Kodeclik Blog
Python vars()
Python vars() is a function that is used to inspect objects and understand the dictionary behind the object. The dictionary behind an object is stored in a variable called “__dict__”. It stores the attributes of the object along with their values.
Python vars() with an object
Let us create a Python class called Book denoting objects with attributes called name, author, year (of publication), and publisher.
class Book:
def __init__(self, name, author, year, pub):
self.name = name
self.author = author
self.year = year
self.pub = pub
The above class (Book) has a constructor that creates an object with the given arguments, i.e., name of the book, author of the book, year of publication, and the publisher.
We can create objects of the above class with statements like this:
book1 = Book("Harry Potter and the Philosopher's Stone","J.K. Rowling",
1997,"Bloomsbury")
This statement creates a very specific book with the values given in the argument list.
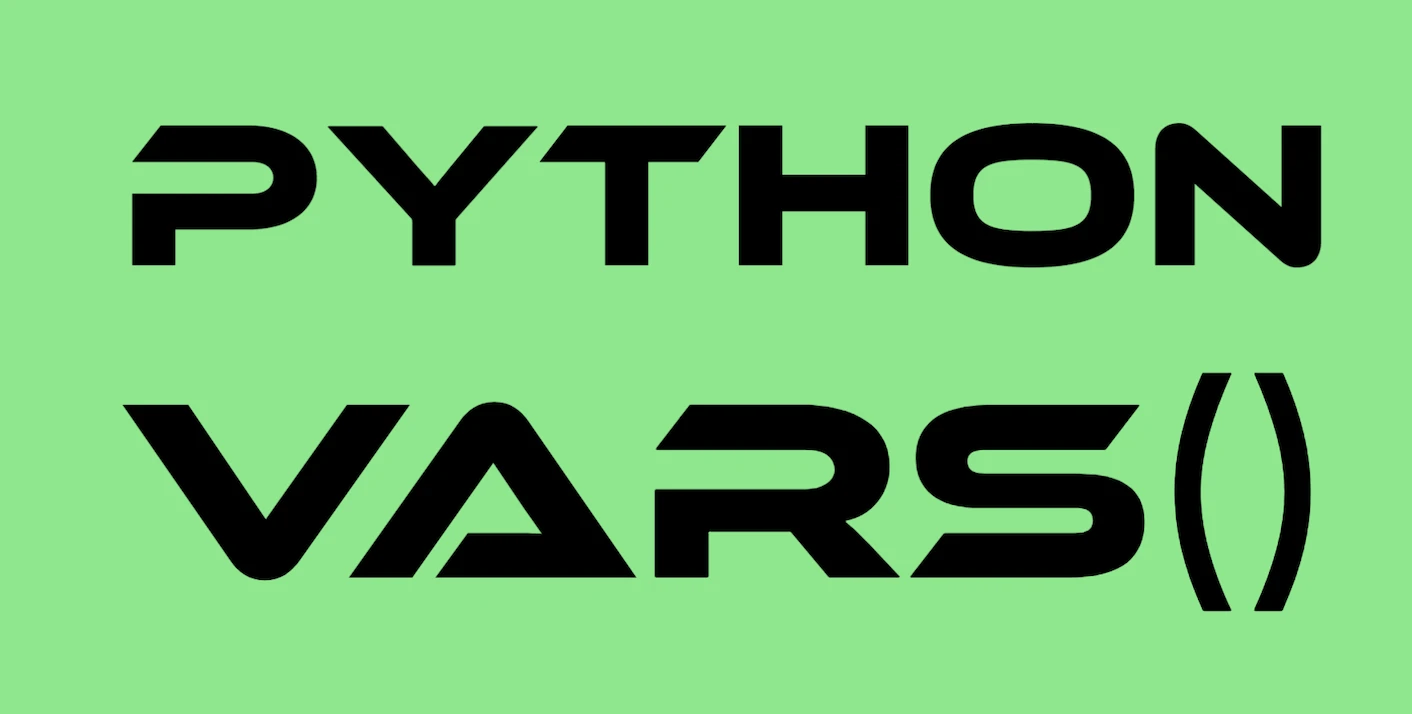
The vars() function is something you can use with an object (in this case, book1) and inspect the inner workings of the object. In our case, these specific values are often stored in a dictionary and the vars() function outputs the dictionary. Thus, if we do:
print(vars(book1))
The output is:
{'name': "Harry Potter and the Philosopher's Stone",
'author': 'J.K. Rowling',
'year': 1997,
'pub': 'Bloomsbury'}
As you can see, the keys of the dictionary are ‘name’, ‘author’, ‘year’, and ‘pub’. The values are ‘Harry Potter and the Philosopher’s Stone’, ‘J.K. Rowling’, 1997, and ‘Bloomsbury’. This dictionary is stored in a pre-defined variable called “__dict__”, so you could have printed the dictionary using:
print(book1.__dict__)
The output is again:
{'name': "Harry Potter and the Philosopher's Stone",
'author': 'J.K. Rowling',
'year': 1997,
'pub': 'Bloomsbury'}
The vars() function is useful for inspecting objects to see what attributes and values they have. For instance you can use this to dynamically generate HTML or other text based on the attributes and values of an object.
Python vars() with a class
We can apply the vars() function to a class rather than an instance of a class:
class Book:
def __init__(self, name, author, year, pub):
self.name = name
self.author = author
self.year = year
self.pub = pub
print(vars(Book))
The output is:
{'__module__': '__main__',
'__init__': <function Book.__init__ at 0x7fe85bf85af0>,
'__dict__': <attribute '__dict__' of 'Book' objects>,
'__weakref__': <attribute '__weakref__' of 'Book' objects>,
'__doc__': None}
Note that the vars() function outputs a dictionary (but most of this output appears gibberish because this is a dictionary used internally by Python to store the details of the Book object.) The main takeaway here is that vars() can be applied to both objects and classes.
Python vars() with a dictionary
Let us create an actual dictionary that stores months and the number of days in each.
calendar = {'January': 31,
'February': 28,
'March': 31,
'April': 30,
'May': 31,
'June': 30,
'July': 31,
'August': 31,
'September': 30,
'October': 31,
'November': 30,
'December': 31}
And now let us try vars() with this dictionary:
print(vars(calendar))
The output is:
Traceback (most recent call last):
File "main.py", line 14, in <module>
print(vars(calendar))
TypeError: vars() argument must have __dict__ attribute
This is very interesting! So an actual dictionary does not have a “__dict__” attribute! Why is this? Let us replace that line with:
print(calendar.__dict__)
The output is:
Traceback (most recent call last):
File "main.py", line 14, in <module>
print(calendar.__dict__)
AttributeError: 'dict' object has no attribute '__dict__'
So, true enough, this object does not have a “__dict__” attribute. This is because Python does not quite store the details of this object in an internal __dict__ structure and thus there is no internal __dict__ object to return.
Python vars() with a list
Let us try vars() with a Python list.
list = [1,2,3,'hello']
print(vars(list))
As expected, the output is:
Traceback (most recent call last):
File "main.py", line 2, in <module>
print(vars(list))
TypeError: vars() argument must have __dict__ attribute
Python vars() with an integer
If we try vars() with an integer:
num = 234
print(vars(num))
The output will be:
Traceback (most recent call last):
File "main.py", line 2, in <module>
print(vars(num))
TypeError: vars() argument must have __dict__ attribute
So in short, vars() is a function that takes a single argument, which can be an object, class, or module. The returned dictionary contains the object's variables and their values.
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.