Kodeclik Blog
How to import a class from another file in Python
An import statement in a Python program helps bring classes, functions, and other structures into your current program. As your programs get larger, you will find it convenient to break down your code into different files so that each file focuses on a specific functionality and you can import necessary functionalities from other files as necessary.
Here we will learn how to import a class from another file in Python.
First, let us create a main.py program containing the following:
class CashRegister:
def __init__(self, make, year, bal):
self.make = make
self.year = year
self.bal = bal
cr1 = CashRegister("Olivetti",2010,0)
cr2 = CashRegister("Honeywell",2022,0)
As you can see we have defined a CashRegister class that has make, year, and bal (balance) attributes. In the last two lines we define two cash registers, called cr1 and cr2. cr1 is an Olivetti cash register manufactured in 2010 with a balance of $0. cr2 is a Honeywell cash register manufactured in 2022 and also set with a balance of $0.
If you run this program nothing seems to happen because you have not issued any print commands etc but rest assured that your two cash registers have been created and initialized.
To confirm, we can write print() method for this class:
class CashRegister:
def __init__(self, make, year, bal):
self.make = make
self.year = year
self.bal = bal
def print(self):
print("I am an " + self.make
+" cash register made in "
+ str(self.year) + " with a balance of "
+ str(self.bal))
If we now do:
cr1.print()
