Kodeclik Blog
How to pass a list as a command-line argument to a Python program
We are used to Python programs being self-contained but some times the inputs to a Python program can come from outside the program, e.g., from the user at invocation time. Such inputs are given as command line arguments to the Python program.
The argparse module provides a powerful way to parse command-line arguments. In this blogpost, we will explore the ArgumentParser class, focusing specifically on using it to handle lists of arguments.
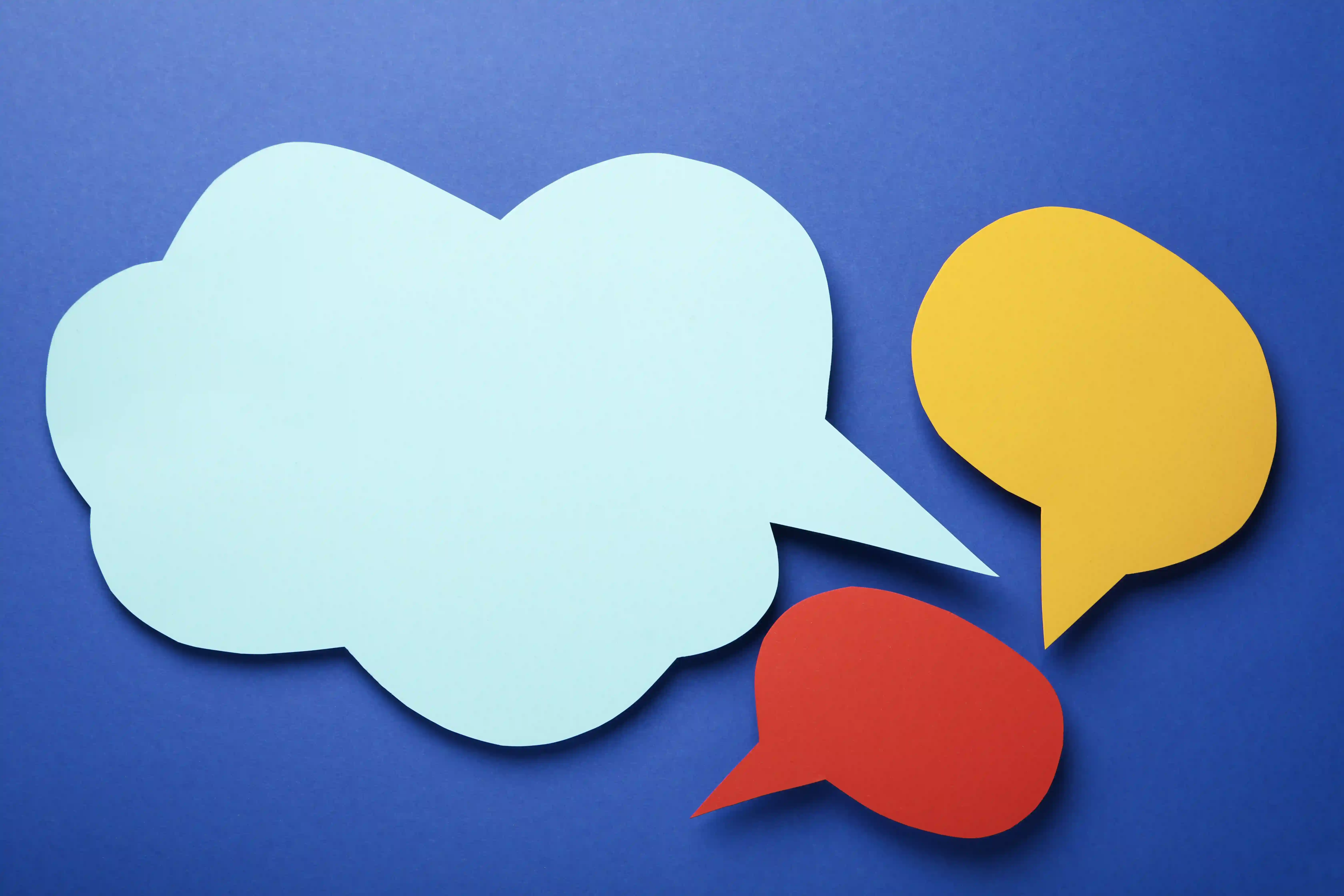
Let's start by understanding the basic usage of the ArgumentParser class without considering list arguments. We will create a simple Python script that takes two integers as command-line arguments and prints their sum:
import argparse as a
def main():
parser = a.ArgumentParser()
parser.add_argument("num1", type=int)
parser.add_argument("num2", type=int)
args = parser.parse_args()
result = args.num1 + args.num2
print(f"{args.num1} + {args.num2} = {result}.")
if __name__ == "__main__":
main()
In the above program, we import argparse as “a”, then use it to parse the inputs from the command line. Note that after the message “Enter two numbers:”, we specify two arguments into variables “num1” and “num2” and parse it. The result is then computed and printed in a suitably formatted message.
If we run this program without any command line arguments the usual manner, we will get:
usage: main.py [-h] num1 num2
main.py: error: the following arguments are required: num1, num2
process died unexpectedly: exit status 2
Thus, we can do:
>python main.py 36 64
and this will output:
36 + 64 = 100.
Note that the program has correctly parsed the two command line inputs 36 and 64 and printed the output as 100.
Handling List Arguments to ArgParse
Often, we may encounter situations where a script requires multiple inputs of the same type. For example, a script might need to process a list of numbers, file paths, or strings. In our case, assume that instead of adding two numbers we desire to add a list of numbers, i.e., a list whose length is not predetermined. To handle such scenarios, we can use the nargs parameter of the add_argument method. The nargs parameter specifies how many arguments a specific option should consume.
import argparse as a
def main():
parser = a.ArgumentParser()
parser.add_argument("numbers", type=int, nargs="+")
args = parser.parse_args()
result = sum(args.numbers)
print(f"The sum is: {result}.")
if __name__ == "__main__":
main()
In the above code, we've changed the nargs parameter to "+", which means that the numbers argument should consume one or more values. The sum() function is then used to calculate the sum of all the numbers in the list.
Now, if we run the script with multiple numbers as command-line arguments:
>python main.py 1 2 3 4 5
we get:
The sum is: 15.
If we run it without any command line arguments like so:
>python main.py
we will get the error:
usage: main.py [-h] numbers [numbers ...]
main.py: error: the following arguments are required: numbers
because as you can see it expects at least one element in “numbers”.
Another situation where you will need an arbitrary number of arguments is in writing a program that processes multiple file paths. Each file should be opened, and its contents should be printed. It is very easy to adapt the above program to meet this need.
These are various possible values for the nargs parameter in ArgumentParser. By using the appropriate nargs value, we can effectively handle different types of command-line arguments and tailor the script's behavior to suit our specific needs.
Use nargs=”+” means that the program needs to have at least one value but can accept more than one value. Similarly, nargs="*" allows the option to have zero or more values. nargs=N specifies that the option should consume exactly N values. This is useful when the script requires a specific number of arguments for an option. Finally, nargs="?" indicates that the option can take zero or one value but not more values.
So in summary, the argparse module in Python provides a robust and user-friendly way to handle different types of arguments, including lists.
With what you have learned in this blogpost, you can now confidently use ArgumentParser to create command-line interfaces for your Python scripts!
If you liked this blogpost, checkout our post on handling boolean command line arguments in your Python program.
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.