Kodeclik Blog
How to capitalize the first letters of words in a string
Haven’t you found it necessary to sometimes take a string and capitalize the first letter of each word? For instance, suppose you have a list of names such as:
names = ['harry','Harry potter','mickey Mouse','donald duck']
print(names)
This yields as output:
['harry', 'Harry potter', 'mickey Mouse', 'donald duck']
Note how inconsistent the names are. We will now explore 5 different ways to standardize the capitalization in these strings.
Method 1: The str.capitalize() method
First, we will explore a handy method called capitalize() that applies to strings.
names = ['harry','Harry potter','mickey Mouse','donald duck']
print(names[0].capitalize())
print(names[1].capitalize())
print(names[2].capitalize())
print(names[3].capitalize())
The output is:
Harry
Harry potter
Mickey mouse
Donald duck
Note how capitalize() capitalizes the first letter of the first word (and only that). Other words are left intact in the string. To apply the capitalize() function uniformly on all characters of the list, we can use the map() function.
Here is one way to apply the map function:
names = ['harry','Harry potter','mickey Mouse','donald duck']
print(map(str.capitalize,names))
The output is (your specific address might vary):
<map object at 0x7f3fea684ca0>
This returns the mapped output object which is computed lazily. See our Python map() function blogpost for more details. To print the output, you can use the list() constructor.
names = ['harry','Harry potter','mickey Mouse','donald duck']
print(list(map(str.capitalize,names)))
The output is:
['Harry', 'Harry potter', 'Mickey mouse', 'Donald duck']
Method 2: Using str.title() to capitalize the first letter of each word in a string
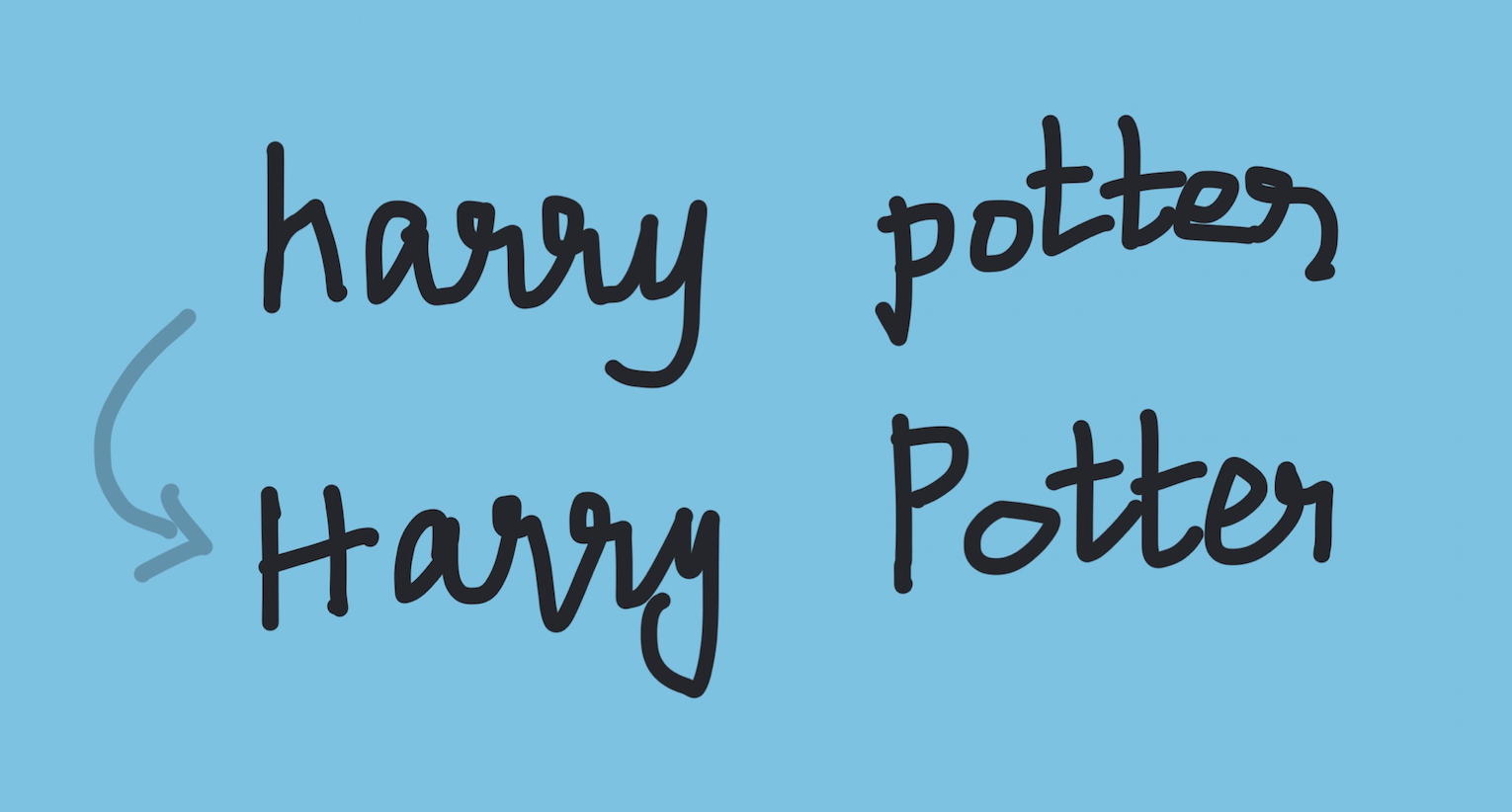
To capitalize the first letter of each word in a string, not just the first, use the str.title() method. For instance, consider the following program:
names = ['harry','Harry potter','mickey Mouse','donald duck']
print(list(map(str.title,names)))
The output is:
['Harry', 'Harry Potter', 'Mickey Mouse', 'Donald Duck']
The str.title() method does not only capitalize the first letter of each word. It also renders the other letters in each word to be in lower case. Consider:
names = ['harry','HARRY potter','mickey Mouse','donald duck']
print(list(map(str.title,names)))
The output will be:
['Harry', 'Harry Potter', 'Mickey Mouse', 'Donald Duck']
Note how in the second element of the list, “HARRY potter” gets converted to “Harry Potter”.
