Kodeclik Blog
The Python __call__ method
Let us consider a simple class to store information about restaurants:
class Restaurant:
def __init__(self, name, address):
self.name = name
self.address = address
Note that we have defined the __init__ method for this class that denotes what needs to happen when we create new objects of the Restaurant class. In this case, the __init__ method takes two arguments (name and address) and these arguments are used to assign respective attributes of the Restaurant object.
We can instantiate a new Restaurant object as follows:
my_favorite_restaurant = Restaurant("Corner Cafe","123 Main Street")
Let us expand our class definition to also include a review component:
class Restaurant:
def __init__(self, name, address, review):
self.name = name
self.address = address
self.review = review
Let us agree for the review to be a list of strings, each string denoting one review. We must also correspondingly update our Restaurant object. In the below, we initialize the object to have no reviews at the outset.
my_favorite_restaurant = Restaurant("Corner Cafe","123 Main Street",[])
Let us now add a add_review method.
def add_review(self,review):
self.review.append(review)
print(self.review)
Now if we add the following two lines:
my_favorite_restaurant.add_review("Great!")
my_favorite_restaurant.add_review("It was OK")
We will get the following lines as output:
['Great!']
['Great!', 'It was OK']
Using the Python __call__ method
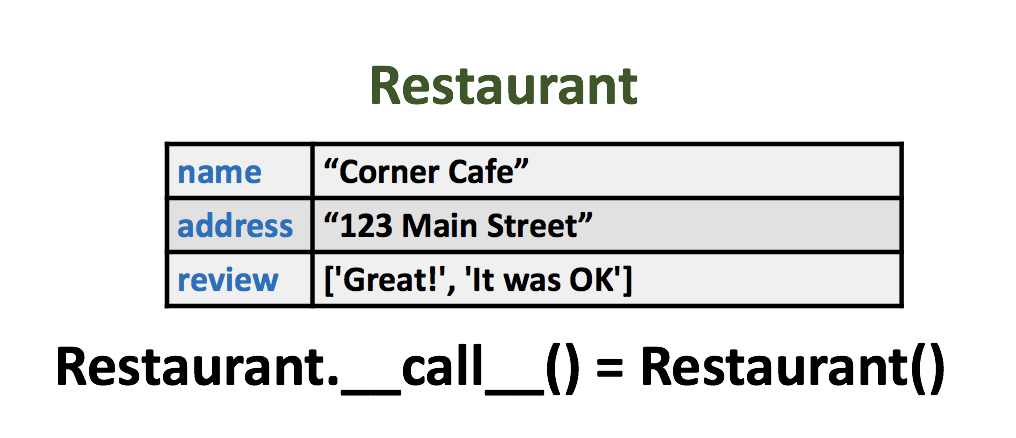
The __call__ method essentially allows you to use objects as functions. To see how, we will update the class definition as follows:
class Restaurant:
def __init__(self, name, address, review):
self.name = name
self.address = address
self.review = review
def __call__(self,review):
self.review.append(review)
print(self.review)
Note that the contents of the __call__ method is exactly the same as the add_review method. However the way we invoke this method, i.e., the way we use it, is syntactically much simpler:
my_favorite_restaurant("Great!")
my_favorite_restaurant("It was OK")
We are essentially using the object (not the class) as a function and passing the review string to it as its argument. The output is still the same:
['Great!']
['Great!', 'It was OK']
So what have we learnt? If you have a __call__ method defined using our class, you can invoke the method using either the syntax “object.__call()” or “object()”.
Now, obviously this idea of calling the object as a function will work only if a corresponding __call__ method is defined inside the class definition. You can verify if this will work by using the “callable()” predicate:
if (callable(my_favorite_restaurant)):
print("I can call this as a function!")
This will output:
I can call this as a function!
Try removing the __call__ function and now callable() will return False. In fact, if you attempt to use it as a callable, you will get the error:
Traceback (most recent call last):
File "main.py", line 11, in <module>
my_favorite_restaurant("Great!")
TypeError: 'Restaurant' object is not callable
The __call__ method is very useful when we are creating APIs and would like to use object oriented programming style in the creation and invocation of the API functions. Note that the arguments to the __call__ method can be anything you would like, so a lot of domain-specific functionality could be embedded inside this method.
If you liked learning about the __call__ method, checkout the Python hasattr() function. Also for more about
Python's object-oriented programming features, see our blogpost on private methods in Python.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.