Kodeclik Blog
List subtraction in Python
There will come a time when you will need to perform element by element list subtraction in Python. For instance, you might have a list of prices (of some items) and a separate list of discounts and you will need to find the discounted price by subtracting the discounts from the original price, in an element by element manner.
For instance, consider the two Python lines of code:
prices = [10,20,30]
discounts = [1,8,5]
Note that both lists are of the same size (i.e., of length 3) and we assume so in this example. We desire to subtract them element by element to find a list of discounted prices.
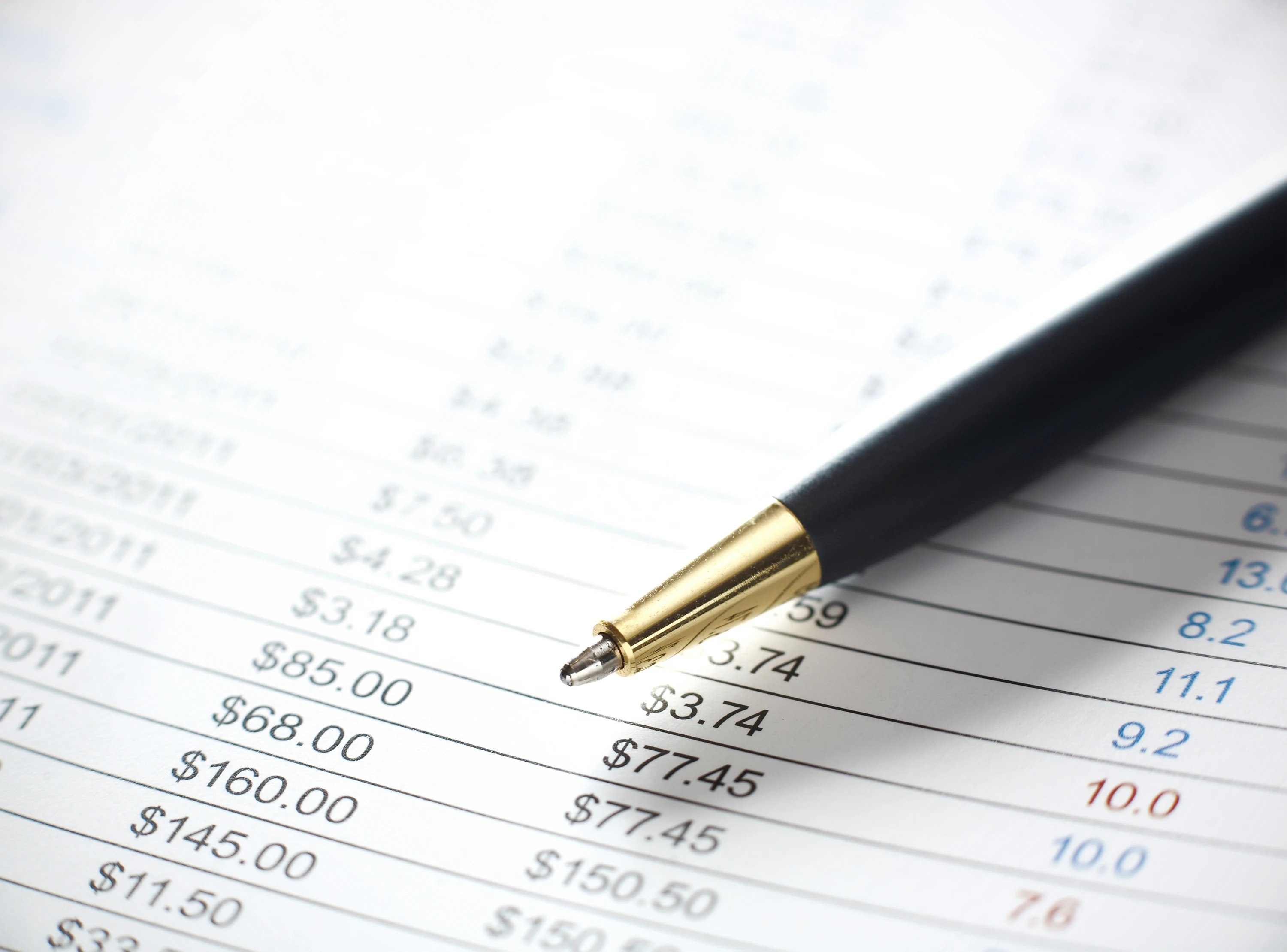
We will learn six different ways to accomplish this task in Python.
