Kodeclik Blog
Finding the lcm using Python’s math.lcm()
The least common multiple is defined as the smallest multiple that two or more numbers have in common. The LCM occurs in many practical contexts.
For instance, let us suppose you are going to a party where there will be children and you want to buy them some candies. But you are not sure whether there will be 2, or 3 or 8 children (but it will be one of these numbers). How many candies should you buy so that each child gets the same number of candies with no candies left over? This is an LCM (least common multiple) problem.
It turns out the LCM of 2, 3, and 8 is 24. This is because 24 is the smallest number divisible by 2, 3, and 8 without leaving any reminder.
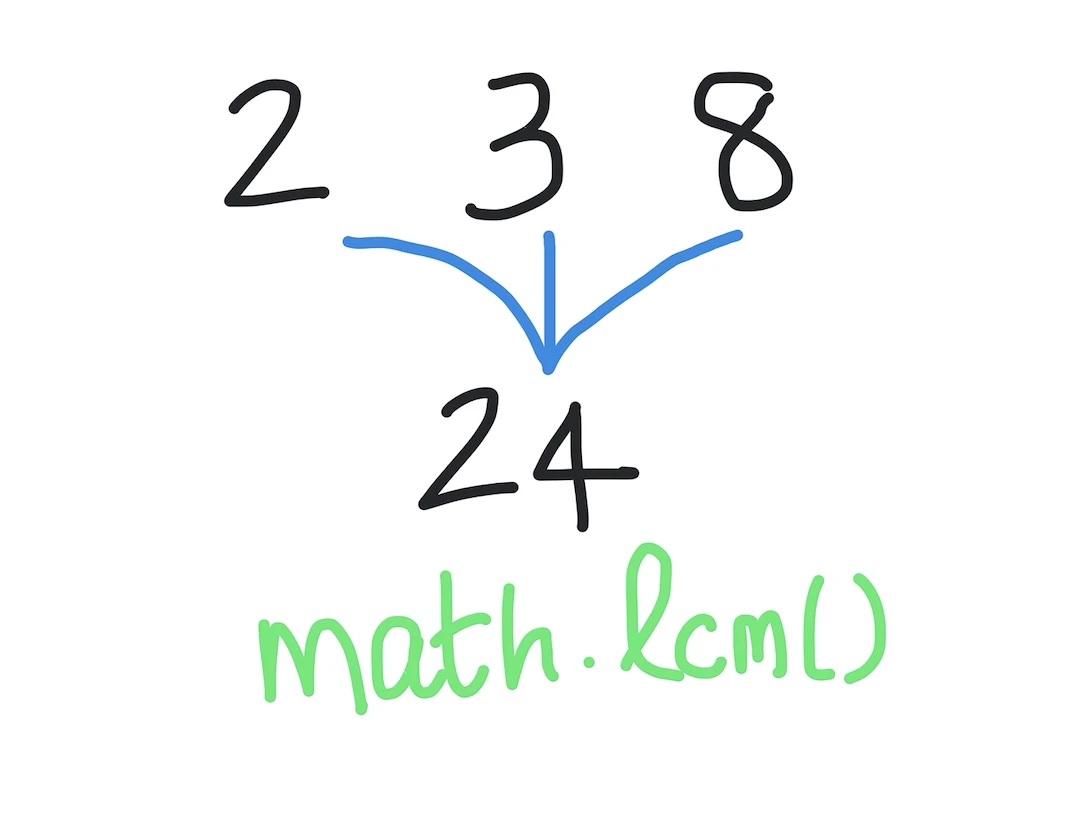
So if you purchased 24 candies it will evenly divide into 2, or into 3, or into 8, so you are covered in all cases.
Python’s math.lcm takes as many integer arguments as desired and returns the least common multiple of the arguments. If all arguments are nonzero, then the returned value is the least common multiple (LCM) of the specified integer arguments.
Here is a very simple program for the above situation:
import math as m
print(m.lcm(2, 3, 8))
The output is:
24
as desired.
Note that the number of arguments to math.lcm() can be arbitrarily long. Let us try to find the LCM of all numbers from 1 to n, for various values of n:
import math as m
for i in range(2,12):
l = list(range(1,i))
print("The lcm of " +
str(l) +
" is " +
str(m.lcm(*l)))
In the above program we are creating a loop from indices 2 to 11 (recall that the second index of the range operator is not inclusive). Inside the loop we are creating a list of numbers from 1 to 1 less than the loop index, so we are creating lists from [1], to [1,2] to [1,2,3,..,10]. Inside we are also using the deconstructing operator to unpack the list into individual arguments before passing onto the lcm() function. The output is:
The lcm of [1] is 1
The lcm of [1, 2] is 2
The lcm of [1, 2, 3] is 6
The lcm of [1, 2, 3, 4] is 12
The lcm of [1, 2, 3, 4, 5] is 60
The lcm of [1, 2, 3, 4, 5, 6] is 60
The lcm of [1, 2, 3, 4, 5, 6, 7] is 420
The lcm of [1, 2, 3, 4, 5, 6, 7, 8] is 840
The lcm of [1, 2, 3, 4, 5, 6, 7, 8, 9] is 2520
The lcm of [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] is 2520
Note that initially the LCM starts out as 1 and with each number added it usually multiplies the old LCM by the new number to obtain the new LCM. Thus, 1*2=2, 2*3=6. But when 4 is added, the LCM is not 6*4=24. It is 12. Similarly, note that the LCM of [1,2,3,4,5] and [1,2,3,4,5,6] is the same, which is 60.
Note that math.lcm() works only with integers—so no decimals or fractions allowed! Further, make sure that all arguments pass to it are non-zero. If any of the arguments is zero, then the returned value is 0. If you do not pass any arguments, lcm() returns 1.
To summarize, Python’s built-in math functions like math.lcm() make tackling complex mathematical problems easier than ever before for programmers like us! This small but powerful function can quickly calculate the least common multiple of integers in one fell swoop - saving us time and effort on our projects that require calculations involving LCMs in particular - allowing us now to focus on other aspects such as debugging or optimization instead!
If you liked learning about math.lcm, checkout Python's math.log10() function.
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.