Kodeclik Blog
How to Copy a List in Python
The versatility of Python is easily seen in the very expressive ways it offers to copy a list in Python. This blogpost shows five different approaches.
Method 1: Copying a list by Assignment
The easiest way to copy lists is by assignment. Consider the following code:
mylist = ['Harry',"Potter"]
mylist_copy = mylist
print(mylist)
print(mylist_copy)
This program prints:
['Harry', 'Potter']
['Harry', 'Potter']
Showing that the original list has been copied correctly. There is one side effect of this method. If the original list is modified, the new list will also get modified. So, for instance:
mylist = ['Harry',"Potter"]
mylist_copy = mylist
print(mylist)
print(mylist_copy)
mylist.append('Book')
print(mylist)
print(mylist_copy)
returns:
['Harry', 'Potter']
['Harry', 'Potter']
['Harry', 'Potter', 'Book']
['Harry', 'Potter', 'Book']
This is because the assignment operator (“=”) merely points to the original list and so any modifications to that list will be reflected in the new list as well.
Note however that this does not happen with re-assignments which cause the copied pointer to continue to point to its original location. For instance:
mylist = ['Harry',"Potter"]
mylist_copy = mylist
print(mylist)
print(mylist_copy)
mylist = ['Book']
print(mylist)
print(mylist_copy)
yields:
['Harry', 'Potter']
['Harry', 'Potter']
['Book']
['Harry', 'Potter']
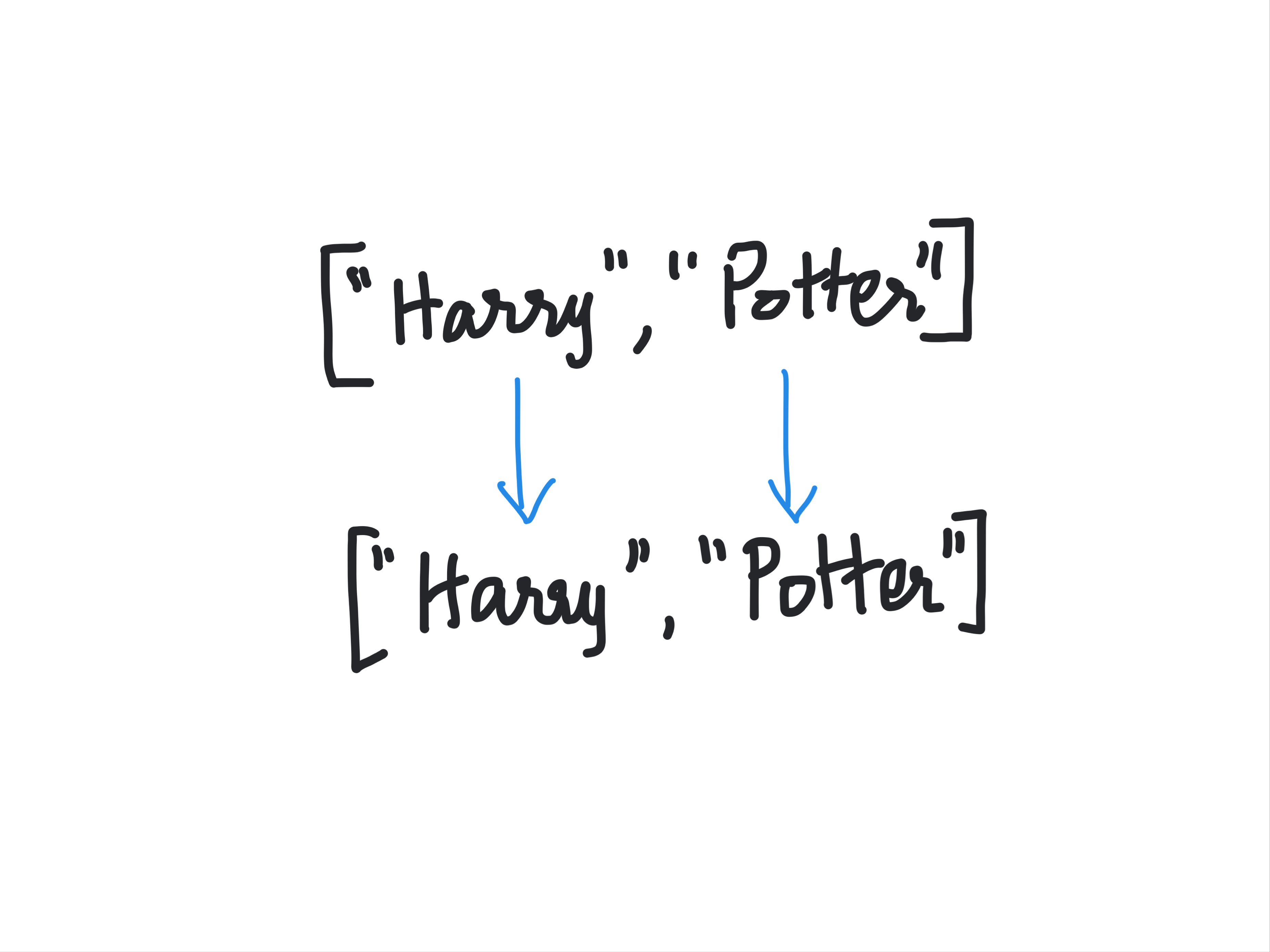
Method 2: Copying a list by the copy() method
The copy() method is another way to copy lists. It doesn’t take any arguments whatsoever and you apply it on a list to generate a copy of it which can be assigned to a new variable. Let us redo the above examples with this method.
mylist = ['Harry',"Potter"]
mylist_copy = mylist.copy()
print(mylist)
print(mylist_copy)
mylist.append('Book')
print(mylist)
print(mylist_copy)
will yield:
['Harry', 'Potter']
['Harry', 'Potter']
['Harry', 'Potter', 'Book']
['Harry', 'Potter']
Note that this behavior is different from the use of the assignment operator. The copy created is a completely new copy so that when the original list is modified, the new one is not modified.
