Kodeclik Blog
Python os.remove()
The Python os.remove() method within the os module takes a filepath as argument and deletes it if it is a valid filepath. If the input happens to be a directory, an error is raised and no deletions are made.
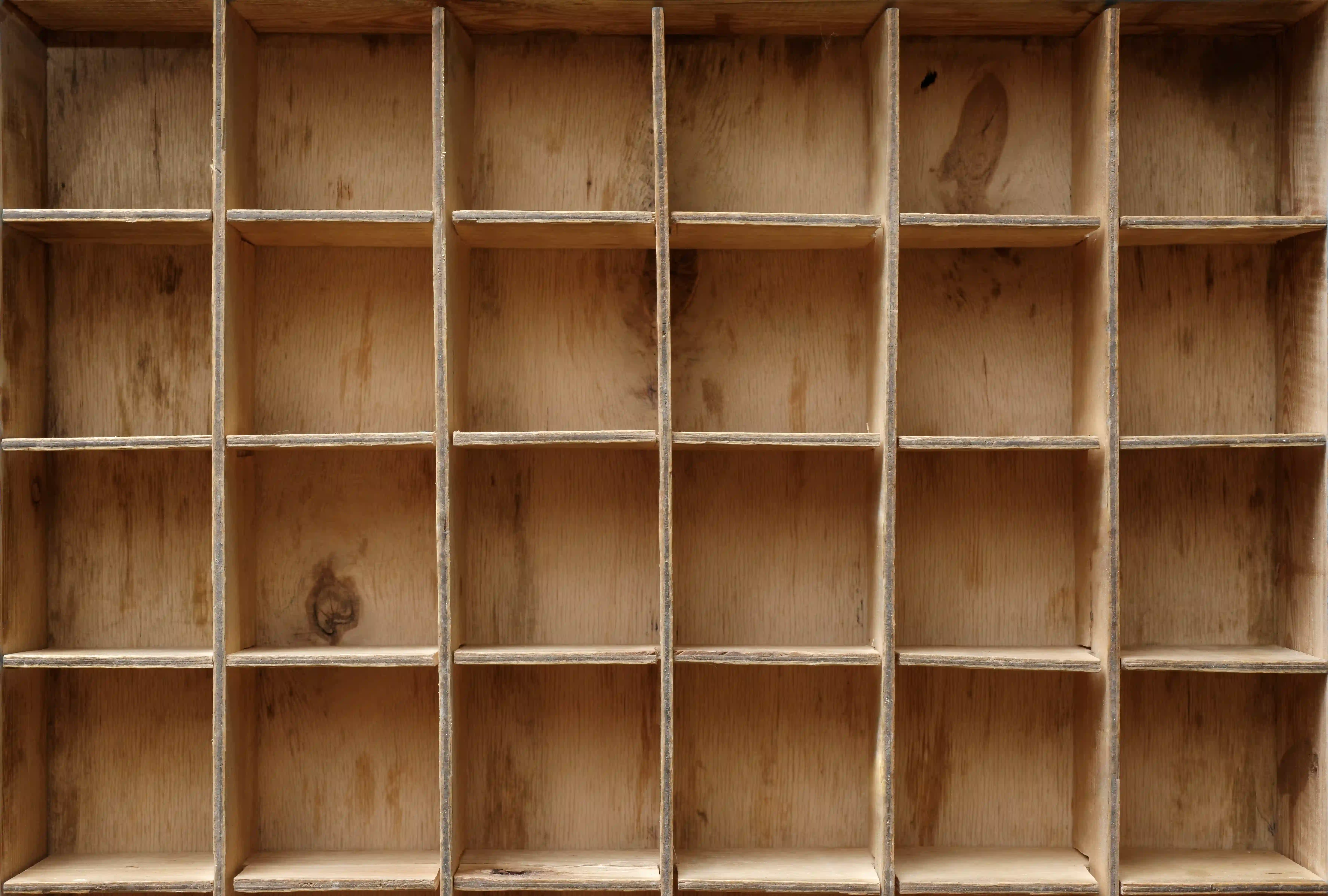
Let us explore this method by creating a repl and opening a Python file.
