Kodeclik Blog
How to remove newlines from a Python string
In Python, newline characters (\n) often find their way into strings, whether through user input, file reading, or other sources. While newline characters are useful for formatting text, there are instances where you may need to remove them from a string. In this blog post, we'll explore four different methods to achieve this task, discussing their advantages and disadvantages along the way.
Method 1: Use the replace() method
The replace() function is a simple and straightforward method to remove newline characters from a string. It replaces all occurrences of a specified substring with another substring. In the below example code we replace all occurrences with a space.
string_with_newlines = "Kodeclik\\nOnline\\nAcademy"
string_without_newlines = string_with_newlines.replace("\\n", " ")
print(string_without_newlines)
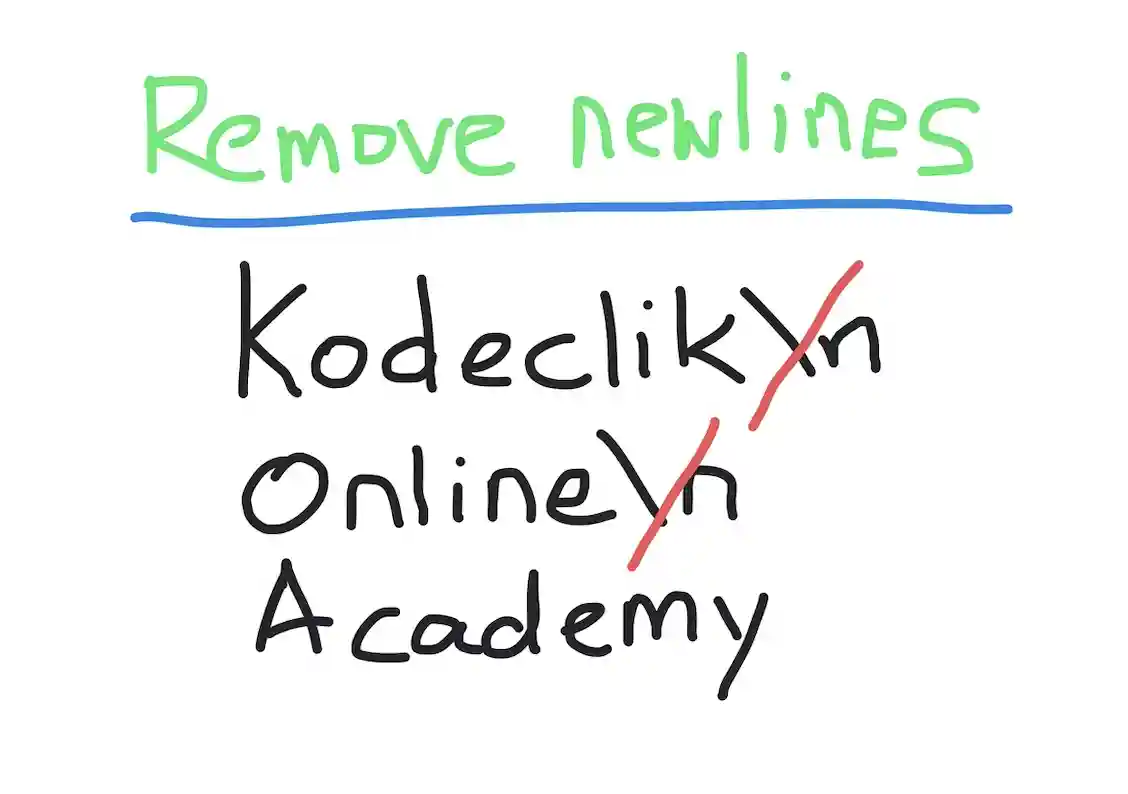
The output is:
Kodeclik Online Academy
Note that the original string had two newline characters which are now replaced with two space characters.
You can also use the replace() method to simply remove, by giving the empty string as a replacement string:
string_with_newlines = "Kodeclik\\nOnline\\nAcademy"
string_without_newlines = string_with_newlines.replace("\\n", "")
print(string_without_newlines)
The above code yields:
KodeclikOnlineAcademy
as expected.
The replace() method is easy to understand and implement. It only removes newline characters, leaving other characters intact. However, the method only removes newline characters and won't handle variations like multiple consecutive newline characters with different control codes, like “\r\n”.
Method 2: Use the regular expression module (re)
Regular expressions provide a powerful pattern matching and substitution capability. The re module in Python allows us to leverage regular expressions to remove newline characters.
import re
string_with_newlines = "Kodeclik\\nOnline\\nAcademy"
string_without_newlines = re.sub(r"\\n", "", string_with_newlines)
print(string_without_newlines)
The above code yields:
KodeclikOnlineAcademy
The above method is powerful, perhaps too powerful. Regular expressions provide advanced pattern matching, allowing you to handle different newline representations (\n, \r\n) or other variations. This also means that they can be challenging to grasp, especially for newcomers.
Method 3: Use the split() and join() functions
In this approach, we use Python's split() function to split the string into a list of substrings based on a specified delimiter (in our case, the newline). By splitting on newline characters and then joining the resulting list without newline characters, we can effectively remove them.
Here is example code that performs this task for us:
string_with_newlines = "Kodeclik\\nOnline\\nAcademy"
string_without_newlines = "".join(string_with_newlines.split("\\n"))
print(string_without_newlines)
The output is as expected:
KodeclikOnlineAcademy
The advantage of this approach is that the split() and join() functions can handle various delimiters, making it useful for other string manipulation tasks. However, this approach involves extra steps, namely splitting the string into a list and joining it again, which may impact performance for large strings. Also this approach only handles exact matches of newline characters and will not support different representations.
Method 4: Use splitlines()
Our final approach uses the Python splitlines() function which splits a string at line boundaries and returns a list of lines. By joining the lines without newline characters, we can effectively remove them. The below code is just a simple replacement of the previous approach:
string_with_newlines = "Kodeclik\\nOnline\\nAcademy"
string_without_newlines = "".join(string_with_newlines.splitlines())
print(string_without_newlines)
The output is again:
KodeclikOnlineAcademy
The advantage of this approach is that the splitlines() function is easy to understand and use. It handles different newline representations: It automatically recognizes and splits the string based on various newline representations, such as \n, \r\n, or \r. However, it only removes newline characters and won't handle other specific cases or variations.
In summary, removing newline characters from a string is a common requirement in Python. In this blog post, we explored four different methods. By understanding these methods, you can now confidently handle newline removal in your Python projects. Which approach is your favorite?
If you liked this blogpost checkout blogpost on doing the reverse, i.e., adding newlines, or line breaks. Also if you wish to remove more types of characters from a string, see our blogpost on how to remove punctuation from a Python string.
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.