Kodeclik Blog
How to use numpy.roll() in Python
numpy.roll() is a function in the Python numpy library and allows you to roll (rotate) an array’s elements along a given axis.
First, let's define what we mean by "rolling" an array. When we roll an array, we're essentially shifting its elements along a particular axis. This can be useful in a variety of scenarios, such as circularly shifting the elements of an array or reordering them in a specific way.
The numpy.roll() function provides a convenient way to perform these operations. Its syntax is simple: numpy.roll(arr, shift, axis=None). Here, arr is the array we want to roll, shift is the number of places by which we want to shift the elements, and axis specifies the axis along which we want to roll the array. If axis is not specified, all dimensions of the array will be rolled.
Let us try it out!
import numpy as np
temperatures = [85.6, 75.2, 81.5, 75.4, 81.3]
print(temperatures)
print(np.roll(temperatures,2))
Here we have a Python list (called temperatures) and we are rolling this list (by 2 positions to the right) which will return an array. Because we are rolling by 2 positions, the 2 values that we push out of the list (array) will get added to the front of the array. So the output will be:
[85.6, 75.2, 81.5, 75.4, 81.3]
[75.4 81.3 85.6 75.2 81.5]
Note that 75.4 and 81.3 are now in the beginning of the numpy array.
You can also try rolling in the opposite direction by giving a negative offset:
import numpy as np
temperatures = [85.6, 75.2, 81.5, 75.4, 81.3]
print(temperatures)
print(np.roll(temperatures,-2))
The output is as expected:
[85.6, 75.2, 81.5, 75.4, 81.3]
[81.5 75.4 81.3 85.6 75.2]
Let us now try rolling a two dimensional array!
import numpy as np
magic_square = np.array([[8, 1, 6], [3, 5, 7], [4, 9, 2]])
print(np.roll(magic_square, 1, axis=0))
Here we are creating an array (denoting a magic square of numbers). The axis=0 specifies that we are only rolling the rows. Thus, the rows of the array have been shifted by one place, with the last row being moved to the beginning of the array. The output is:
[[4 9 2]
[8 1 6]
[3 5 7]]
Let us now try rolling only the columns!
import numpy as np
magic_square = np.array([[8, 1, 6], [3, 5, 7], [4, 9, 2]])
print(np.roll(magic_square, 1, axis=1))
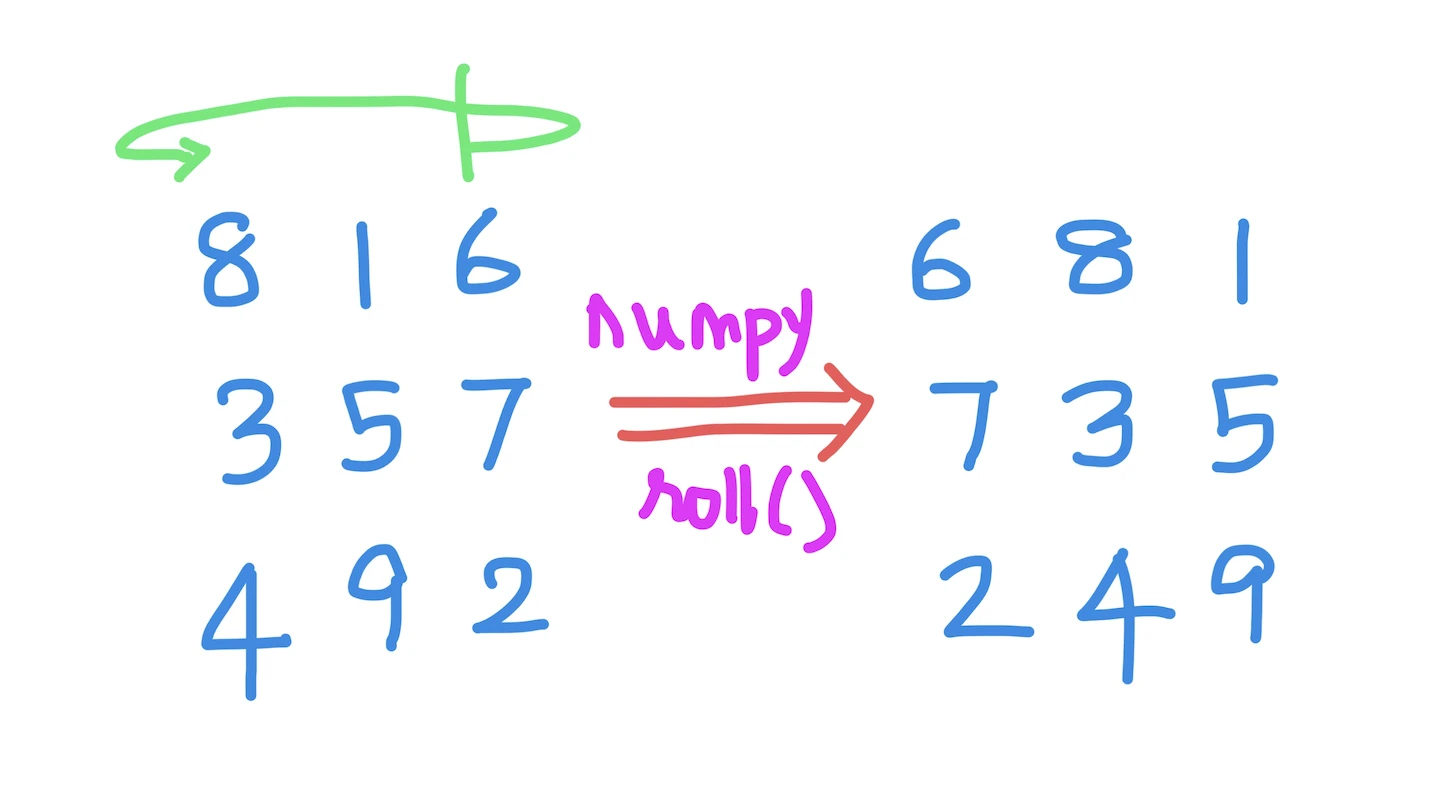
The output is:
[[6 8 1]
[7 3 5]
[2 4 9]]
Note that if you roll with an offset equal to the size of the dimension, you will obtain the same array back. Thus if we change the above program as:
import numpy as np
magic_square = np.array([[8, 1, 6], [3, 5, 7], [4, 9, 2]])
print(np.roll(magic_square, 3, axis=1))
it will yield:
[[8 1 6]
[3 5 7]
[4 9 2]]
This is because rolling along the column dimension 3 times gives the same array back.
Finally, you can roll along both axes, with specific offsets, like so:
import numpy as np
magic_square = np.array([[8, 1, 6], [3, 5, 7], [4, 9, 2]])
print(np.roll(magic_square, (1,3), axis=(0,1)))
Here we are rolling along both dimensions. Along the rows (ie axis=0) we are rolling by 1. Along the columns (i.e., axis=1) we are rolling by 3 (so they will be unchanged). The output is:
[[4 9 2]
[8 1 6]
[3 5 7]]
i.e., what we obtained in our first program earlier.
These are just a few examples of how numpy.roll() can be used to manipulate array elements in Python. With this powerful tool at your disposal, you can easily perform a variety of data manipulation tasks, such as circularly shifting an array or reordering its elements in a specific way.
In conclusion, numpy.roll() is a must-have tool for anyone working with arrays in Python. Its versatility and ease of use make it an indispensable part of the numpy library, and its powerful functionality can help you accomplish a wide variety of data manipulation tasks with ease.
If you liked this blogpost, learn how numpy.clip() works!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.