Kodeclik Blog
How to remove leading zeros in Python
In your Python journey, you will encounter situations where you have a string denoting a number but which might contain extraneous leading zeros. For instance, you might have a string such as “00314” or “01234” and you desire to remove the leading zeros, so that we obtain “314” or “1234”. Here are five ways to remove leading zeros from a Python string.
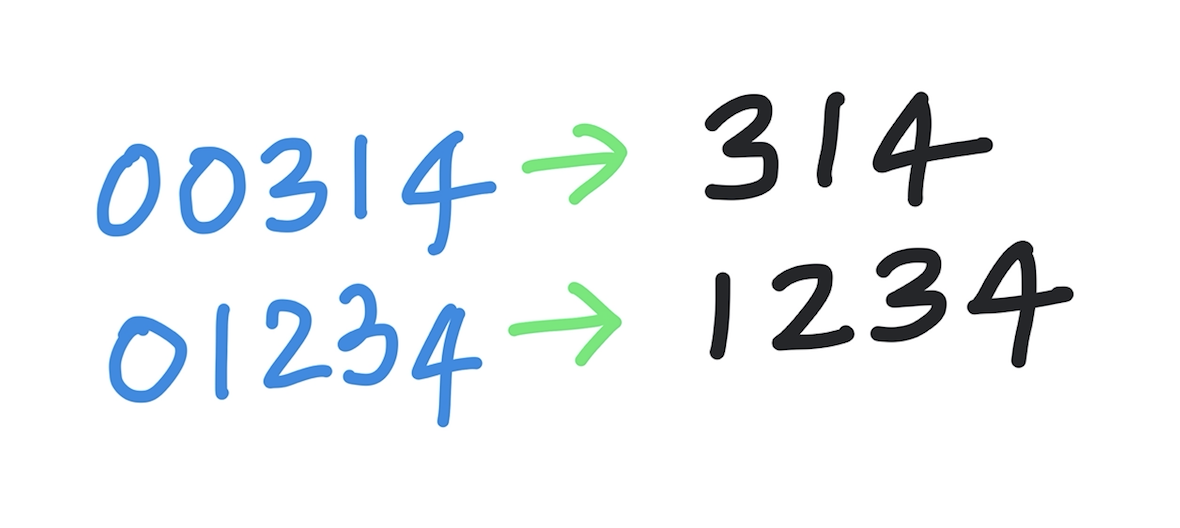
Method 1: Use a for loop
We can write a vanilla for loop going through the string one character at a time and remove the leading zeros. For this purpose we create a variable to keep track of whether we are at the beginning of the string and once we cross the segment of leading zeros we recognize that we no longer need to remove leading zeros.
In the code below, mystring contains the original string with potentially leading zeros and newstring contains the modified string, with zeros removed. The variable beginning keeps track of whether we are at the beginning of the string. Note also that we use the pass statement because we have an empty block in our if..else statement.
mystring = "000203"
beginning = True
newstring = ''
for x in mystring:
if (beginning and x=="0"):
pass
else:
newstring = newstring + x
beginning = False
print(newstring)
The output is, as expected:
203
Method 2: Use string slicing operators in a while loop
The above code can be made more succinct using Python’s string slicing operators in a while loop. For instance, consider the following code:
mystring = "000203"
while (mystring[0] == '0'):
mystring = mystring[1:]
print(mystring)
This will output as before:
203
Method 3: Use the lstrip() method
The lstrip() method operates on a given string and returns a string with leading characters removed (and you can specify which leading characters you are interested in).
mystring = "000203"
print(mystring)
print(mystring.lstrip('0'))
In the above code lstrip works on the mystring variable and removes leading characters where the leading character is specified to be 0.
This code outputs:
000203
203
See how simple the code is? This is because somebody has done the hard work of writing the logic we need inside the lstrip() method.
Method 4: Recursive removal of leading zeros
In this method we write our own function that recursively calls itself to remove leading zeros. If there are no zeros it simply returns its input as the output.
def remove_leading_zeros(s):
if s.startswith('0'):
return(remove_leading_zeros(s[1:]))
else:
return(s)
In the above we use the startswith() method to check if the string has a leading zero. Note that this will only check if there is a first leading zero and will not be able to distinguish between one or two (or more) leading zeros. Thus, as soon as a leading zero is detected, we recursively call the function with the string from the second character. If no leading zero is detected, the given string is simply returned (the base case for the recursion).
If we use this function like so:
print("00203")
print(remove_leading_zeros("00203"))
we will get:
00203
203
Method 5: Use int() and str() casting functions
The built-in int() function converts (casts) a string to an integer and str() converts (casts) an integer to a string. Implicitly int() helps remove leading zeros. Thus we can create a simple routine to remove leading zeros with:
print("00203")
print(str(int("00203")))
This will output:
00203
203
Note that this approach will only work for integers. It will not work if you have a decimal point in your number (but the earlier methods will work).
If we try:
print("0020.3")
print(str(int("0020.3")))
we will get an error like:
0020.3
Traceback (most recent call last):
File "main.py", line 2, in <module>
print(str(int("0020.3")))
ValueError: invalid literal for int() with base 10: '0020.3'
However, the earlier methods will work for this case. To make this approach work with decimals, you simply have to update the int() function to use a float() instead. So the code becomes:
print("0020.3")
print(str(float("0020.3")))
which yields:
0020.3
20.3
What happens if we give a negative integer, such as “-0023”? Which methods work? We will leave it as an exercise for you to try them out and modify them as/if necessary.
If you enjoyed this blogpost, checkout our post on removing trailing zeros in a Python string. Learn also how to pad a Python string and how to remove the first character from a Python string.
Finally, learn about other casting functions such as the float() function in Python to convert strings to floating point values.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.