Kodeclik Blog
How to add days to dates in Javascript
There are numerous ways Javascript offers to add a specified number of days to the current date in Javascript. In each of these ways you first need to retrieve the current date and then add the desired number of days to that date. There are specific methods that we will study next.
Method 1: Use the getDate() and setDate() methods
In our first approach, we define a function addDays() that takes a Date object and the number of days to add as arguments. The function then calls the getDate() method to get the current date, adds the number of days to it, and calls the setDate() method to set the new day of the month for the date. Finally, the function returns the modified Date object.
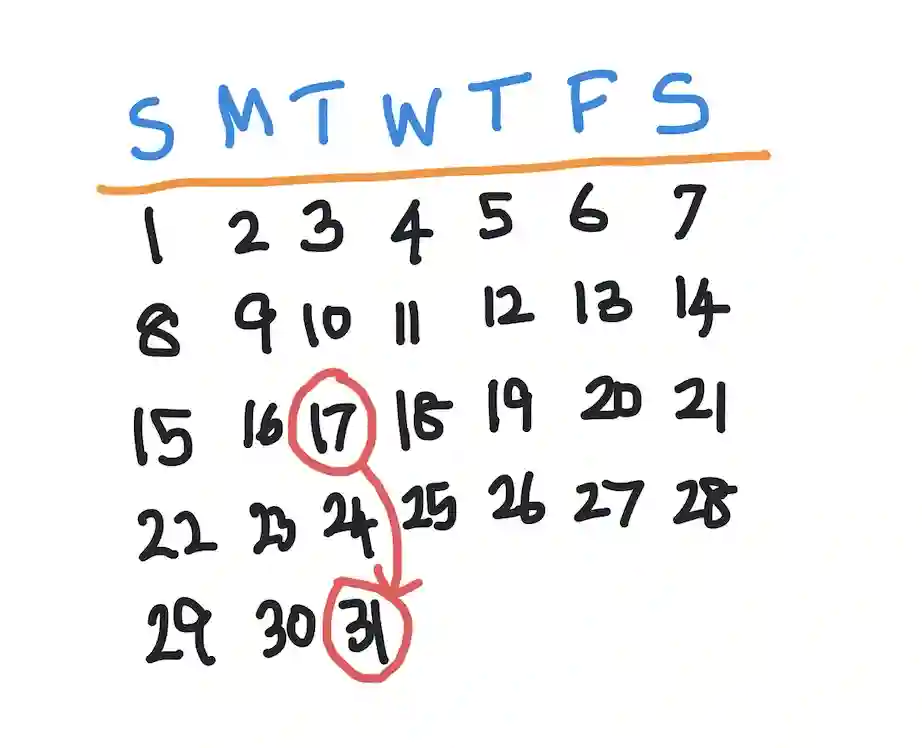
function addDays(date, days) {
date.setDate(date.getDate() + days);
return date;
}
const date = new Date('2023-10-17 00:00:00');
const newDate = addDays(date, 14);
console.log(newDate);
In this example, we define a function addDays() that takes a Date object and the number of days to add as arguments. The function then calls the getDate() method, adds the number of days to it, and calls the setDate() method to set the new date. Finally, the function returns the modified Date object.
There are a few things to note here. It is often important to specify the time when creating the date object (as we have done above). This is because depending on the time zone in which your program is running the results might be off by a day. So it is good to be explicit about the time. Second, note that we are adding 14 days, a multiple of 7. Thus the new date will fall on the same day of the week as the original date. The original date is Oct 17, 2023, a Tuesday. Thus the final answer will also be a Tuesday. If we run this program and inspect the console, we will get:
Tue Oct 31 2023 00:00:00 GMT-0400 (Eastern Daylight Time)
(your results might indicate a different time zone but you should still see the offset lead to Oct 31, 2023).
Method 2: Use the getTime() and setTime() methods
In this approach, we define a function addDays() that takes a Date object and the number of days to add as arguments, as before. The function then calls the getTime() method to get the number of milliseconds since January 1, 1970, adds the number of days converted to milliseconds to it, and then uses setTime() to apply the modified time value.
function addDays(date, days) {
const oldtime = date.getTime();
const newtime = oldtime + (days * 24 * 60 * 60 * 1000);
date.setTime(newtime);
return date;
}
const date = new Date('2023-10-17 EDT');
const newDate = addDays(date, 14);
console.log(newDate);
Outside the function we add two weeks to Oct 17, 2023 as before and we will get this output in the console. Once again the output should fall on the same day of the week as Oct 17, 2023, ie a Tuesday. Note also that we explicitly gave the input as EDT and obtained the result as EDT, again as before:
Tue Oct 31 2023 00:00:00 GMT-0400 (Eastern Daylight Time)
Method 3: Use the setUTCDate and getUTCDate() methods
In our third approach, we once again define a function addDays() that takes a Date object and the number of days to add as arguments. The function then calls the getUTCDate() method, adds the number of days to its output, and calls the setUTCDate() method to set the new date. Finally, the function returns the modified Date object.
function addDays(date, days) {
date.setUTCDate(date.getUTCDate() + days);
return date;
}
const date = new Date('2023-10-17 00:00:00');
const newDate = addDays(date, 7);
console.log(newDate);
The output is:
Tue Oct 24 2023 00:00:00 GMT-0400 (Eastern Daylight Time)
as expected.
These are just a few examples of how to add days to a date in JavaScript. There are many other ways to do it, depending on your specific use case and requirements. To understand these other options, take a closer look at the above methods for the Date() class and the options in each of them.
There are many scenarios to use the programs above. Here are three practical examples of Javascript programs where we might need to add days to dates:
First, consider booking systems such as used in hotels or dormitories. In a booking system, we might need to add a certain number of days to a given date to calculate the check-out date for a reservation. For example, if a customer books a hotel room for 3 nights starting on October 17, 2023, we would need to add 3 days to the start date to calculate the check-out date, which would be October 20, 2023.
Second, consider scheduling systems used for project management in a company. In a scheduling system, we might need to add a certain number of days to a given date to calculate the due date for a task or project. For example, if a task is due in 7 days starting on October 17, 2023, we would need to add 7 days to the start date to calculate the due date, which would be October 24, 2023.
Finally, consider countdown timers. In a countdown timer, we might need to add a certain number of days to a given date to calculate the end date for the timer. For example, if we want to create a countdown timer that counts down from 30 days starting on October 17, 2023, we would need to add 30 days to the start date to calculate the end date, which would be November 16, 2023.
These are just a few examples of Javascript programs where we might need to add days to dates. There are many other use cases where date manipulation is required, such as financial applications, event planning, and more.
If you liked this blogpost, learn about how to add days to dates in Python! Also see how to pretty print dates in Javascript using the toISOString() method!
Want to learn Javascript with us? Sign up for 1:1 or small group classes.