Kodeclik Blog
Python’s file naming convention
As you begin developing more and more complex Python programs you should begin using the conventions followed by programmers to name files, functions, classes, modules, and packages. While your program will still work if you do not follow some of these conventions, it is good practice to follow the style guide laid out by the community. In other words, these are “style conventions” and do not affect the functioning of your program.
Naming Python files
Python file names (i.e., the file containing your Python program) must usually end in a “.py” extension and must not contain dashes. It is typically made up of lowercase letters (numbers, occasionally) with underscore characters (“_”) for improving readability.
For instance, if you have a program to play the “Hi Lo” game, you might want to name it as “hi_lo_game.py”.
Similarly, if you have a program to output poems, you might want to name it as “poem_writer.py”.
If you have a program to count combinatorial possibilities, you might want to name it as “count_possibilities.py”.
Naming Python functions
To name a Python function, similar to naming files, you should use lowercase letters with possibly underscore characters.
If the function is meant to be non-public then you typically name it with a beginning underscore character. Again this is a style guide and not really enforced by the Python interpreter.
Sometimes you have double leading underscores or both double leading and double trailing underscores. The former are typically used for name mangling or to define special universal class methods, respectively (out of scope for this blogpost but suffice it to say that you will not need to worry about them for now).
Here is a very simple file called count_possibilities.py that contains two functions. Note how both functions are named using lowercase letters with underscores as appropriate.
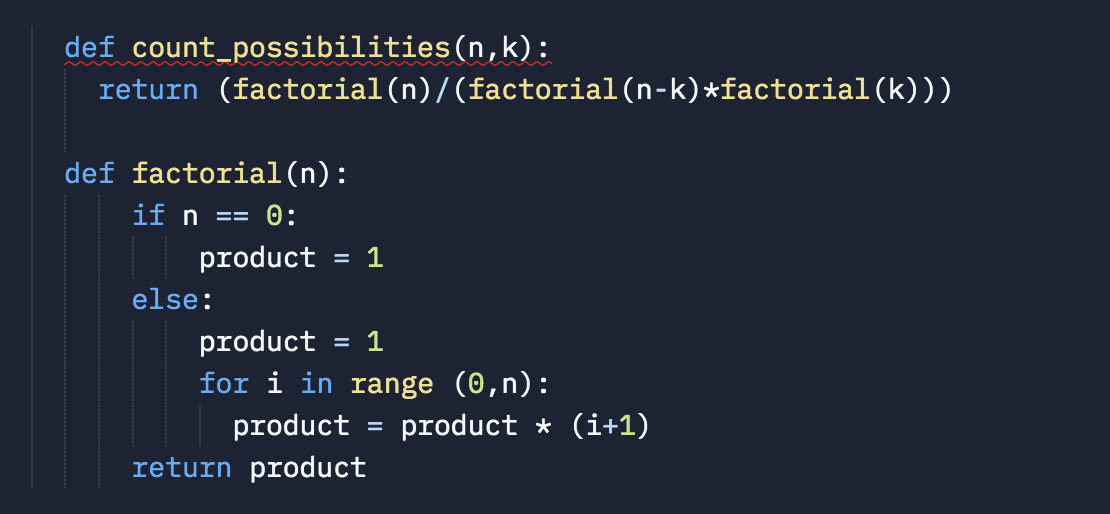
def count_possibilities(n,k):
return (factorial(n)/(factorial(n-k)*factorial(k)))
def factorial(n):
if n == 0:
product = 1
else:
product = 1
for i in range (0,n):
product = product * (i+1)
return product
Naming Python classes
Unlike files and functions, Python classes should use the CapWords style. However, note that some built-in Python classes might be in lowercase (which is a way to signal that they are built-in classes as opposed to user-defined classes). Also if you have classes meant to handle exceptions it is recommended that such Exception classes have the word Error at the end of their names. Finally, just like in the case of functions, if a Python class begins with an underscore it signals that it is usually an internal class.
Here is some sample code that defines and uses a Python class called “MathStudent”:
class MathStudent:
def __init__(self, name, grade):
self.name = name
self.grade = grade
Adam = MathStudent("Adam",6)
Emily = MathStudent("Emily",9)
Note the use of the “__init__” function that is typically used when an object is constructed (and is called automatically when an object is created).
Naming Python modules
A Python module is a collection of reusable code that is inside a file with extension “.py” and which can be imported inside another Python program. Typically a module contains a set of functions that is helpful to break down a program into manageable parts.
Similar to files and functions, modules should be named in lowercase characters with an underscore used when the name is too long. It is recommended that modules be named with just a single word.
Naming Python packages
A Python package is a collection of modules. It is typically a folder containing modules and maybe other folders each of which can contain more modules and/or folders. A package folder typically contains a file called “__init__.py” which is a way to signal to Python that this folder is a package. The contents of “__init__.py” typically contain code to be executed upon package initialization within a larger program.
For more details about Python naming conventions, see the Python style guide, specifically their section on naming conventions. Google has also released its Python style guide. Also see our blogpost discussing case sensitivity in Python.
Interested in more things Python? See our blog post on merging multiple files programmatically into a composite file. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.