same thing? This is a feature of the expressivity of the language.
In this blog post, we have explored three methods: using a for loop to iterate from 'a' to 'z', employing list comprehension, and leveraging the string module. Each approach has its advantages, and the choice depends on your personal preference. Which one is your favorite?
If you like working with lists of strings, learn how to convert a Python list to a string with comma separated values.
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.