Kodeclik Blog
How to reverse a range in Python
The range() operator is very common in Python and you will usually see it in for loops, like so:
for i in range(1,10):
print(i)
The output is:
1
2
3
4
5
6
7
8
9
The range operator essentially says we begin the variable “i” indexed at 1, and we keep incrementing it (by 1) until we exceed 10. To make the increment size explicit, we can do:
for i in range(1,10,1):
print(i)
The output is the same as before:
1
2
3
4
5
6
7
8
9
We can make the stepsize to be more than 1, such as 2:
for i in range(1,10,2):
print(i)
The output will be:
1
3
5
7
9
As can be seen, the range operator above takes three arguments. It starts at 1 (the first argument) and increments it by 2 (the third argument). It stops when it exceeds 10 (the second argument). In fact, the range operator really returns a list, so you could try to do:
print(range(1,10,2))
This yields:
range(1, 10, 2)
which is not quite informative. To see the elements in the range, you should do:
print(list(range(1,10,2)))
This will give the output we are looking for:
[1, 3, 5, 7, 9]
In Python, therefore, the range() function is a helpful tool for producing numerical sequences. It's frequently used in for loops as we show above, to iterate over a set number of items. However, sometimes you may need to reverse the order of the sequence. This blogpost shows you two ways to achieve this purpose.
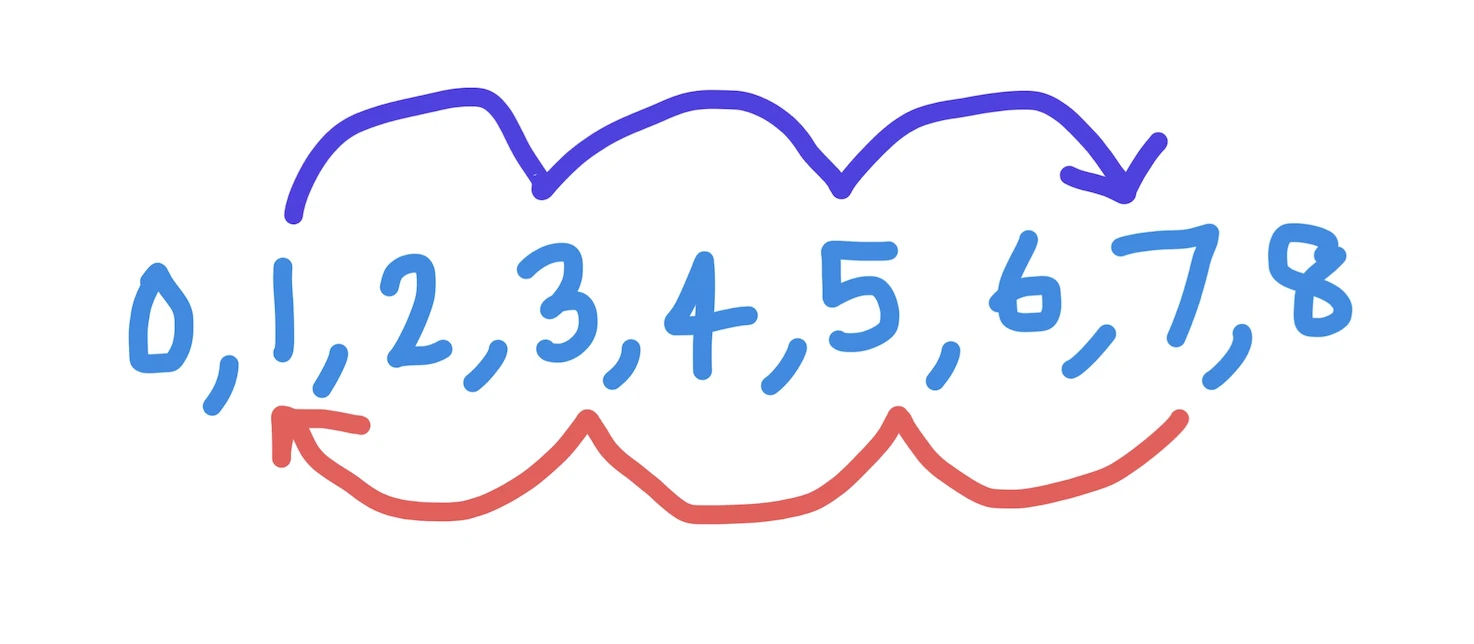
Reversing a range using a negative step size
The easiest way to count down rather than count up is to use a negative step, like so:
print(list(range(10,1,-1)))
The output will be:
[10, 9, 8, 7, 6, 5, 4, 3, 2]
Note that the range will stop before it reaches 1 (i.e., the second argument is considered to be outside the range).
Let us try a negative step size but higher, like:
print(list(range(7,0,-2)))
The output will be:
[7, 5, 3, 1]
as expected.
Reversing a range using the reversed() function
A different way to reverse a range is to write the range operation as if it is incrementing and then use the reversed() function:
print(list(reversed(range(3,10,3))))
Here, the range goes from 3 in steps of 3, so it yields values of 3, then 6, then 9. The reversed() function flips the order, so the above statement prints:
[9, 6, 3]
This is thus another way to reverse a range. But note that the actual results returned by the reversed() function will not be quite the same as if you had simply flipped the start and ending values and negated the step size.
So if we do:
print(list(range(10,3,-3)))
The output is:
[10, 7, 4]
This is because in the first example we started at 3 (before reversing) and we will never reach 10 going in steps of 3. In the second example, we started at 10 and we will never reach 3 going in steps of -3. You have to take this into account when you use reversed() as an option for reversing a range.
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.