Now let us create a Python program in it:
import os
directory = os.getcwd()
print(directory)
To support your understanding this is how our setup looks:
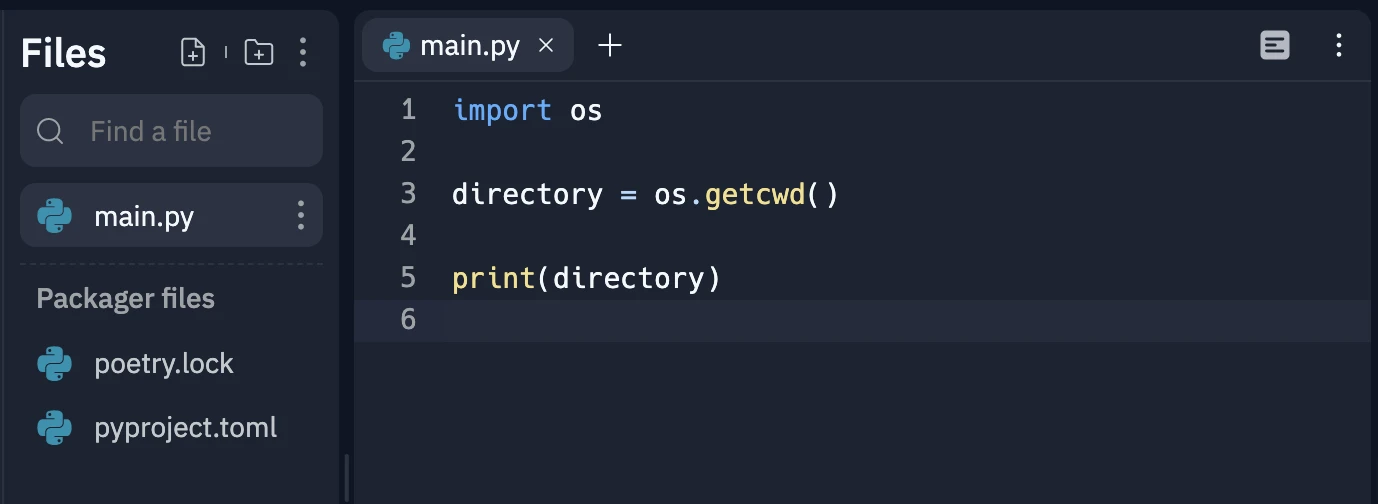
In the program above, we first import the “os” module in Python. Then we obtain the current working directory (cwd) using the getcwd() command and save it in the variable called “directory”. Note that the output of this command will be different in different environments. When we print the directory, we get the following output (your output might vary):