Method 1: List subtraction using a for loop
The easiest way to do list subtraction is to use a simple for loop. Here is how that might work:
prices = [10,20,30]
discounts = [1,8,5]
answer = []
for x in range(len(prices)):
answer.append(prices[x]-discounts[x])
print("Prices after discounts:")
print(answer)
The bulk of the computation happens in the for loop. Before the for loop, we create an empty “answer” list. At each step of the for loop, we append to this list the element-wise difference between the prices and discounts list. Note the range of the for loop. Because the lists are assumed to be the same length we use the range() function to set the limit of the for loop.
When we run this program, we get:
Prices after discounts:
[9, 12, 25]
as expected.
Method 2: List subtraction using a while loop
A second approach is to replace the for loop with a while loop.
prices = [10,20,30]
discounts = [1,8,5]
answer = []; x = 0
while x < len(prices):
answer.append(prices[x]-discounts[x])
x = x+1
print("Prices after discounts:")
print(answer)
Note that in this program we initialize the variable “x” to zero, then increment it step by step inside the while loop till we reach the end of the list (i.e., the length of the “prices” list). Inside the while loop, the actual operation is still the same.
The output of the program is the same as before:
Prices after discounts:
[9, 12, 25]
Method 3: List subtraction using list comprehensions
We can accomplish our list subtraction goals in a succinct manner using list comprehensions:
prices = [10,20,30]
discounts = [1,8,5]
answer = [(prices[x]-discounts[x])
for x in range(len(prices))]
print("Prices after discounts:")
print(answer)
Note that we have a single line of code that computes the “answer” list. In that line, we are iterating over the length of the “prices” list (similar to Method 1 above) and using a list comprehension to add the necessary elements instead of using an append function.
The output is as before:
Prices after discounts:
[9, 12, 25]
Method 4: List subtraction using the zip() function
The zip function gives element wise pairs from two (or more) lists and then we can use a similar approach to the previous method to do the subtraction:
prices = [10,20,30]
discounts = [1,8,5]
answer = [(x-y) for (x,y) in
zip(prices, discounts)]
print("Prices after discounts:")
print(answer)
Here, see that the zip function yields pairs (x,y) and then we construct the elements of the “answer” list by doing subtractions of these pairs.
The output is the same as before:
Prices after discounts:
[9, 12, 25]
Method 5: Using the numpy library
Our fifth method (wow!) uses the numpy library in Python that has an inbuilt element-wise subtraction function for arrays. But first we need to convert our lists to numpy arrays. Here is how that works:
import numpy as np
prices = [10, 20, 30]
discounts = [1, 8, 5]
prices_array = np.array(prices)
discounts_array = np.array(discounts)
answer_array = np.subtract(prices_array, discounts_array)
answer = list(answer_array)
print("Prices after discounts:")
print(answer)
Note that we first import the numpy library (as “np”). Then we create a “prices_array” numpy array based on the prices list and, similarly, a “discounts_array” based on the discounts list. The main action takes place in the computation of the “answer_array” (which is another numpy array) using the np.subtract function. Finally, this numpy array is converted back to a regular Python list using the list() constructor.
The output is:
Prices after discounts:
[9, 12, 25]
Method 6: Using map and lambda functions
Our final method takes a “back to basics” approach and uses a lambda function (i.e. in pure functional programming style) and a map function that applies a function uniformly throughout a data structure, such as a list. Here is how that works:
prices = [10, 20, 30]
discounts = [1, 8, 5]
answer = list(map(lambda x, y: x-y, prices, discounts))
print("Prices after discounts:")
print(answer)
Note that the lambda function simply takes two arguments (x and y) and computes their difference. In other words, it takes single numerical values and subtracts them. This lambda function is then applied over the full arrays (namely, prices and discounts) using the map function. The output of these map values is used in the list constructor to construct the “answer” list.
The output is:
Prices after discounts:
[9, 12, 25]
If you did not use the list constructor, you would have obtained an answer like:
Prices after discounts:
<map object at 0x7f85b4fac430>
because directly printing the map object is not very informative.
We have learnt six different ways to do element-wise list subtraction in Python. Which one is your favorite? Also checkout our blogpost on unzipping lists in Python!
The above codes have not been rigorously tested for all cases. For instance, try them when one of the lists is empty, or when the lists are of different sizes, or when the lists have elements for which subtraction is defined. We will leave it as an exercise for you to handle these cases.
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.