Method 3: Using string.capwords() to capitalize the first letter of each word in a string
The method capwords() is available as part of the string module in Python. Thus, to use this method, you need to first import the string module.
import string
names = ['harry','HARRY potter','mickey Mouse','donald duck']
print(list(map(string.capwords,names)))
The output is:
['Harry', 'Harry Potter', 'Mickey Mouse', 'Donald Duck']
Thus the output is exactly similar to the str.title() method.
What is the difference between string.capwords() and str.title()?
One key difference between these two methods is str.title() treats an apostrophe as the beginning of a new word (and thus capitalizes the beginning of that word) whereas string.capwords() does not. Consider:
import string
myname = "Kodeclik's Online Academy"
print(string.capwords(myname))
print(str.title(myname))
The output is:
Kodeclik's Online Academy
Kodeclik'S Online Academy
Method 4: Using a list comprehension to capitalize the first letter of each word in a string
We can go back to basics and use the str.capitalize() and apply it to each word in a string by first breaking down the string into individual words and then joining the results back. Here is a program that uses this approach:
def mycapitalize(phrase):
return ' '.join(w.capitalize() for w in phrase.split(' '))
print(mycapitalize("Kodeclik online academy"))
This will give the output:
Kodeclik Online Academy
As the above code shows, the function mycapitalize first splits the input phrase into words and for each word (w), capitalizes the first letter and then joins these capitalized words back using the join() function.
Method 5: Use string slicing operators to capitalize first letter of each word in a string
The final approach we will use is a small variant of the previous. We will use string slicing operators to convert the first character of the word to uppercase using the upper() method and then use the same split and join mechanism as before.
Here is a program that uses this technique:
def mycapitalize(phrase):
return ' '.join(w[0].upper() + w[1:] for w in phrase.split(' '))
print(mycapitalize("Kodeclik online academy"))
The output is:
Kodeclik Online Academy
Like many other Python topics, Python provides multiple ways to tackle a given problem. In this blogpost, we have learnt 5 different ways to capitalize the first letter of a string with different properties and preferences on when these methods can be used. What is your favorite method?
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.