/home/runner/MedicalOrneryGame
Now, let us create a new file in our directory to illustrate the python os.remove() function. In the example below we have created a file called “example.txt”:
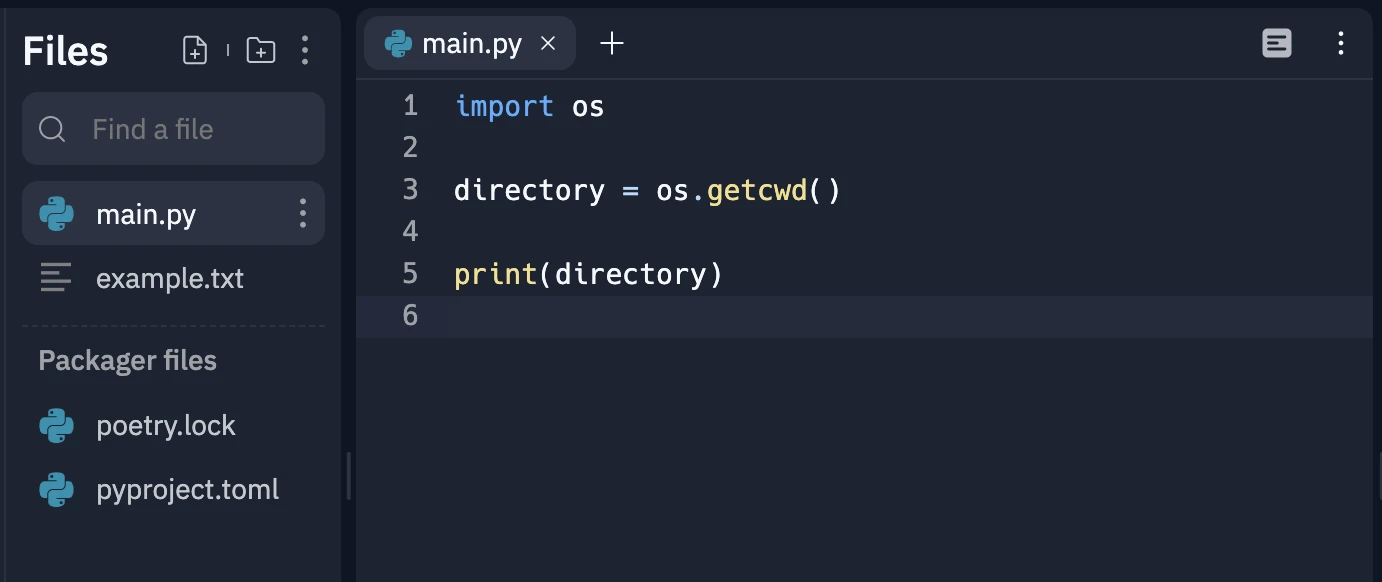
Now, let us update our program as follows:
import os
directory = os.getcwd()
print(directory)
os.remove('example.txt')
If we run this program, we will see that the example.txt file has been removed and your environment looks back to what it used to be:
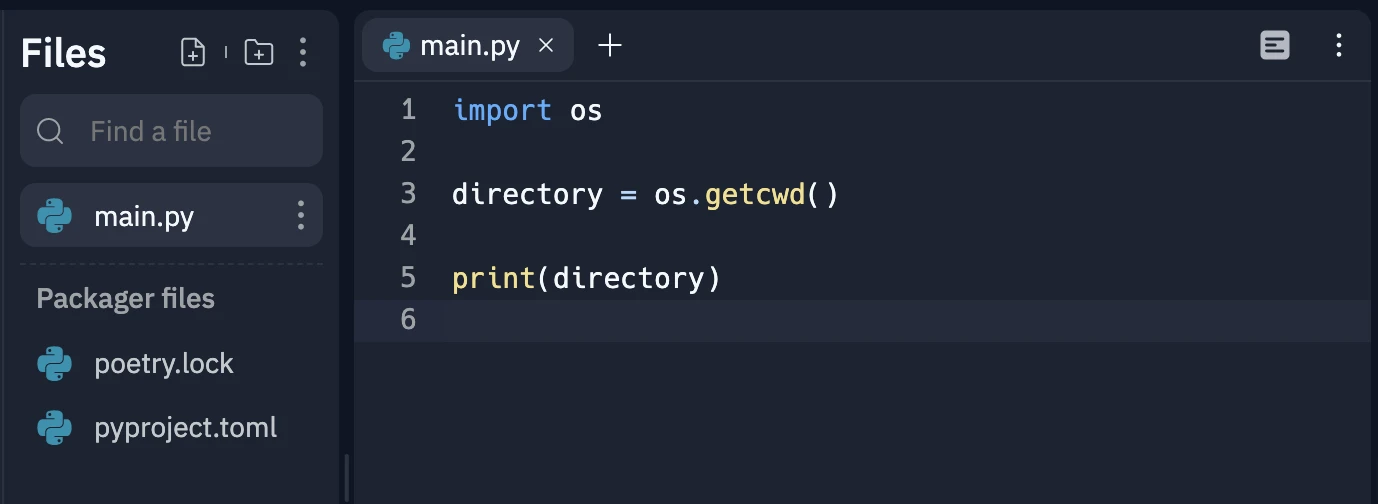
