Kodeclik Blog
Loop count in Python
Imagine if you have a for loop iterating through the characters of a string, like so:
message = "Kodeclik Online Academy"
for x in message:
print(x,end=' ')
print()
The output will be:
K o d e c l i k O n l i n e A c a d e m y
What if you would like to update the above code so that after every fourth character you would like to print an emoji, like a green heart symbol, as below.
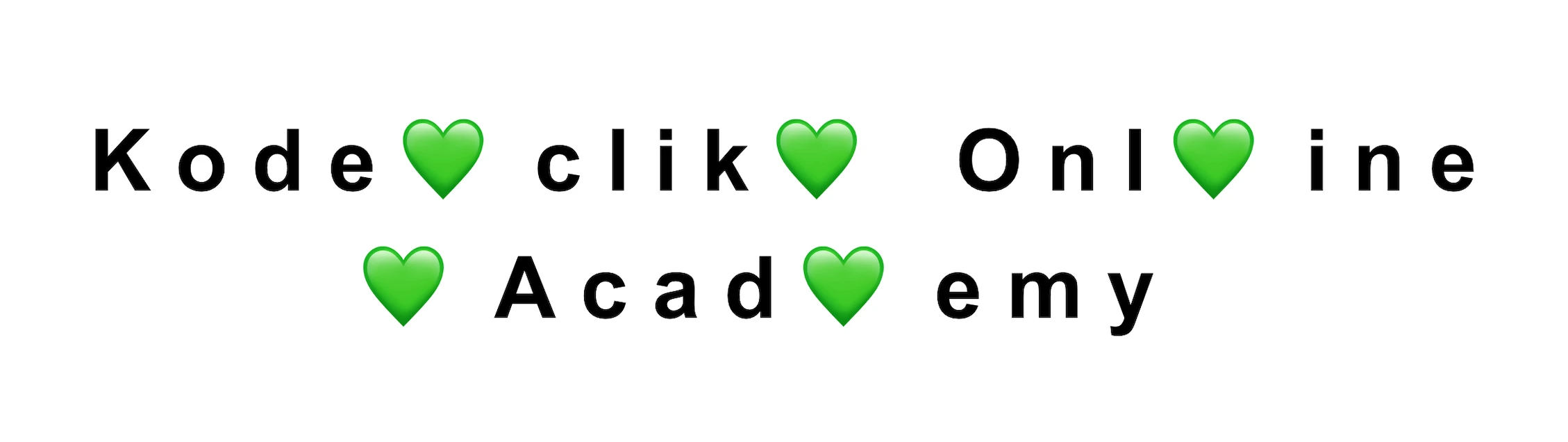
The code above does not directly help you with that because x is just a variable holding the actual character, not the index of the for loop.
You can of course do it by first finding the length of the string, setting up a for loop for that length and inside the for loop accessing the character and printing it (or printing a heart symbol as appropriate). But this is not the Python-ic way to do it. The correct approach is to use Python’s enumerate function, which returns both the loop index and the character:
message = "Kodeclik Online Academy"
for (position, character) in enumerate(message):
if ((position+1) % 4 == 0):
print(character,"\\U0001F49A ",end=' ')
else:
print(character,end=' ')
print()
Here, the enumerate() function operates on the string (“Kodeclik Online Academy”) and returns the elements of the string one by one for use in the loop. For each element of the string, it returns the position (starting from 0) and the character (starting from “K”). Inside the loop we check for every fourth element and if so we print the character followed by a green heart symbol. Else we just print the character. The output will be:
K o d e 💚 c l i k 💚 O n l 💚 i n e 💚 A c a d 💚 e m y
Note how succinct the code is: it does not require you to do the loop counting yourself or even to use the count to access the character yourself. Python returns both of them directly to you in one step for use in your program.
Highlighting a month in a calendar
Here is a second example of where you would need to do a loop count and where enumerate() comes in handy. Let us assume we would like to print a list of months in a year but highlight only one month (which is given as input). Here is an initial start:
months = ['January','February','March','April',
'May','June','July','August','September',
'October','November','December']
for (x,y) in enumerate(months):
message = str(x+1) + ": " + y
print(message)
This program uses enumerate() to obtain both the number of the month (recall that they begin with 0) in variable x and the month name in variable y. Then we construct a suitable message and print it. The output will be:
1: January
2: February
3: March
4: April
5: May
6: June
7: July
8: August
9: September
10: October
11: November
12: December
Let us now write a function called “highlight” that takes a month number as input and highlights just that row in the calendar with an underline:
months = ['January','February','March','April',
'May','June','July','August','September',
'October','November','December']
def highlight(month_num):
for (x,y) in enumerate(months):
message = str(x+1) + ": " + y
if (x+1 == month_num):
print("\\033[92m{}\\033[00m".format(message))
else:
print(message)
highlight(3)
Again in this program, we have used enumerate() to fetch both the month number (in variable month_num) and month name. We check if the month number is equal to the index and if so we print it in a different color (again taking into account the fact that the indices returned from enumerate begin at 0, not 1).
The output will be:
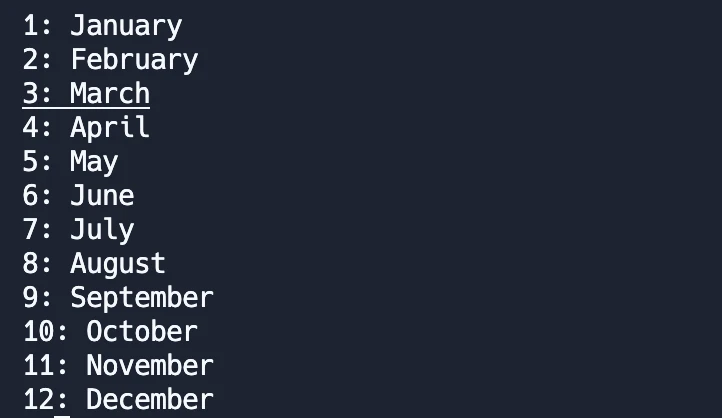
Thus the main advantage of using enumerate() is that it saves us from having to create our own loop counter variable. By returning tuples containing both indices and the contents at these indices in an iterable, we make programs simpler to understand and easier to maintain.
If you liked this blogpost, learn more applications of Python enumerate().
For more Python content, checkout the math.ceil() and math.floor() functions! Also
learn about the math domain error in Python and how to fix it!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.