Method 3: Copying a list by slicing operators
You can use slicing syntax to copy lists, like so:
mylist = ['Harry',"Potter"]
mylist_copy = mylist[:]
print(mylist)
print(mylist_copy)
This outputs:
['Harry', 'Potter']
['Harry', 'Potter']
If we try to modify the original list after copying:
mylist = ['Harry',"Potter"]
mylist_copy = mylist[:]
mylist.append('Book')
print(mylist)
print(mylist_copy)
we get:
['Harry', 'Potter', 'Book']
['Harry', 'Potter']
So the semantics of the slicing operator are similar to that of copy(), i.e., a completely fresh copy is created that is unmodified by changes to the original list.
Method 4: Copying a list by the list() method
We can use the list constructor list() to copy lists. Consider the following code:
mylist = ['Harry',"Potter"]
mylist_copy = list(mylist)
print(mylist)
print(mylist_copy)
mylist.append('Book')
print(mylist)
print(mylist_copy)
This outputs:
['Harry', 'Potter']
['Harry', 'Potter']
['Harry', 'Potter', 'Book']
['Harry', 'Potter']
So the semantics of the list() constructor are similar to the copy() method and to the slicing operator and unlike the assignment operator.
Method 5: Copying a list by list comprehension
We can use the method of list comprehension to loop through the current list and copy elements one by one. See:
mylist = ['Harry',"Potter"]
mylist_copy = [x for x in mylist]
print(mylist)
print(mylist_copy)
mylist.append('Book')
print(mylist)
print(mylist_copy)
which yields:
['Harry', 'Potter']
['Harry', 'Potter']
['Harry', 'Potter', 'Book']
['Harry', 'Potter']
Again, this yields the same semantics as the copy() method, slicing operator, and the list() constructor.
So there you have it - 5 different ways to copy lists! Which one is your favorite? In fact, it should not surprise you that there are many more ways to copy lists in Python!
The assignment operator approach to copying lists is considered a “deep” approach because modifications to one are reflected in the other. The other approaches are sometimes referred to as “shallow” approaches.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.