Note that the os.remove() function in our case worked without specifying a full path (i.e., you just had to give “example.txt” as input and not the full path name). This is because the current working directory (obtained by os.getcwd()) is also the directory where you have created the file. If the location of the file is different, like in the below example:
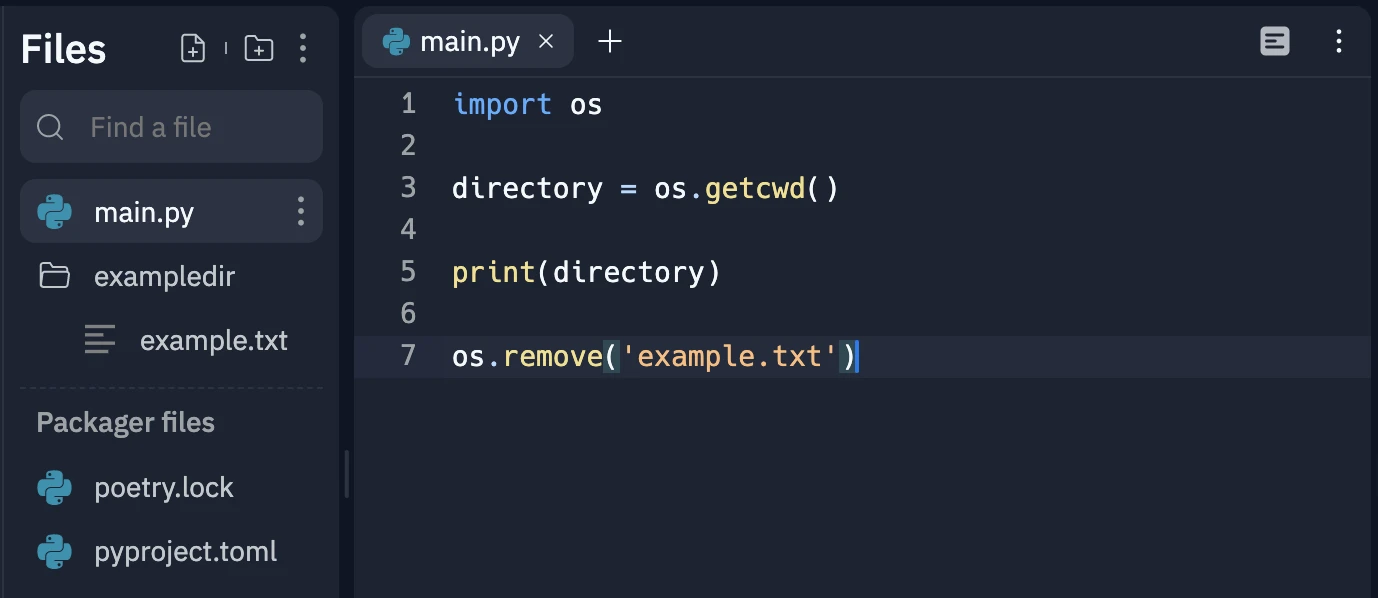
Here we have created a directory called “exampledir” and inside this directory there is a file called “example.txt”.
