Kodeclik Blog
Python string buffers
Strings are a very popular data structure in Python. One important characteristic of Python strings is their immutability. This means that once a string object is created, its contents cannot be changed. Any operation that appears to modify a string actually creates a new string object with the desired modifications and does not really modify the original string.
The immutability of strings brings several benefits. Firstly, it ensures the integrity of the original data, lest you accidentally modify it. Secondly, immutability allows strings to be used as keys in dictionaries or elements in sets, as their values cannot change. This enables efficient data retrieval and comparison operations, enhancing overall performance.
Consider the following code:
original_string = "Hello World!"
# Attempt to modify the string
modified_string = original_string.upper()
# Print the original and modified strings
print("Original string:", original_string)
print("Modified string:", modified_string)
In the code above, we start with an original_string variable containing the text "Hello World!". We then attempt to modify the string by calling the upper() method, which converts all characters in the string to uppercase. However, instead of modifying the original string, the upper() method creates a new string object with the desired modifications and assigns it to the modified_string variable.
The output is thus:
Original string: Hello World!
Modified string: HELLO WORLD!
Now that we have that out of the way, what is a string buffer?
What is a string buffer?
A string buffer in Python is an object that holds a sequence of characters, allowing us to manipulate and modify strings efficiently. It serves as a temporary storage space for building or modifying strings, without incurring the overhead of creating new string objects for every operation. String buffers are mutable and provide a convenient way to concatenate, modify, and manipulate strings in a memory-efficient manner.
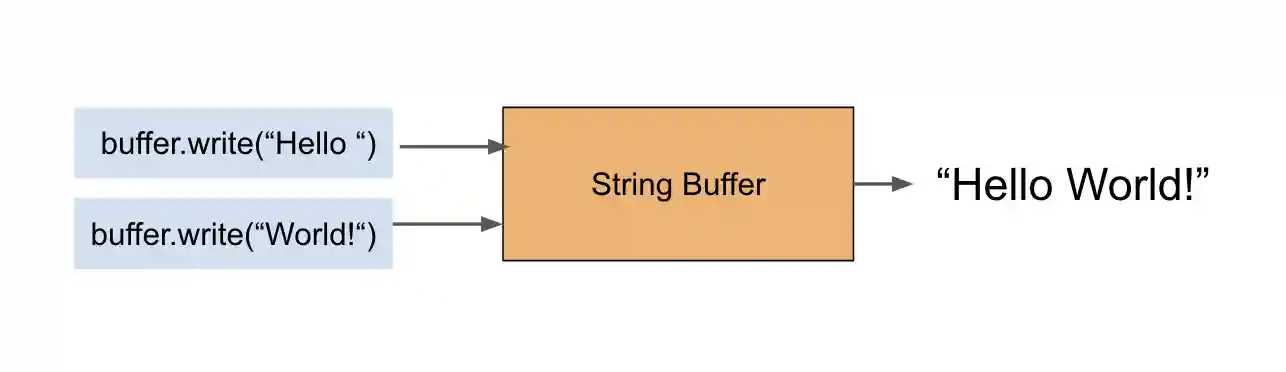
Creating a String Buffer
Python provides the io.StringIO module, which offers a convenient way to create a string buffer. Let's take a look at a simple example:
import io
buffer = io.StringIO()
buffer.write("Hello ")
buffer.write("World!")
result = buffer.getvalue()
print(result)
The output is:
Hello World!
Note that in the example above, we import the io module and create an instance of the StringIO class. We then use the write() method to append strings to the buffer. During this process the buffer keeps getting updated, unlike the string example earlier. Finally, we retrieve the contents of the buffer using the getvalue() method.
Since string buffers are mutable, they avoid the memory overhead associated with creating new string objects. This is particularly useful when dealing with large strings or in scenarios where your computer does not have much memory.
By leveraging string buffers, we can optimize memory usage, enhance performance, and simplify complex string manipulation tasks.
If you liked this blogpost, learn about Python string truncation which shortens strings to desired limits.
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.