we will get:
I am an Olivetti cash register made in 2010 with a balance of 0
Note that we cannot quite do print(cr1) to obtain this result. Why? Because that will default to the system print() function in Python which doesn’t quite know how to print your cash register details.
Importing a Python class from another file
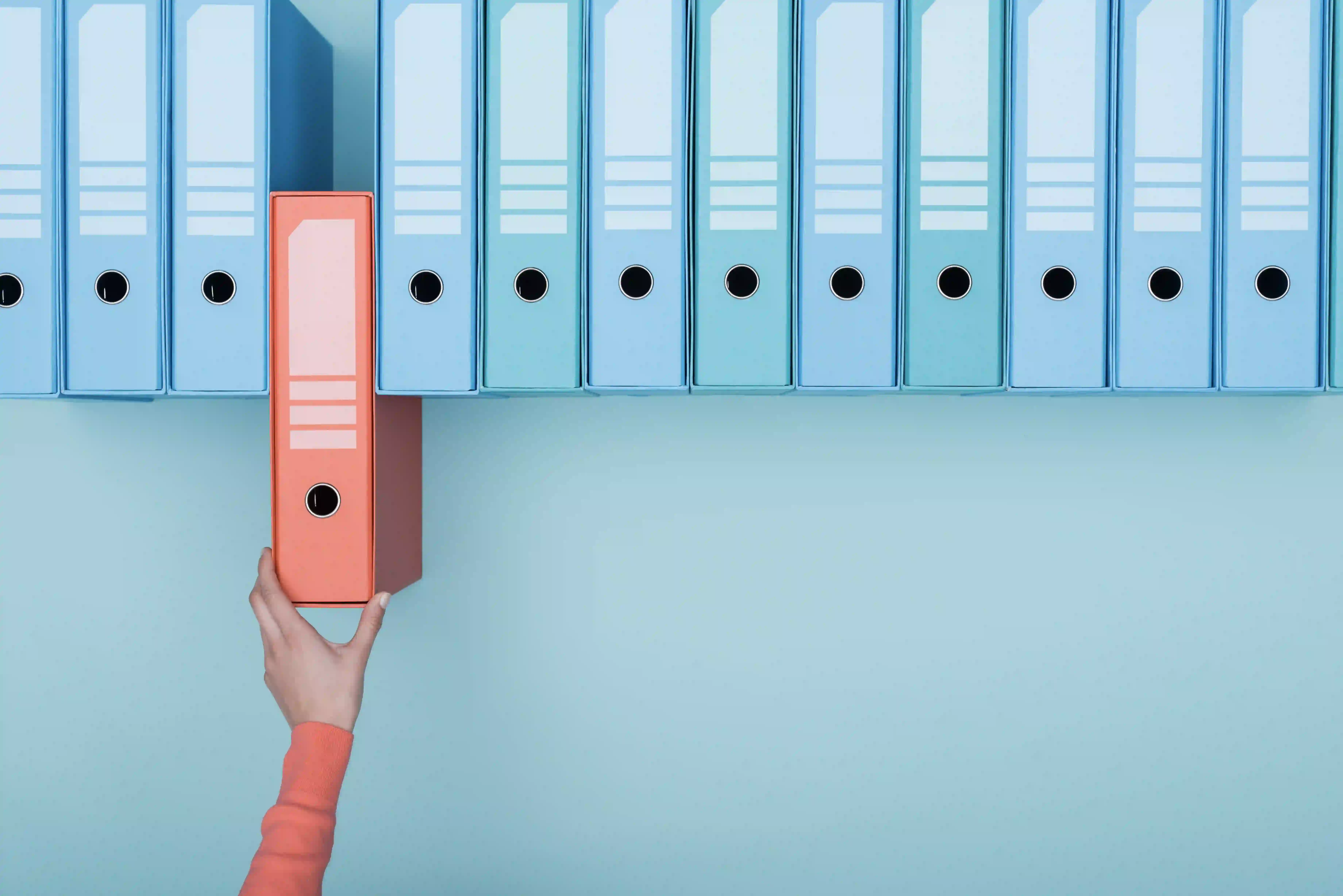
Now as programs get larger the part of the program that defines your class (i.e., CashRegister) is typically separate from the program that uses your class to define objects (like the last two lines above). We will keep these parts in two separate files as described below.
First let us create a Python file called cashregister.py and in it place our cash register definition as shown below:
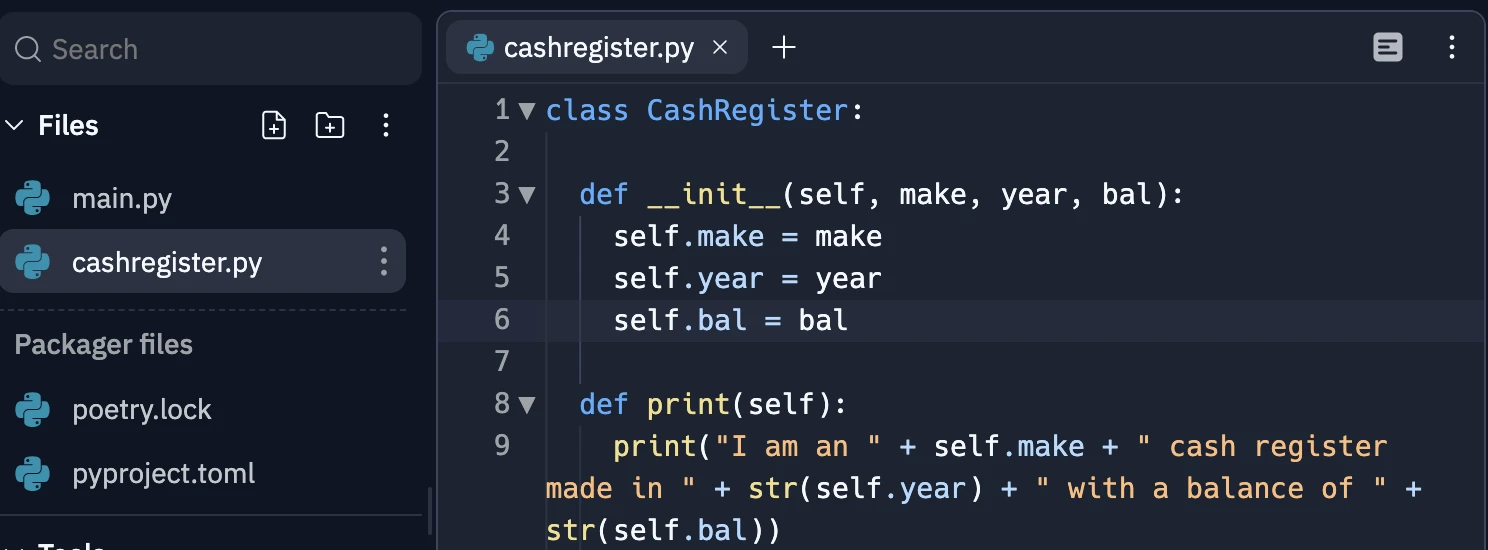
Now our main.py program will be much slimmer:
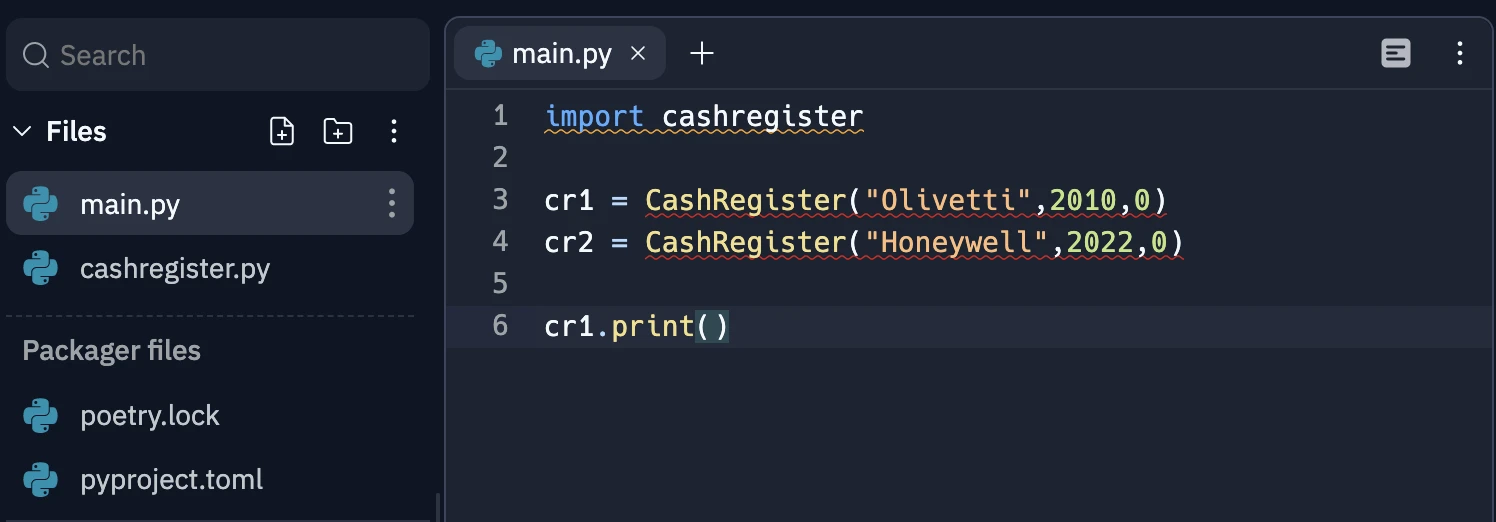
import cashregister
cr1 = CashRegister("Olivetti",2010,0)
cr2 = CashRegister("Honeywell",2022,0)
cr1.print()
Note that the first line of the program is to “import cashregister”. Let us try to run this program:
Traceback (most recent call last):
File "main.py", line 3, in <module>
cr1 = CashRegister("Olivetti",2010,0)
NameError: name 'CashRegister' is not defined
Hmm, it is complaining that “CashRegister” is not defined even though we have imported it. The problem is that even though we have imported it, we have to qualify to Python that the CashRegister we mean is the CashRegister found in the cashregister.py module. To do this, we update the program as:
import cashregister
cr1 = cashregister.CashRegister("Olivetti",2010,0)
cr2 = cashregister.CashRegister("Honeywell",2022,0)
cr1.print()
The output is now:
I am an Olivetti cash register made in 2010 with a balance of 0
Now the program works! However, this can be a bit cumbersome to do each time. One shortcut we can take is to give a shorthand name when importing a module like so:
import cashregister as cr
cr1 = cr.CashRegister("Olivetti",2010,0)
cr2 = cr.CashRegister("Honeywell",2022,0)
cr1.print()
The output is still:
I am an Olivetti cash register made in 2010 with a balance of 0
In this example, we are referring to the cashregister module as “cr” so that henceforth whenever we write “cr dot something” we are referring to functions/classes/etc found within the cashregister module.
In summary, the idea of an import statement is to get definitions of a class from another file into your current program. All experienced programmers make use of import statements. In fact in the modern world of software re-use you will often be building on code written by other programmers. In such a case, we will import modules from multiple sources (so there will be multiple import statements in your program) and use these classes and functions without worrying about how these classes are implemented. Another advantage of using import statements (even if you are the person both implementing the class and using the class) is that it helps separate these two facets, a good programming practice.
In you liked this blogpost, learn about nested classes in Python!
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.