Now, let us run the below program again:
import os
directory = os.getcwd()
print(directory)
os.remove('example.txt')
We get the error:
/home/runner/MedicalOrneryGame
Traceback (most recent call last):
File "main.py", line 7, in <module>
os.remove('example.txt')
FileNotFoundError: [Errno 2] No such file or directory: 'example.txt'
This error happens because there is no file called “example.txt” in the current working directory (cwd). To remove this file, you need to prefix the filename with the path:
import os
directory = os.getcwd()
print(directory)
os.remove(directory + "example.txt")
We now get:
/home/runner/MedicalOrneryGame
Traceback (most recent call last):
File "main.py", line 7, in <module>
os.remove(directory + "example.txt")
FileNotFoundError: [Errno 2] No such file or directory:
'/home/runner/MedicalOrneryGameexample.txt'
Hmm.. We are still getting an error. Do you see what happened? We needed to add a slash (“/”) between the current working directory and the filename. Without the slash, the name of the file is considered to be “MedicalOrneryGameexample.txt” which is of course a non-existent file. Also we have made the mistake of omitting the directory “exampledir” because the example.txt file is inside the directory. Let us update the program taking into account all these issues:
import os
directory = os.getcwd()
print(directory)
os.remove(directory + "/" + "exampledir/example.txt")
Now the program works and the directory structure looks like:
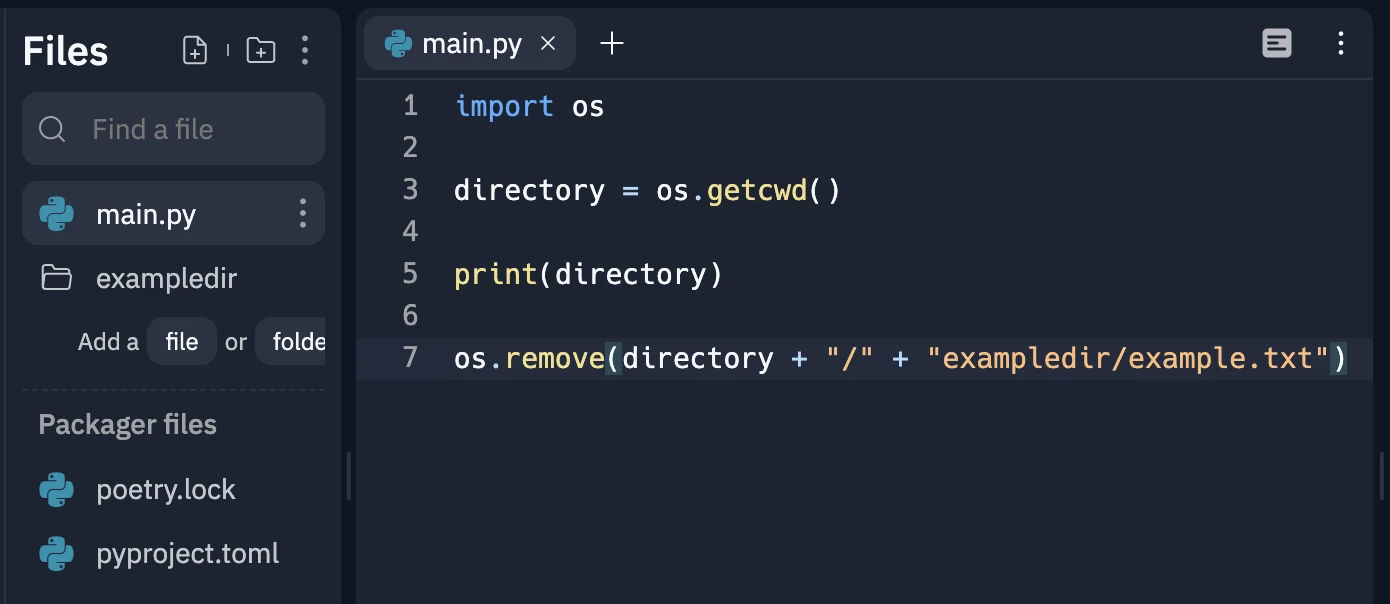
Let us now try to remove the “exampledir” directory from within Python. Let us update the program:
import os
directory = os.getcwd()
print(directory)
os.remove(directory)
The output is:
/home/runner/MedicalOrneryGame
Traceback (most recent call last):
File "main.py", line 7, in <module>
os.remove(directory)
IsADirectoryError: [Errno 21] Is a directory:
'/home/runner/MedicalOrneryGame'
As you can see, the os.remove() function works only with files (with a full path specified), not with directories. (Not to mention the fact that you are attempting to remove the directory in which you are currently situated.)
In this blogpost, we have seen how Python’s os.remove() function can help remove files from within your Python program. If you liked this, checkout our blogpost on the Python os.mkdir() function which as you can surmise helps create directories! Also checkout os.listdir() which lists the contents of a directory and os.chdir() which changes the current working directory!
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.