Kodeclik Blog
Python’s math.isclose() function
Python’s math.isclose() function is a function available in the math module that is used to determine whether two floating point values are close in value. This function is important because it allows for an approximate comparison of two values. Now how close is close enough? That is left to you, the programmer. The closeness of two values is determined by you specifying a tolerance parameter when the math.isclose() function is called.
In its general form, the math.isclose() function has two parameters: x and y. These parameters represent the two values that will be compared.
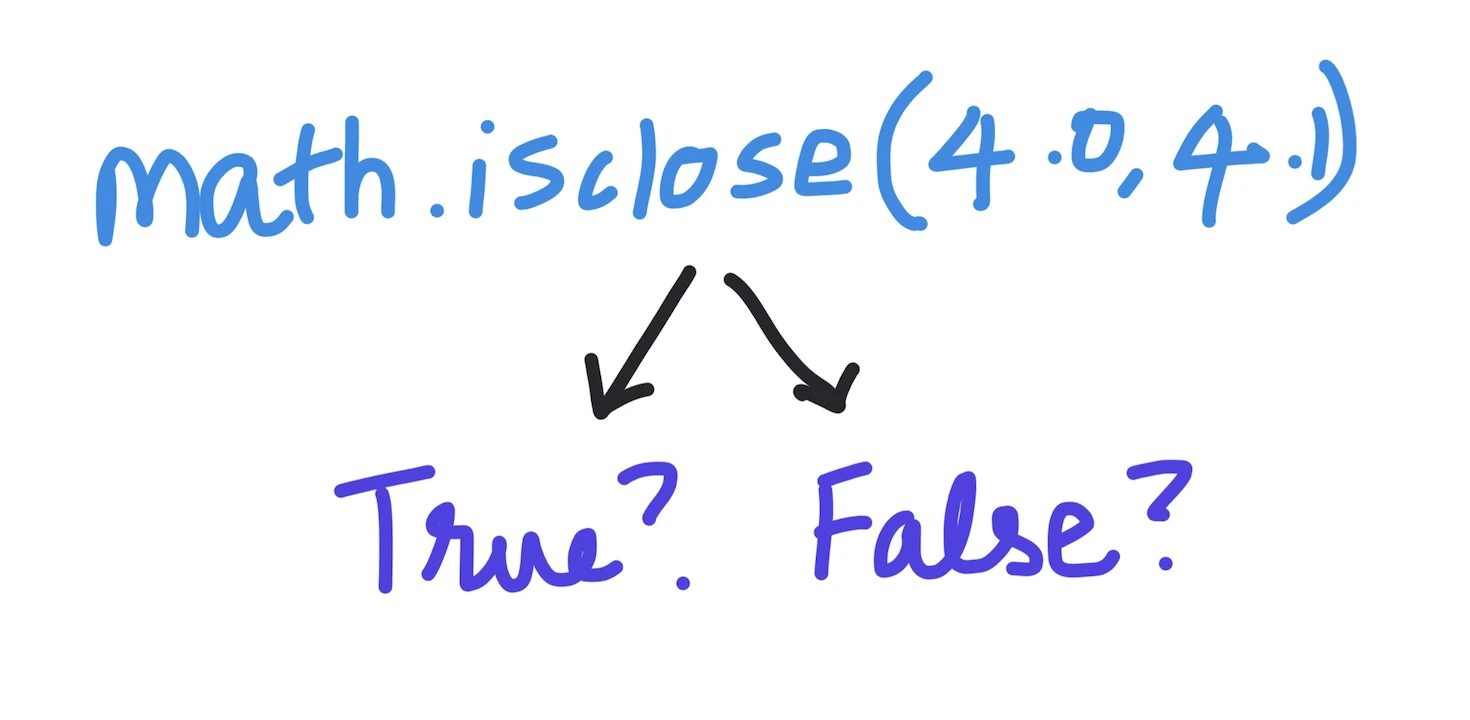
math.isclose() can optionally take two more parameters. One of them is rel_tol which denotes the relative tolerance, and the other parameter is abs_tol, which is the absolute tolerance. Both of these are settings by which we can specify the maximum amount by which the two values can differ and still be considered close.
If neither rel_tol nor abs_tol are specified, then the values default to 1e-09. Let’s take a look at some examples to see how this function works in practice.
math.isclose() returns True if the inputs are deemed to be close, and False otherwise.
Comparing two values using Math.isclose() without specific tolerances
Let us try exploring the Math.isclose() function. Consider the following code:
import math
print(math.isclose(1.0,2.0))
print(math.isclose(1.005,1.004))
The output is:
False
False
meaning these sets of numbers are not considered close.
Consider two more examples:
print(math.isclose(100000000,100000001))
print(math.isclose(1000000000,1000000001))
The output is:
False
True
Note that the first two numbers are not deemed close but the second two are. This is because they pass the absolute (or relative) closeness thresholds. In the second case, we are comparing 10^9 and 10^9+1. So the difference between them is 1. This is the absolute difference. The relative difference is 1 divided by 10^9, or 10^(-9) which fits within our relative threshold and thus math.isclose() returns True.
Comparing two values using Math.isclose() using abs_tol
Let us try adjusting the abs_tol so that we can make two values that are quite distinct be considered close enough. Consider the following code:
print(math.isclose(4.0,4.1))
print(math.isclose(4.0,4.1,abs_tol=0.5))
The output is:
False
True
In the first print statement, the values are not considered close enough by either measure (absolute or relative tolerance) and thus math.isclose() returns False. In the second statement, we are specifying an absolute tolerance of 0.5. This means if the difference between values are less than 0.5, they are considered close and thus the statement returns True.
Here are a few more examples:
print(math.isclose(4.0,4.5,abs_tol=0.5))
print(math.isclose(4.0,4.6,abs_tol=0.5))
The output is:
True
False
In the first case, the difference is 0.5 which is exactly our threshold and thus the math.isclose() function returns True. In the second case, the difference is 0.6 which exceeds our threshold and thus the function returns False.
Comparing two values using Math.isclose() using rel_tol
Now let us use rel_tol to compare two numbers. Consider:
print(math.isclose(100,101,rel_tol=0.01))
print(math.isclose(10,11,rel_tol=0.01))
The output is:
True
False
In the first case, the relative error between 100 and 101 is (101-100) divided by 100, or 0.01. Since our relative tolerance is 0.01, the values are considered close enough and thus the first statement returns True. In the second case, the relative error is 1 divided by 10, or 0.1 which is (much) higher than 0.01, and therefore this statement returns False.
To summarize, math.isclose() is very useful because it helps you set thresholds for closeness and you can then test two numbers to determine if they are close. Now that you have mastered the math.isclose() function, checkout the math.ceil() and math.floor() functions!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.