Kodeclik Blog
How to round down a number in Javascript
The Math.floor() method is a handy way to round down a number in Javascript. This method always rounds down and returns the largest integer less than or equal to a given number:
let num = 3.7;
let roundedDown = Math.floor(num);
console.log(roundedDown); // Output: 3
In the above code, we are using Math.foor() on the operand 3.7. The largest integer less than or equal to 3.7 is 3 and thus the roundedDown variable contains 3.
A situation where you might use Math.floor() is when you need to calculate the number of whole units of a certain item you can buy with a given amount of money. For example, if you have $20 and each item costs $3.50, you can use Math.floor() to calculate the maximum number of items you can buy:
let budget = 20;
let itemCost = 3.50;
let maxItems = Math.floor(budget / itemCost);
console.log(maxItems); // Output: 5
In this case, Math.floor() is used to ensure that the result is a whole number, since you can't buy a fraction of an item.
Note that Math.floor() rounds down to the nearest integer, regardless of whether the input is an integer or a floating-point number. When using Math.floor() with floating-point numbers, it is important to keep in mind that floating-point arithmetic can sometimes lead to rounding errors.
For example, Math.floor(1.9999999999999999) would return 1, rather than 2, due to the imprecision of floating-point arithmetic. However, for most practical purposes, Math.floor() can be used with floating-point numbers to round down to the nearest integer.
Also note that Math.floor() always rounds down to the nearest integer, even if the decimal part of the number is 0.5 or greater. In other words, it does not round up.
Rounding down negative numbers
Here are some examples of using Math.floor() with negative floating point numbers:
let num1 = -3.7;
let num2 = -2.1;
let roundedDown1 = Math.floor(num1);
let roundedDown2 = Math.floor(num2);
console.log(roundedDown1); // Output: -4
console.log(roundedDown2); // Output: -3
In both cases, Math.floor() rounds down to the nearest integer, which is a smaller negative number.
For more discussion on rounding down, see our detailed blogpost on Math.floor() in Javascript.
Rounding down to different levels in Javascript
Let us attempt to write a program to round down to different levels, e.g., rounding down to the nearest ten, or the nearest hundred, rather than just the nearest integer. For this purpose, we can write a function that takes two arguments: the number you want to round down and the level of rounding down needed. Here is an example of such a function:
function roundDown(num, level) {
let factor = Math.pow(10, level);
return (Math.floor(num/factor) * factor);
}
In this function, the num parameter is the number you want to round down, and the level parameter is the level of rounding down needed. The factor variable is calculated as 10 to the power of the level parameter, which determines the decimal place to which the number should be rounded down. We then divide by the factor to move the decimal point suitably, use Math.floor() on this value, and then multiply the factor again to get the desired final rounded down value.
Below are some trials with it:
let num = 387.4;
console.log(roundDown(num,0))
console.log(roundDown(num,1))
console.log(roundDown(num,2))
console.log(roundDown(num,3))
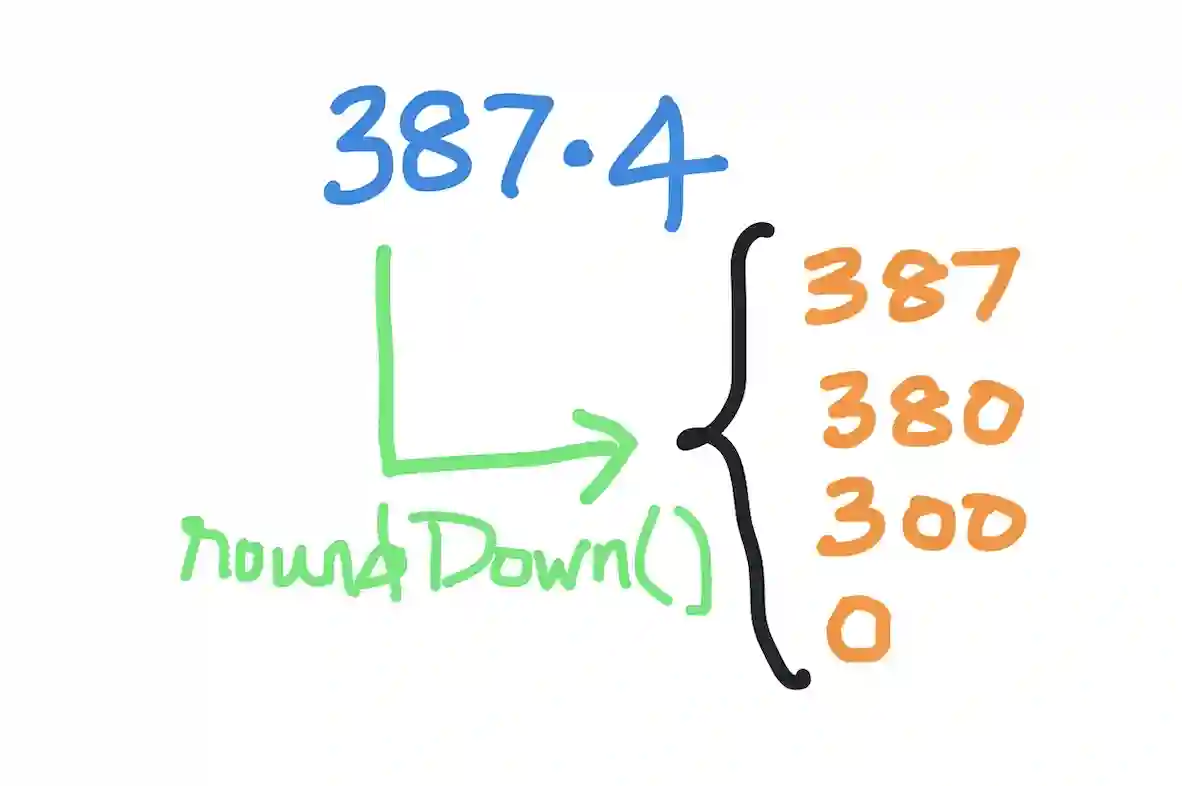
The output will be:
387
380
300
0
In other words, the nearest integer (rounded down) is 387, nearest ten is 380, nearest hundred is 300 (remember we are still rounding down), and the nearest thousand is 0 (i.e., 0 thousands).
There are many situations where you will find this useful.
Below are four examples of where you can use the roundDown() function to round down to different levels:
Financial calculations: When working with financial data, it is often necessary to round down to a specific decimal place. For example, you might need to round down to the nearest penny when calculating sales tax or rounding down to the nearest dollar when calculating a budget.
Grade calculations: When calculating grades for students, it is common to round down to the nearest whole number or decimal place. For example, you might need to round down to the nearest tenth when calculating a student's grade point average.
Real-world measurements: When working with real-world measurements, it is often necessary to round down to a specific decimal place. For example, you might need to round down to the nearest inch when measuring the length of a piece of wood or rounding down to the nearest milliliter when measuring a liquid.
Situational interview questions: In a job interview, you might be asked a situational question that requires you to round down to a specific decimal place. For example, you might be asked to calculate the cost of a product that is priced at $4.99 and is on sale for 20% off, rounded down to the nearest penny.
In each of the above examples, the roundDown() function can be used to round down to the appropriate decimal place, making calculations more accurate and precise.
Want to learn Javascript with us? Sign up for 1:1 or small group classes.