Kodeclik Blog
How to remove trailing zeros in Python
In your Python journey, you will encounter situations where you have a string denoting a floating point number but which might contain extraneous trailing zeros. For instance, you might have a string such as “314.560” or “123.4000” and you desire to remove the trailing zeros, so that we obtain “314.56” or “123.4”. Here are five ways to remove trailing zeros from a Python string.
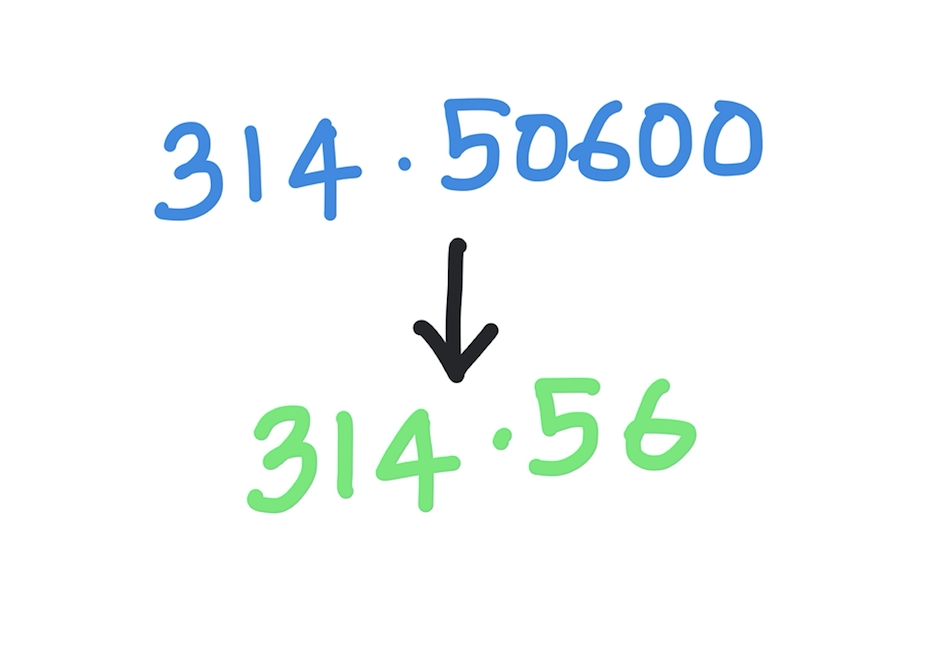
Method 1: Use a for loop
We can write a vanilla for loop going through the string in reverse and remove trailing zeros as we encounter them. For this purpose we create a variable to keep track of whether we have encountered the first non-zero character and once we do so we no longer remove zeros. For instance, a string such as “314.50600” should be converted to “314.506”, not “314.56”.
In the code below, mystring contains the original string with potentially trailing zeros and answer contains the modified string, with zeros removed from the end. The variable “inside” keeps track of whether we have crossed the trailing zeros portion of the string. Note also that we use the pass statement because we have an empty block in our if..else statement.
To keep the notation simple we reverse the string for use in an iterator and then re-reverse it (using string slicing operators) after the zeros are removed.
mystring = "314.50600"
inside = False
newstring = ''
for x in reversed(mystring):
if (not(inside) and x=="0"):
pass
else:
newstring = newstring + x
inside = True
answer = newstring[::-1]
print(answer)
The output is, as expected:
314.506
Method 2: Use string slicing operators in a while loop
The above code can be made more succinct using Python’s string slicing operators in a while loop. For instance, consider the following code:
mystring = "314.50600"
while (mystring[-1] == '0'):
mystring = mystring[:-1]
print(mystring)
This will output as before:
314.506
Method 3: Use the rstrip() method
The rstrip() method operates on a given string and returns a string with trailing characters removed (and you can specify which trailing characters you are interested in).
mystring = "314.50600"
print(mystring)
print(mystring.rstrip('0'))
In the above code rstrip works on the mystring variable and removes trailing characters where the trailing character is specified to be 0.
This code outputs:
314.50600
314.506
See how simple the code is? This is because somebody has done the hard work of writing the logic we need inside the rstrip() method.
Method 4: Recursive removal of trailing zeros
In this method we write our own function that recursively calls itself to remove trailing zeros. If there are no zeros it simply returns its input as the output.
def remove_trailing_zeros(s):
if s.endswith('0'):
return(remove_trailing_zeros(s[:-1]))
else:
return(s)
In the above we use the endswith() method to check if the string has a trailing zero. Note that this will only check if there is a single trailing zero and will not be able to distinguish between one or two (or more) trailing zeros. Thus, as soon as a trailing zero is detected, we recursively call the function with the string with that last character removed. If no trailing zero is detected, the given string is simply returned (the base case for the recursion).
If we use this function like so:
print("314.50600")
print(remove_trailing_zeros("314.50600"))
we will get:
314.50600
314.506
Method 5: Use float() and str() casting functions
The built-in float() function converts a string to a float and str() converts a float (or an integer) to a string. Implicitly float() helps remove trailing zeros. Thus we can create a simple routine to remove trailing zeros with:
print("314.50600")
print(str(float("314.50600")))
This will output:
314.50600
314.506
Note that this approach will also work if you have leading zeros. Thus the code:
print("00314.50600")
print(str(float("00314.50600")))
yields:
00314.50600
314.506
What will happen with the earlier methods? Will they also work with leading zeros? Explore it and see if you need to make any modifications.
What happens if we give a negative integer, such as “-00314.50600”? Which methods work? We will leave it as an exercise for you to try them out and modify them as/if necessary.
If you enjoyed this blogpost, checkout our post on removing leading zeros in a Python string. For more string manipulation, see our post on removing parentheses from a Python string
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.