Kodeclik Blog
How to scramble a word in Javascript
Let us try to write a Javascript function to scramble a word, i.e., to permute the characters in the word to make up a new word.
To explore this problem, we will write and embed our Javascript code inside a HTML page like so:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Scrambling a word in Javascript</title>
</head>
<body>
<script>
</script>
</body>
</html>
In the above HTML page you see the basic elements of the page like the head element containing the title (“Scrambling a word in Javascript”) and a body with an empty script tag. The Javascript code goes inside these tags.
Note that strings in Javascript are immutable so if we desire to scramble a word represented as a string, we must first convert it into an array, do the scrambling operations on the array, and then convert the array back into a string.
One way to do it is to loop through the characters of a string (now in an array) one by one. For each character, swap it with a random character that appears earlier in the array. This way we can guarantee that we preserve the number of specific characters in the array.
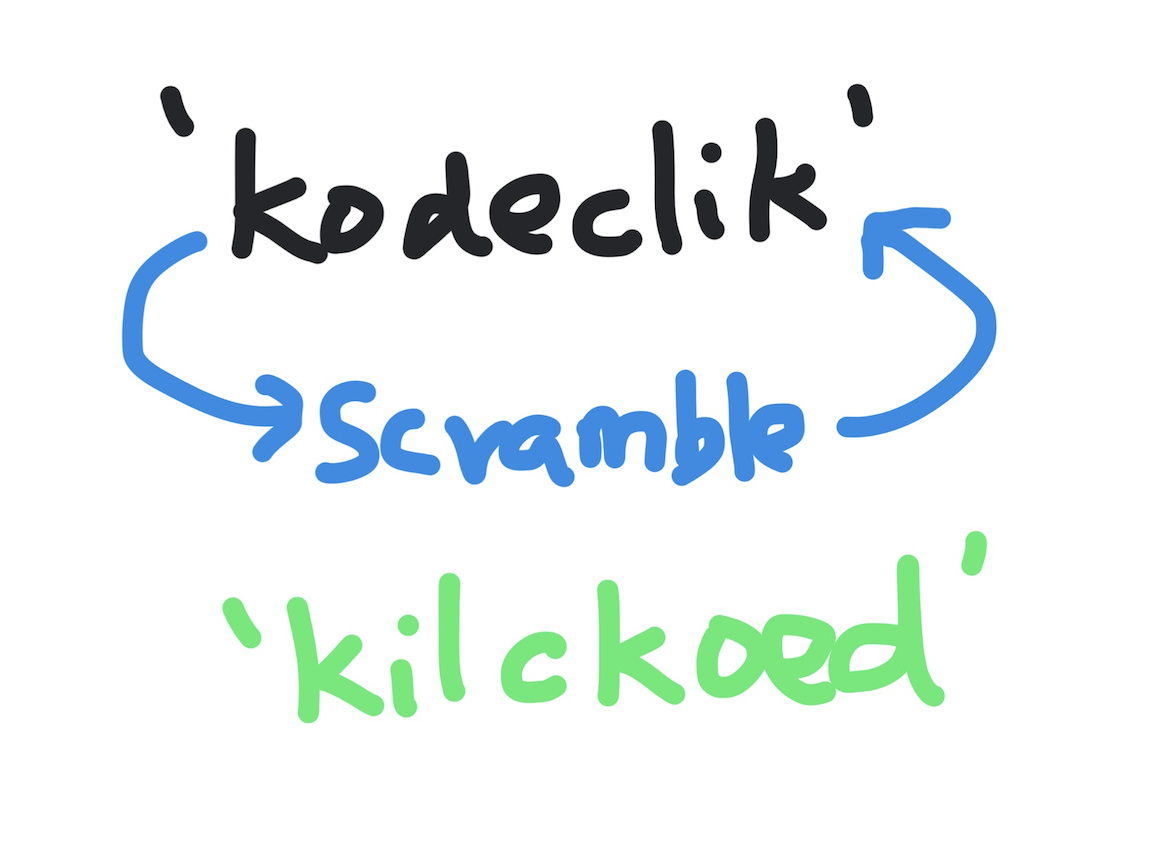
Here is a basic Javascript program:
<script>
function scramble(word) {
strarray = word.split('');
var i,j,k
for (i = 0; i < strarray.length; i++) {
j = Math.floor(Math.random() * i)
k = strarray[i]
strarray[i] = strarray[j]
strarray[j] = k
}
word = strarray.join('');
return word;
}
document.write(scramble("kodeclik"))
</script>
In the above program we have a function called scramble that takes a string (word) as input. The first line of scramble converts the string into an array (strarray). This conversion is done using the split() method. Similarly the last two lines of the function convert the array back to a string and return it. Likewise, this conversion is done using the join() method.
Within the function we loop through the array. For each element we find a random location preceding that element’s location. Then we swap the characters using these three lines:
k = strarray[i]
strarray[i] = strarray[j]
strarray[j] = k
If we run this program, we will obtain:
dcokekli
(your specific output might vary due to the random choice of characters to swap.)
To understand more about how this program, let us write some document.write() statements, like so:
<script>
function scramble(word) {
document.write('Original Word: ' + word)
document.write('<BR>')
strarray = word.split('');
var i,j,k
for (i = 0; i < strarray.length; i++) {
j = Math.floor(Math.random() * i)
k = strarray[i]
strarray[i] = strarray[j]
strarray[j] = k
document.write('Exchanging ' + strarray[j]
+ ' -> ' + strarray[i] + '<BR>')
document.write('New Word: ' + strarray.join('') + '<BR>')
}
word = strarray.join('');
return word;
}
document.write(scramble("kodeclik"))
</script>
The output is (for instance):
Original Word: kodeclik
Exchanging k -> k
New Word: kodeclik
Exchanging o -> k
New Word: okdeclik
Exchanging d -> o
New Word: dkoeclik
Exchanging e -> k
New Word: deokclik
Exchanging c -> k
New Word: deocklik
Exchanging l -> o
New Word: delckoik
Exchanging i -> e
New Word: dilckoek
Exchanging k -> d
New Word: kilckoed
kilckoed
As the output above shows, for the first character (namely, ‘k’) there is no other character to swap it with, so it is swapped with itself giving the same string, i.e., ‘kodeclik’. The second character (‘o’) is swapped with the first (‘k’), yielding ‘okdeclik’. This process continues till the last character, which is ‘k’. This is swapped with ‘d’ yielding ‘kilckoed’ as the final string which is returned and printed by the program.
We hope you enjoyed learning how to scramble a word in Javascript!
Interested in learning more Javascript? Learn how to loop through two Javascript arrays in coordination, how to remove elements from a DOM using Javascript, and how to make a Javascript function return multiple values!
Want to learn Javascript with us? Sign up for 1:1 or small group classes.