Kodeclik Blog
Named Tuples in Python
Named tuples are a type of tuple that allows you to access elements by name rather than by index. They're similar to classes in that they have attributes that can be accessed using dot notation, but they're immutable like regular tuples. This means that once created and assigned you cannot modify their values. However, you can create new instances of them with different values if you need to.
To understand named tuples, lets review “unnamed” tuples, i.e., the usual way of working with tuples in Python. Lets create a tuple to denote books; a book has a name, author, year, and publisher.
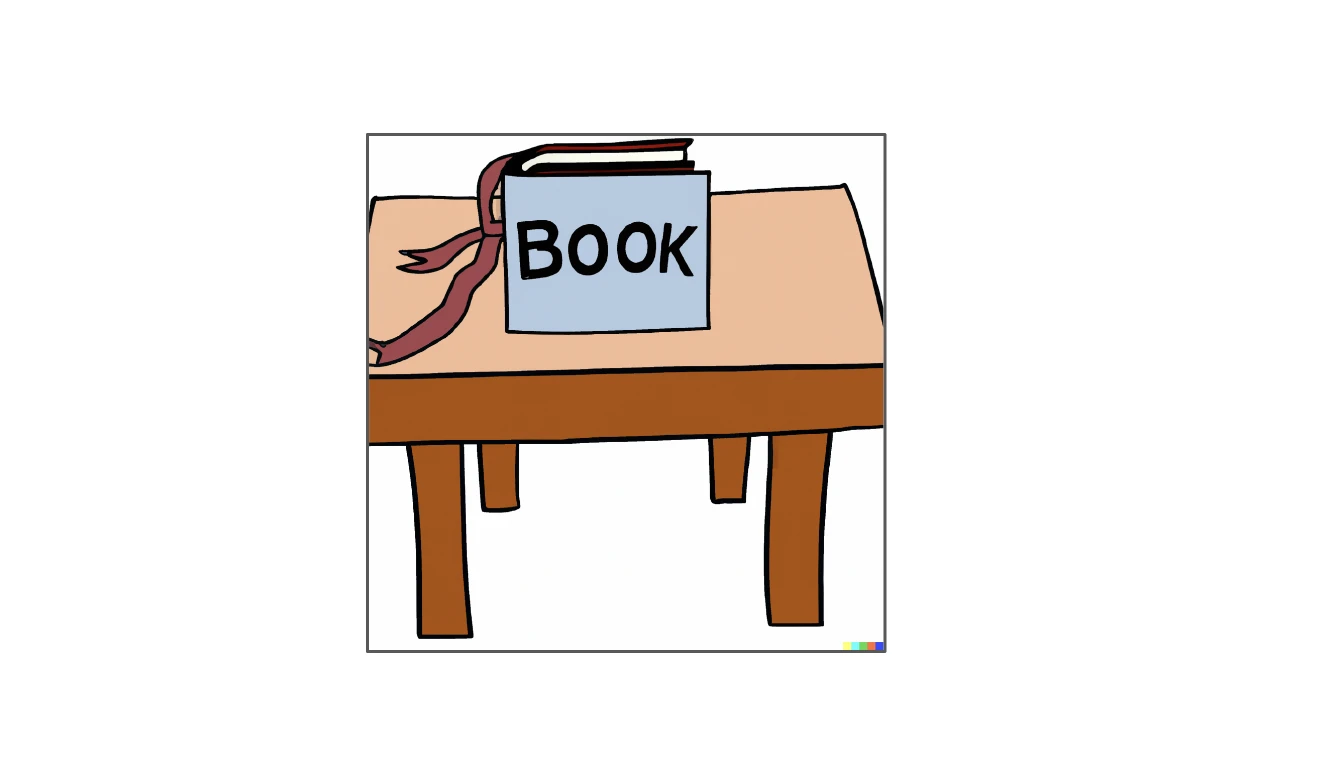
HarryPotter1 = ("Harry Potter and the Philosopher's Stone",
'J.K. Rowling',1997,'Bloomsbury')
print(HarryPotter1)
In the above program we are creating a tuple in variable “HarryPotter1” (denoting the first book in the Harry Potter series) containing four attributes of the corresponding book. The output is:
("Harry Potter and the Philosopher's Stone", 'J.K. Rowling',
1997, 'Bloomsbury')
To access individual facets of the book, we do:
HarryPotter1 = ("Harry Potter and the Philosopher's Stone",'J.K. Rowling',
1997,'Bloomsbury')
print(HarryPotter1[0])
print(HarryPotter1[1])
print(HarryPotter1[2])
print(HarryPotter1[3])
The output now is:
Harry Potter and the Philosopher's Stone
J.K. Rowling
1997
Bloomsbury
While this is useful, note that the above code is not particularly user-friendly. You will need to remember whether the author is the second field or the third field and use the corresponding index (taking care to remember that they begin at 0, not 1).
The idea of a named tuple in Python is to make the access of data elements easier so that we can refer to them by name rather than position. Named tuples are not standard in Python so we will have to import them from the collections module.
Here is basic code to illustrate how named tuples work:
from collections import namedtuple
Book = namedtuple("Book", ['name','author','year','publisher'])
HarryPotter1 = Book("Harry Potter and the Philosopher's Stone",
'J.K. Rowling',1997,'Bloomsbury')
print(HarryPotter1)
print(HarryPotter1.author)
print(HarryPotter1.publisher)
The output will be:
Book(name="Harry Potter and the Philosopher's Stone",
author='J.K. Rowling', year=1997, publisher='Bloomsbury')
J.K. Rowling
Bloomsbury
Note that the first line of the output prints the tuple with a Book() prefix surrounding it, denoting that this is a named tuple. The next two lines print the author and publisher respectively.
The interesting thing about named tuples is that you can still access them by index, eg;
print(HarryPotter1[0])
yields:
Harry Potter and the Philosopher's Stone
But recall that named tuples are immutable just like regular tuples, so you cannot modify the year or the author attribute once the tuple has been assigned. Thus the following code:
from collections import namedtuple
Book = namedtuple("Book", ['name','author','year','publisher'])
HarryPotter1 = Book("Harry Potter and the Philosopher's Stone",
'J.K. Rowling',1997,'Bloomsbury')
HarryPotter1.year = 1998
will yield:
Traceback (most recent call last):
File "main.py", line 6, in <module>
HarryPotter1.year = 1998
AttributeError: can't set attribute
In conclusion, named tuples are a type of Python tuples that allows you to access elements by name as well as by index. They are similar to classes in that they have attributes that can be accessed using dot notation; however, they're immutable like regular tuples. When working with large programs where you need to be able to access data elements by both name and index frequently, named tuples can be very useful.
If you liked this blogpost, learn about unpacking tuples in Python.
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.