Kodeclik Blog
math.sin() in Python
Recall that the sine of an angle is defined as the opposite side (opposite to the angle) divided by the hypotenuse in a right-angled triangle. It is well known that the sine of zero is zero and the sine of 90 degrees (which is pi/2 radians) is 1. The Math.sin() is a handy function in Python to compute these trigonometric ratios. Consider the following program:
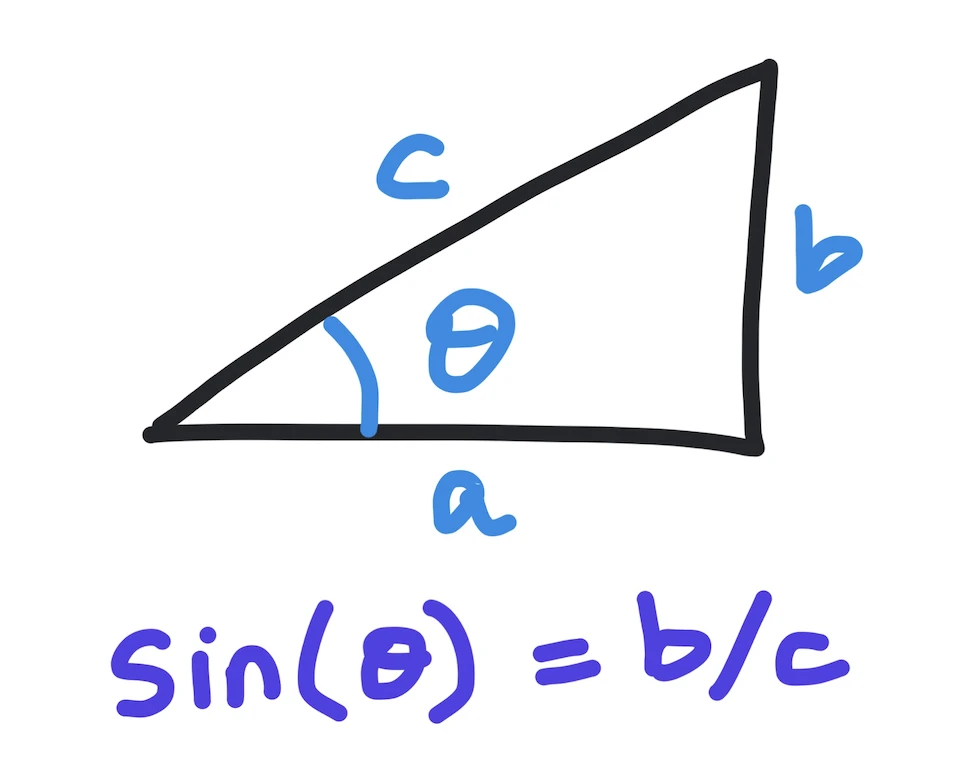
import math
print("Sine of " + str(0) + " degrees is "
+ str(math.sin(0)))
print("Sine of " + str(90) + " degrees is "
+ str(math.sin(90*math.pi/180)))
The output is:
Sine of 0 degrees is 0.0
Sine of 90 degrees is 1.0
Note that in the second line we are careful to convert degrees to radians (by scaling by pi/180) before passing the result to the sin() function. (In the first line, we know that 0 degrees is the same as 0 radians, so we do not need to be so careful.)
Computing the sine function using Taylor series approximation
If you did not have access to the sin() function you can approximate the sin function by using a Taylor series expansion (expanded around 0) as shown in the below function:
import math
def sine_approx(theta,n):
# first convert theta degrees to radians
t = theta*math.pi/180
sum = 0
# now use a
for i in range(0,n):
sum = sum + math.pow(-1,i)*math.pow(t,2*i+1)/math.factorial(2*i+1)
return(sum)
The above function takes two inputs, viz. Theta the angle for which we wish to compute the sine() and n, the number of terms. Inside the function, we first convert the angle to radians and then use a for loop to add up terms according to the Taylor expansion for sin(theta). Even though the Taylor’s series is infinite, we are approximating it here with a finite number of terms.
Now we can use this function like so:
print("Sine of " + str(0) + " degrees is "
+ str(math.sin(0)))
print("Sine of " + str(90) + " degrees is "
+ str(math.sin(90*math.pi/180)))
print("Sine of " + str(90) + " degrees is "
+ str(sine_approx(90,5)))
The output will be:
Sine of 0 degrees is 0.0
Sine of 90 degrees is 1.0
Sine of 90 degrees is 1.0000035425842861
As you can see we are using five terms in the sine function expansion to approximate the value of sin(). And you can see that the result is not exactly 1.0. (as produced by the Math.sin() function). If we increase the number of terms we will get increasingly more accurate results. Here is some code to explore this:
import math
def sine_approx(theta,n):
# first convert theta degrees to radians
t = theta*math.pi/180
sum = 0
# now use a
for i in range(0,n):
sum = sum + math.pow(-1,i)*math.pow(t,2*i+1)/math.factorial(2*i+1)
return(sum)
print("Taylor series computation of sin(x)")
for k in range(1,20):
print("Sine of " + str(90) + " degrees, with "
+ str(k) + " terms is " + str(sine_approx(90,k)))
The output will be:
Taylor series computation of sin(x)
Sine of 90 degrees, with 1 terms is 1.5707963267948966
Sine of 90 degrees, with 2 terms is 0.9248322292886504
Sine of 90 degrees, with 3 terms is 1.0045248555348174
Sine of 90 degrees, with 4 terms is 0.9998431013994987
Sine of 90 degrees, with 5 terms is 1.0000035425842861
Sine of 90 degrees, with 6 terms is 0.999999943741051
Sine of 90 degrees, with 7 terms is 1.0000000006627803
Sine of 90 degrees, with 8 terms is 0.9999999999939768
Sine of 90 degrees, with 9 terms is 1.0000000000000437
Sine of 90 degrees, with 10 terms is 1.0
Sine of 90 degrees, with 11 terms is 1.0000000000000002
Sine of 90 degrees, with 12 terms is 1.0000000000000002
Sine of 90 degrees, with 13 terms is 1.0000000000000002
Sine of 90 degrees, with 14 terms is 1.0000000000000002
Sine of 90 degrees, with 15 terms is 1.0000000000000002
Sine of 90 degrees, with 16 terms is 1.0000000000000002
Sine of 90 degrees, with 17 terms is 1.0000000000000002
Sine of 90 degrees, with 18 terms is 1.0000000000000002
Sine of 90 degrees, with 19 terms is 1.0000000000000002
As you can see, after a few terms we see the series converging to the desired value.
In conclusion, you can use the math.sin() function which is a built-in function in the math module of Python or you can construct your own approximation using Taylor series.
If you liked learning about the math.sin() function, you should checkout our blogposts on the math.cos() and math.tan() functions!
Now that you have mastered the math.sin() function, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.